Streamlining TinyMCE 6 Plugin Integration in Java Applications
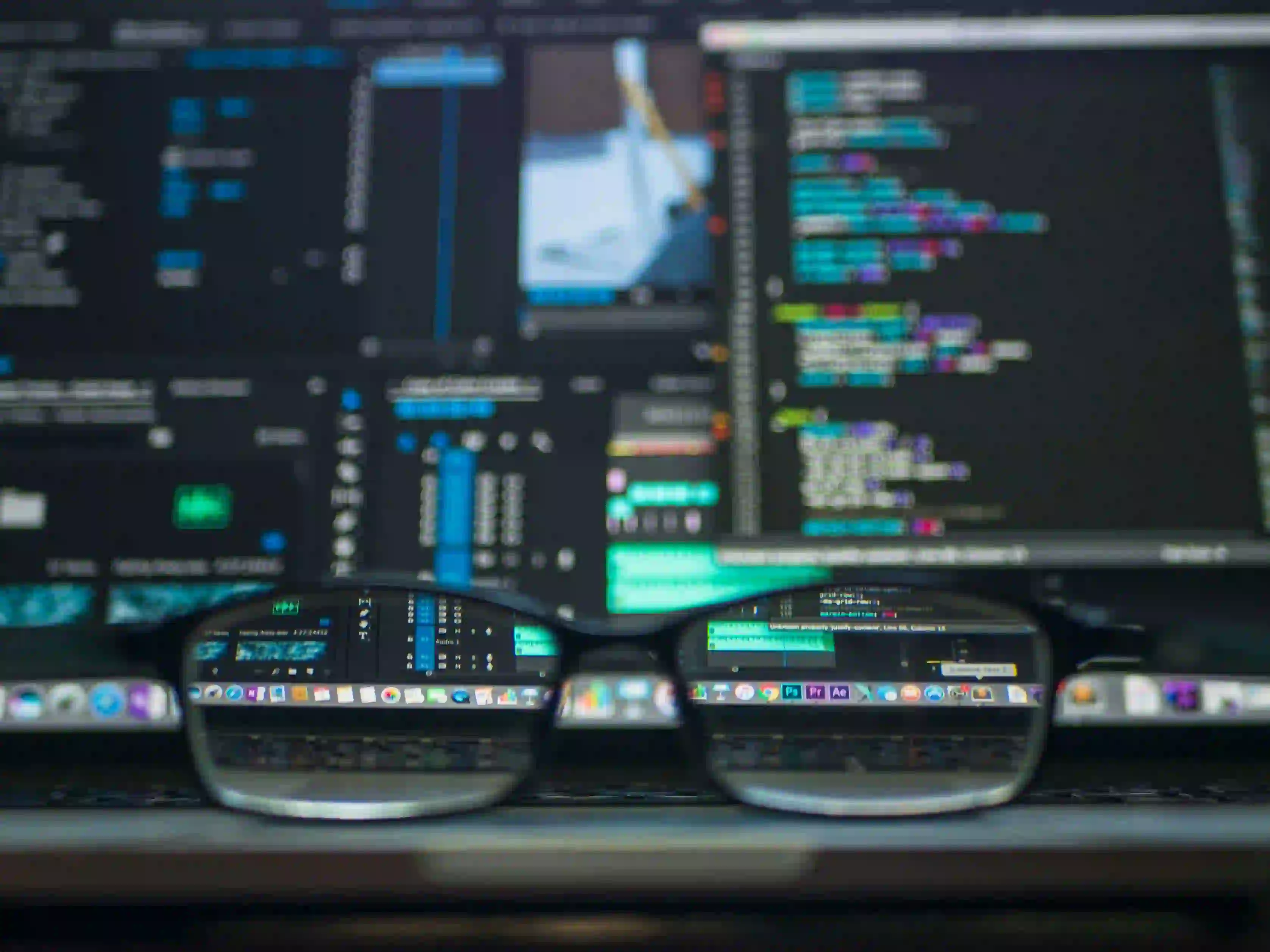
Streamlining TinyMCE 6 Plugin Integration in Java Applications
TinyMCE is a versatile WYSIWYG editor widely used to enhance user-generated content in web applications. Its flexibility lies not only in its feature-rich interface but also in integrations with various frameworks and languages. This blog post will focus on Streamlining TinyMCE 6 Plugin Integration in Java Applications, aimed at developers who want to leverage TinyMCE within their Java ecosystem effectively.
Understanding TinyMCE and Its Plugins
TinyMCE is an open-source rich text editor that provides dynamic editing features right in the browser. Unlike static content editors, it allows users to create and edit content in a more engaging way. Plugins enhance its capability, allowing developers to introduce additional features, functionalities, or integrations seamlessly.
Why Use TinyMCE?
- User-Friendly Interface: Its easy-to-navigate UI simplifies the content creation process.
- Highly Customizable: TinyMCE can be extended using plugins, custom skins, and toolbar configurations.
- Wide Compatibility: Works well across different browsers and platforms.
Plugin Architecture
Plugins are an essential part of TinyMCE. They enable functionalities such as image upload, formatting tools, and more. Understanding how to integrate these plugins into your Java application can enhance the editing experience, making it more intuitive and functional.
Setting Up TinyMCE in a Java Application
To integrate TinyMCE into a Java application, follow these steps:
1. Include TinyMCE in Your Project
You can include TinyMCE in your project using <script>
tags directly from a CDN or download the package to serve it locally.
Using a CDN:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Java Application</title>
<script src="https://cdn.tiny.cloud/1/no-api-key/tinymce/6/tinymce.min.js" referrerpolicy="origin"></script>
</head>
<body>
<h1>Integrating TinyMCE</h1>
<textarea id="mytextarea">Hello, World!</textarea>
<script>
tinymce.init({
selector: '#mytextarea'
});
</script>
</body>
</html>
Commentary
In the code above, we use a content delivery network (CDN) to load TinyMCE. This method is quick for testing but may not be suitable for production. When in production environments, ensure local hosting or integration with your build tool.
2. Configure TinyMCE with Java Backend
Now that TinyMCE is set up in your HTML file, we need to implement the Java backend to handle content submission after editing.
Example Servlet
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/submitContent")
public class ContentSubmitServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String content = request.getParameter("content");
// Here, you would typically save content to your database
System.out.println("Submitted Content: " + content);
response.getWriter().write("Content Submitted Successfully");
}
}
Commentary
In this servlet, we retrieve the submitted content from the frontend. The doPost
method handles the form submission. You should always sanitize and validate incoming data to avoid security vulnerabilities like XSS attacks.
3. Updating JavaScript to Handle Form Submission
Once configured, you need to modify the JavaScript setup for handling content submissions.
<script>
tinymce.init({
selector: '#mytextarea',
setup: function(editor) {
editor.on('change', function() {
editor.save();
});
}
});
function submitContent() {
const content = tinymce.get('mytextarea').getContent();
fetch('/submitContent', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: 'content=' + encodeURIComponent(content)
}).then(response => {
return response.text();
}).then(data => {
alert(data);
});
}
</script>
Commentary
The JavaScript snippet listens for changes in the TinyMCE editor. When content is updated, it automatically saves the state. On submission, it fetches the content and sends it to the Java backend. Make sure the content type aligns with what your server expects for accurate processing.
Troubleshooting Integration Issues
Even seasoned developers encounter hiccups during integration. For instance, plugin conflicts or configuration mistakes could lead to unexpected behaviors. This is where resources like "Troubleshooting Common Issues in TinyMCE 6 Plugins with React" can provide insight and solutions.
Common Problems and Solutions
- Incorrect Selector: Ensure that the selector used in TinyMCE matches that of your textarea.
- Response Handling: After submitting, always check server logs for debugging any submission issues.
- Plugin Initialization Failure: Double-check the plugin integration during the TinyMCE setup.
Best Practices for TinyMCE Integration
To ensure a seamless experience with TinyMCE in Java applications, consider these best practices:
1. Minimize HTTP Requests
Load CSS and JS files from a single source whenever possible to reduce latency. Consider using a bundler or minifier in your build process.
2. Safety and Security
Always validate user input to shield against malicious content. Utilize libraries like OWASP Java HTML Sanitizer for this purpose.
3. Stay Updated
Keep track of updates to TinyMCE and its plugins. Regularly check the TinyMCE documentation for new features and changes.
The Closing Argument
Integrating TinyMCE 6 into Java applications enhances user experience significantly. By properly setting it up and utilizing the above practices, you can streamline your integration process. From implementing rich text editing capabilities to handling content submissions effectively, TinyMCE is a powerful tool that can elevate your application’s functionality.
Remember, troubleshooting is part and parcel of development. Refer back to the resources available, including the article on Troubleshooting Common Issues in TinyMCE 6 Plugins with React to help mitigate any challenges during the integration process.
Happy coding!