Mastering Java Frameworks: Overcoming Learning Curve Hurdles
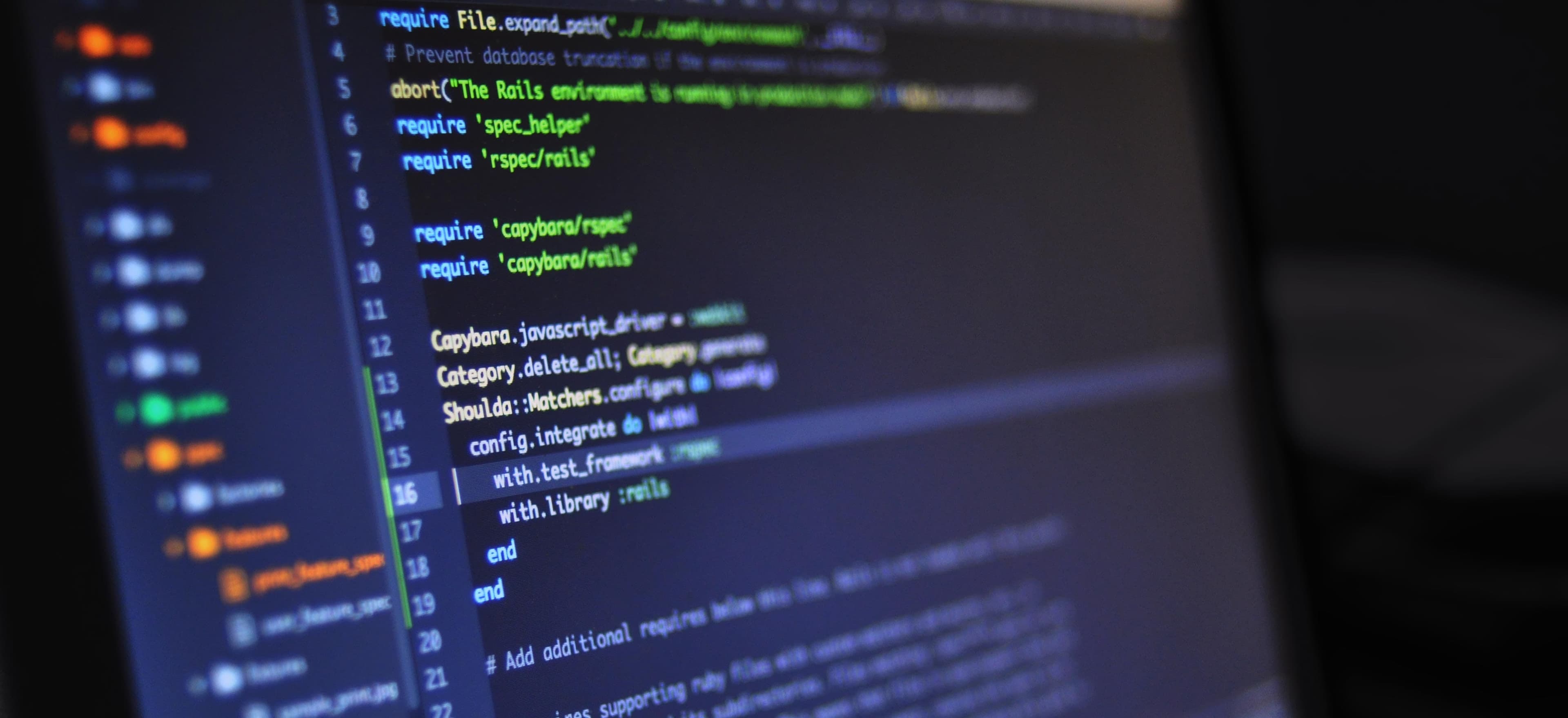
- Published on
Mastering Java Frameworks: Overcoming Learning Curve Hurdles
Java is one of the most versatile programming languages in the world, used for everything from web applications to mobile applications and enterprise-level software. However, beginners and even seasoned developers often encounter a significant barrier when trying to master Java frameworks. This article dives deep into common hurdles and effective strategies to overcome them, ensuring your journey into the Java ecosystem is as smooth as possible.
Understanding Java Frameworks
Before diving into the specifics, it's essential to understand what a Java framework is. A framework provides a structure and set of best practices for building applications, allowing developers to focus on business logic instead of boilerplate code. Java frameworks come in various shapes and sizes; some popular ones include:
- Spring: A comprehensive framework for building enterprise applications.
- Hibernate: An object-relational mapping framework for database interactions.
- JavaServer Faces (JSF): A component-based UI framework for web applications.
By leveraging these frameworks, developers can increase productivity and enhance application quality.
Common Learning Curve Challenges
-
Complexity of Frameworks: Many Java frameworks tend to be complex and require a deep understanding of various underlying concepts, such as Dependency Injection in Spring or JPA in Hibernate.
-
Documentation Overload: While good documentation is crucial, excessive or overly complex documentation can overwhelm new learners.
-
Abstraction Hurdles: Frameworks often abstract many details away from the developer, making it hard to understand how things work under the hood.
-
Community Support Variability: Some frameworks have thriving communities while others may struggle with providing adequate resources for beginners.
Strategies to Overcome Learning Curve Hurdles
1. Start with Basics
Before diving deep into a framework, ensure you have a solid understanding of core Java principles. Topics to focus on include:
- Object-Oriented Programming (OOP)
- Java Collections Framework
- Exception Handling
Familiarizing yourself with these concepts can make it easier to grasp more complex frameworks.
2. Choose One Framework
Don't overwhelm yourself by trying to learn multiple frameworks at once. Pick a framework that aligns with your project needs. For new developers, Spring is a popular choice due to its extensive documentation and large community support.
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class HelloWorld {
public static void main(String[] args) {
// Creating a Spring Application Context
ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml");
// Retrieving a bean from the application context
HelloWorld helloWorld = (HelloWorld) context.getBean("helloWorld");
helloWorld.getMessage();
}
public void getMessage() {
System.out.println("Hello, World!");
}
}
Commentary: The code snippet demonstrates a basic Spring application context and bean retrieval, illustrating how dependency injection works within the Spring framework.
3. Follow Tutorials and Online Courses
There is a plethora of resources available online for learning Java frameworks. Websites such as Coursera, edX, and Udacity offer curated courses that walk you through a framework.
YouTube Channels
YouTube channels like "Java Brains" and "Telusko" provide practical tutorials that can enhance your understanding.
4. Read and Analyze Code
Often, analyzing existing code is one of the best ways to learn. Look for open-source projects on platforms like GitHub that use your chosen framework.
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class User {
@Id
private Long id;
private String name;
// Getters and setters...
}
Commentary: In this example, we see an entity class in Hibernate. Understanding how annotations simplify Object-Relational Mapping (ORM) can help illustrate the framework's ease of use.
5. Engage with Communities
Participating in developer communities can accelerate your learning curve. Websites like Stack Overflow and Reddit's Java community are great places to ask questions and learn from others' experiences.
6. Practical Use Cases
Concrete projects give a practical perspective on how frameworks operate and fit into workflows. Consider building a small web application using Spring Boot or a data-access layer using Hibernate to deepen your understanding.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Commentary: The above Spring Boot example illustrates how easy it is to get started with a new Spring application, making it a developer-friendly choice.
Reference Existing Articles
If you're struggling with frameworks, you're not alone. In an article titled “Why React Relay’s Learning Curve Still Frustrates Developers”, the challenges that come with navigating complex libraries and frameworks are highlighted. The insights from such discussions can be applied to Java frameworks as well, emphasizing the universality of these learning curves.
7. Maintain a Growth Mindset
The journey of mastering Java frameworks involves a learning curve. Embrace failures and setbacks as part of the process. Remember, every challenge provides an opportunity to learn something new.
Closing Remarks
Mastering Java frameworks is not an overnight endeavor, but with the right approach, you can overcome the learning curve. Focus on foundational knowledge, choose your frameworks wisely, seek resources, and engage with the community. The rewards of mastering frameworks are significant and will pay off in both your professional and personal development as a software engineer.
Embrace the challenge, and enjoy the journey of learning. Happy coding!
Checkout our other articles