Resolving TinyMCE 6 Plugin Conflicts in Java Applications
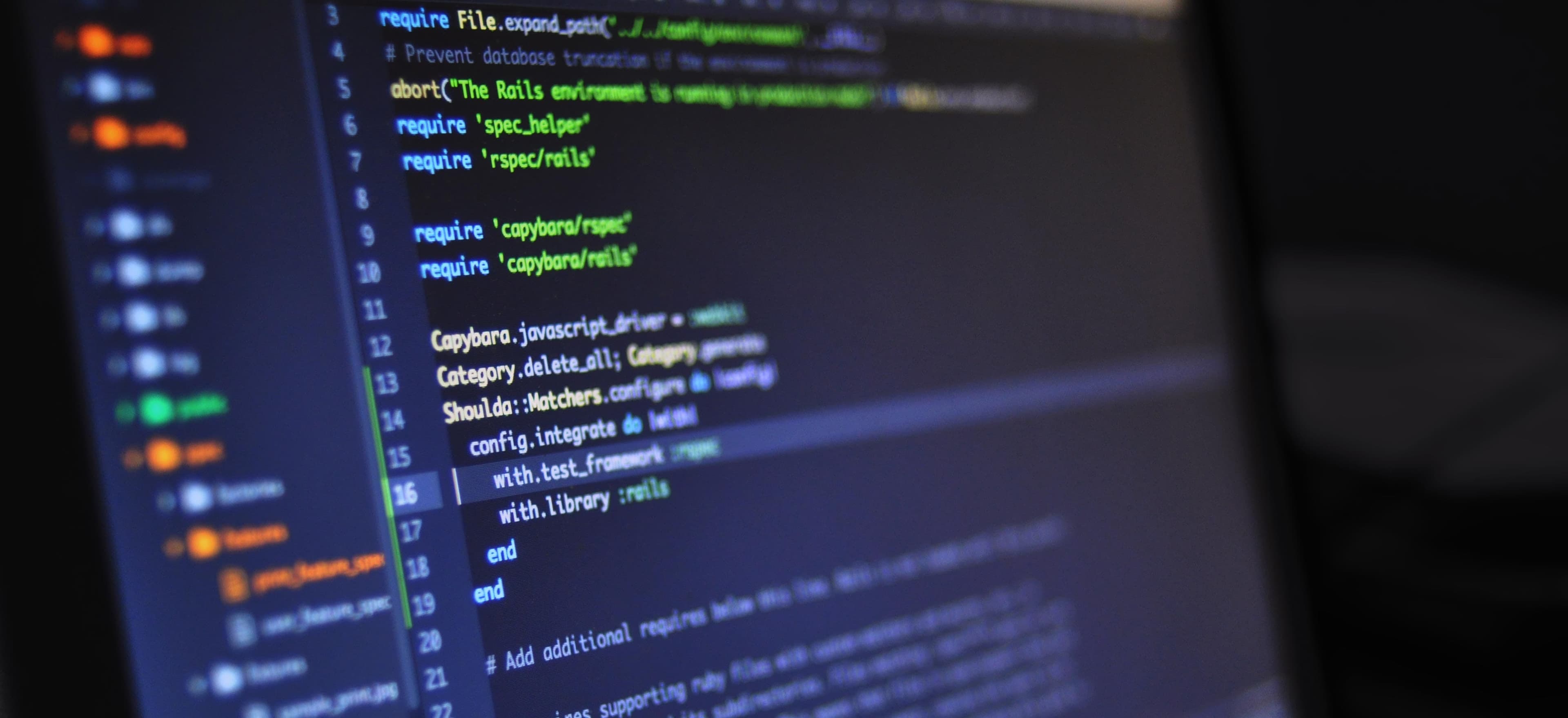
- Published on
Resolving TinyMCE 6 Plugin Conflicts in Java Applications
Java developers often face unique challenges when integrating rich text editors like TinyMCE 6 into their applications. These conflicts can occur due to overlapping functionalities, compatibility issues, or incorrect configurations. In this blog post, we will explore strategies to resolve TinyMCE 6 plugin conflicts within your Java applications. We will also take a closer look at common issues and offer clear solutions, illustrated with concise code snippets that explain both functionality and purpose.
What is TinyMCE?
TinyMCE is a popular web-based rich text editor that enables developers to incorporate text-editing capabilities into web applications. With its extensive plugin system, TinyMCE can be tailored to meet diverse user needs. However, with great flexibility comes the potential for conflicts among plugins. Understanding how to resolve these conflicts is crucial for a seamless user experience.
Common Plugin Conflicts
Before diving into solutions, let's specify a few common types of conflicts you might encounter when using TinyMCE 6 plugins in Java applications:
- Overlapping Functionalities: Different plugins may provide similar functions or features, leading to unexpected behavior.
- Compatibility Issues: Certain plugins may not be compatible with TinyMCE 6 or other selected plugins.
- Configuration Errors: Incorrect settings can cause conflicts even among compatible plugins.
Troubleshooting TinyMCE 6 Plugin Issues
When you encounter issues with TinyMCE plugins, the first step is to identify the conflict. You can reference excellent resources, like the article on Troubleshooting Common Issues in TinyMCE 6 Plugins with React, which provides in-depth solutions that can also apply to Java applications.
Now, let's examine some effective strategies to resolve plugin conflicts.
1. Simplifying Plugin Selection
Sometimes, the simplest approach is to minimize the number of plugins you use. Evaluate the functionalities you've implemented and consider if you can achieve your goals with fewer plugins.
Example:
tinymce.init({
selector: '#editor',
plugins: 'lists link image',
toolbar: 'undo redo | styleselect | bold italic | alignleft aligncenter alignright alignjustify | outdent indent | link image',
});
Why It's Important: By limiting the number of plugins to the essentials, you reduce the likelihood of conflicts while enhancing performance. Focus on what you truly need.
2. Explicitly Defining Plugin Order
The order in which plugins are initialized can affect their behavior. TinyMCE processes plugins sequentially, so loading order may resolve conflicts.
Example:
tinymce.init({
selector: '#editor',
plugins: 'link image code',
toolbar: 'undo redo | link image | code',
});
Why It Matters: Explicit plugin ordering ensures that dependencies are resolved in the required sequence, preventing clashes and enhancing stability.
3. Debugging with the Browser Console
The browser console is an invaluable tool for diagnosing issues. Use it to check for error messages or warnings that might indicate conflicts between plugins.
Steps:
- Open the Developer Tools in your browser (usually F12).
- Check the Console tab for any error messages related to TinyMCE.
- Review the Stack Trace to pinpoint where the conflict might be occurring.
Why Debugging? Using the console to debug issues allows you to quickly identify and resolve potential conflicts, saving development time.
4. Customize Plugin Configuration
If you find specific plugins causing issues, consider customizing their settings. TinyMCE allows a variety of configurations that can help to avoid conflicts.
Example:
tinymce.init({
selector: '#editor',
plugins: 'table autolink',
table_default_attributes: {
class: 'my-custom-class'
},
autolink: {
// Custom autolink settings to avoid conflicts
handleEmail: true,
handleUrl: true
}
});
Reasoning: Customizing individual plugin settings can mitigate conflicts by changing how they interact with each other. Tailor them to meet your specific needs.
5. Updating TinyMCE and Plugins
Using outdated versions of TinyMCE or its plugins can result in compatibility issues. Always ensure that you are running the latest versions.
Check for Updates:
- Regularly visit the TinyMCE official website to check for updates.
- Update your dependencies accordingly.
Why Keep Updated? Updates often resolve known bugs and conflicts, improving overall performance and security within your applications.
6. Implementing Fallback Functions
In case of specific plugins that cause recurrent issues, create fallback functions. This assures your application remains functional even if a plugin fails.
Example:
function initTinyMCE() {
if (typeof tinymce !== 'undefined') {
tinymce.init({
selector: '#editor',
plugins: 'lists link',
// additional settings...
});
} else {
console.error('TinyMCE could not be initialized');
// Implement fallback logic here...
}
}
Why This Approach? Creating fallback functions ensures your application maintains core functionalities—allowing for a graceful degradation during unexpected plugin failures.
To Wrap Things Up
Incorporating TinyMCE 6 into your Java applications can raise complexities, especially concerning plugin conflicts. By simplifying plugin selections, explicitly defining orders, utilizing debugging techniques, customizing configurations, keeping components updated, and implementing fallback strategies, you can effectively navigate these challenges.
Consider visiting Troubleshooting Common Issues in TinyMCE 6 Plugins with React for more insights and advice. With diligence and the right strategies in place, smooth integration of TinyMCE into your Java project is entirely achievable.
Whether you're building a blog, a content management system, or any application requiring rich text editing, these strategies will serve you well. Happy coding!
Checkout our other articles