Mastering ELK Stack: Fixing Java Errors on Kubernetes
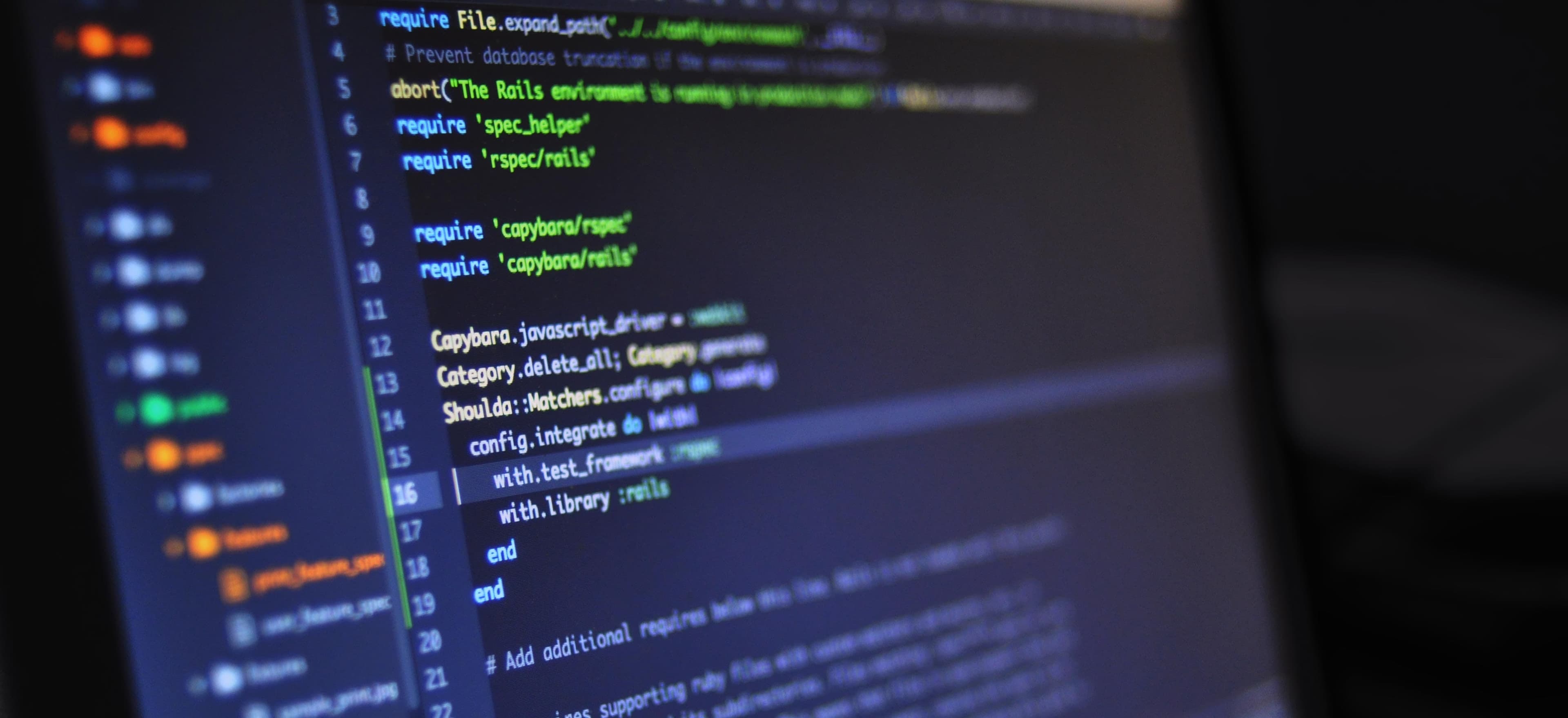
- Published on
Mastering ELK Stack: Fixing Java Errors on Kubernetes
As we embark on the quest to master the ELK stack (Elasticsearch, Logstash, and Kibana) within a Kubernetes environment, it's imperative to understand the complexities involved, especially when working with Java applications. The ELK stack provides powerful tools for centralized logging, which is invaluable in monitoring and troubleshooting Java applications. In this blog post, we will delve into common Java errors that might arise while setting up the ELK stack on Kubernetes and explore effective solutions to tackle these issues.
Understanding the ELK Stack
Before jumping into the specifics of troubleshooting Java errors, let’s take a moment to understand the core components of the ELK stack:
- Elasticsearch: A distributed, RESTful search and analytics engine capable of storing and searching large volumes of data in near real-time.
- Logstash: A server-side data processing pipeline that ingests data from multiple sources, transforms it, and then sends it to a "stash" like Elasticsearch.
- Kibana: A visualization layer that works on top of Elasticsearch, allowing users to interactively explore their data.
By integrating these components, developers can efficiently log, analyze, and visualize application data to make informed decisions. However, the path to achieving a seamless setup can be fraught with errors, especially in a dynamic environment like Kubernetes.
Common Java Errors When Using ELK on Kubernetes
- Connection Issues with Elasticsearch
One of the preliminary hurdles developers often face is ensuring their Java application can connect to the Elasticsearch cluster running on Kubernetes. Connection issues can stem from misconfigurations, service unavailability, or network policies.
Fixing Connection Issues
We'll start by verifying the connection string in your Java application.
public class ElasticSearchClient {
private RestHighLevelClient client;
public ElasticSearchClient(String hostname, int port) {
client = new RestHighLevelClient(
RestClient.builder(new HttpHost(hostname, port, "http"))
);
}
public void close() throws IOException {
client.close();
}
}
In this code, we instantiate a RestHighLevelClient
using the hostname and port of the Elasticsearch service. Ensure that the hostname is resolvable within your Kubernetes cluster, usually done with a DNS entry that points to the service.
- Why This Matters: Proper configuration ensures that your Java application can communicate with Elasticsearch to log data.
- NullPointerExceptions when Sending Logs
Another frequent issue developers notice is NullPointerExceptions
when attempting to send logs from their Java application to Logstash. This usually occurs due to improperly initialized logging frameworks.
Example: Proper Logger Initialization
public class MyApplication {
private static final Logger logger = LoggerFactory.getLogger(MyApplication.class);
public static void main(String[] args) {
try {
// Application logic here
} catch (Exception e) {
logger.error("An error occurred: ", e);
}
}
}
In this example, we initialize a logger which is used to log errors effectively. Ensure that your logger is not null before attempting to log an error.
- Why This Matters: Avoiding null references ensures that your logging operations are robust and do not crash your application.
Configuring Logstash in Your Kubernetes Cluster
As your Java application successfully logs data to Logstash, you'll want to make sure that Logstash is configured properly to handle incoming logs.
Example Logstash Configuration
In your Logstash configuration file (usually logstash.conf
), specify the input settings and filters:
input {
tcp {
port => 5044
codec => json_lines
}
}
filter {
mutate {
add_field => { "source" => "java-logs" }
}
}
output {
elasticsearch {
hosts => ["elasticsearch:9200"]
index => "java-logs-%{+YYYY.MM.dd}"
}
}
This configuration sets up a TCP input on port 5044 and exports the processed logs to Elasticsearch, creating a new index for each day.
- Why This Matters: The correct configuration here ensures that your logs are formatted properly and saved to the appropriate index in Elasticsearch.
Kubernetes Specific Troubleshooting
Kubernetes introduces additional layers of complexity. Configurations for resource limits, health checks, and service discovery must be carefully crafted.
Rolling Updates and Version Compatibility
When updating your application or deploying new versions of your ELK stack, ensure that there are no version compatibility issues. For instance, if you're using a newer version of Java, check if it supports the libraries being used in Logstash and Elasticsearch.
- Tip: Always refer to the official documentation for the respective versions of Java, Elasticsearch, and Logstash you are using to avoid integration issues.
Monitoring Logs with Kibana
Once you've set up ELK correctly, the next step is ensuring that your logs appear in Kibana. This tool allows you to visualize and analyze logs from your Java applications.
- Access Kibana at
http://<kibana-service>:5601
. - Create an index pattern that matches the indices you created (e.g.,
java-logs-*
). - Explore your logs using various visualizations.
Common Kibana Issues
If logs do not appear after following the above steps, check the Elasticsearch logs by running:
kubectl logs <elasticsearch-pod-name>
Look for any error messages that could indicate why logs are not ingested.
Helpful Resources
For additional context and troubleshooting advice, consider reviewing the article titled Troubleshooting Common Issues When Setting Up ELK on Kubernetes. This article dives deep into environmental setups and sheds light on issues you may encounter.
A Final Look
Mastering the ELK stack in conjunction with Java applications on Kubernetes can be daunting, but with the right tools and knowledge, it becomes manageable. Always ensure that your configurations are optimal, your dependencies are in sync, and you have effective logging in place.
As you encounter errors, use the strategies shared in this blog post to troubleshoot effectively. The ELK stack, coupled with Kubernetes, provides a powerful observability platform when configured properly.
Remember, the key to resolving issues is not just about fixing the symptoms but understanding the underlying causes. Happy logging!
Checkout our other articles