Mastering Code Execution: A Guide for Java Frameworks
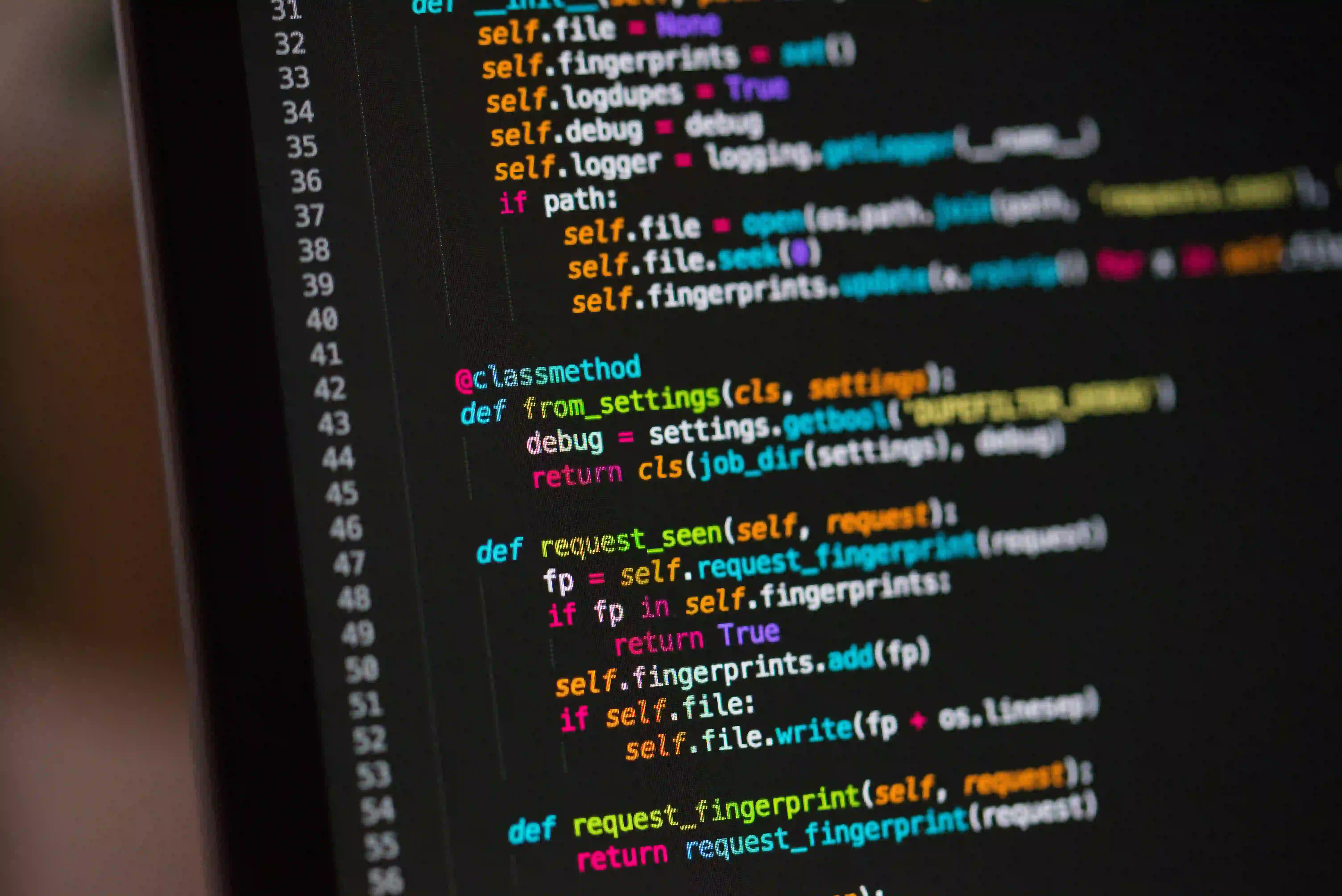
Mastering Code Execution: A Guide for Java Frameworks
In today's fast-paced software development landscape, mastering code execution is essential for developing efficient and maintainable applications. Java, being one of the premier programming languages for server-side applications, offers a plethora of frameworks that ease the development process. This blog post will explore the intricacies of code execution within popular Java frameworks, highlight best practices, and provide actionable insights to help you optimize your development workflow.
Understanding Java Frameworks
Before diving into code execution, let's clarify what we mean by Java frameworks. A framework is a reusable set of libraries or components that helps developers build applications more efficiently. Frameworks such as Spring, Hibernate, and JavaServer Faces (JSF) enable developers to implement complex functionalities with minimal code, streamline their workflow, and maintain cleaner, more scalable applications.
Why Code Execution Matters
Understanding how to control code execution effectively can have a significant impact on your application's performance and security. In a multi-tiered architecture, different parts of your application communicate with one another, making it vital to ensure that this interaction is both seamless and efficient. Poor code execution can lead to bottlenecks, inefficiencies, and vulnerabilities.
For a deeper dive into code execution techniques, check out the article titled Taming the Section Expansion: Controlling Code Execution.
Key Aspects of Code Execution in Java Frameworks
-
Understanding Dependency Injection (DI)
Dependency Injection is a fundamental concept in frameworks like Spring. It allows objects to be created and managed externally rather than within the objects themselves. This makes your code more modular and testable.
Here's a simple example of how DI works in Spring:
☕snippet.java@Component public class UserService { private final UserRepository userRepository; @Autowired public UserService(UserRepository userRepository) { this.userRepository = userRepository; } public User findUserById(Long id) { return userRepository.findById(id); } }
In this example, the
UserService
class depends onUserRepository
. Through the@Autowired
annotation, Spring automatically injects theUserRepository
instance when creatingUserService
.Why This Matters: This decouples your service classes from specific implementations, making unit testing easier by allowing you to mock the
UserRepository
interface. -
Aspect-Oriented Programming (AOP)
AOP complements DI by providing a way to handle cross-cutting concerns such as logging, security, and transaction management without cluttering your business logic.
Here’s a basic example of an AOP aspect in Spring:
☕snippet.java@Aspect @Component public class LoggingAspect { @Before("execution(* com.example.service.*.*(..))") public void logBefore(JoinPoint joinPoint) { System.out.println("Executing: " + joinPoint.getSignature()); } }
This code logs the execution of any method in the specified package before it runs.
Why This Matters: Using AOP can enhance readability and maintainability by separating concerns, allowing you to focus on the core functionality of your application.
-
Leveraging Hibernate for ORM
Object-Relational Mapping (ORM) is another powerful feature that significantly simplifies database interactions. Hibernate is a popular ORM framework in Java that allows developers to map Java classes to database tables effortlessly.
Here’s a straightforward example of using Hibernate:
☕snippet.java@Entity public class Product { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; // Getters and setters... } Session session = sessionFactory.openSession(); Transaction transaction = session.beginTransaction(); session.save(new Product("Sample Product")); transaction.commit(); session.close();
In this code, we define a
Product
entity that represents a table in the database. The session handles the persistence of this entity.Why This Matters: Hibernate abstracts away complex SQL queries, enabling the developer to interact with databases using object-oriented principles, thus speeding up the development process.
Testing Code Execution
Testing is a crucial part of the development lifecycle. Here are some best practices for testing code execution effectively:
-
Unit Testing with JUnit and Mockito
Using JUnit along with Mockito helps you create isolated tests for your code. This is vital for ensuring components function as expected. Here's an example:
☕snippet.java@RunWith(MockitoJUnitRunner.class) public class UserServiceTest { @Mock private UserRepository userRepository; @InjectMocks private UserService userService; @Test public void testFindUserById() { when(userRepository.findById(1L)).thenReturn(new User("John Doe")); User user = userService.findUserById(1L); assertEquals("John Doe", user.getName()); } }
Why This Matters: Isolated unit tests ensure that your code works as intended and maintain reliability during future development iterations.
-
Integration Testing
Integration tests evaluate how different components work together in your application. Frameworks like Spring provide built-in support for this.
☕snippet.java@SpringBootTest public class UserServiceIntegrationTest { @Autowired private UserService userService; @Autowired private TestEntityManager entityManager; @Test public void testIntegration() { User user = new User("Jane Doe"); entityManager.persist(user); User result = userService.findUserById(user.getId()); assertEquals("Jane Doe", result.getName()); } }
Why This Matters: Integration tests can uncover issues related to configurations and interactions between various components, ensuring end-to-end reliability.
Closing Remarks
Mastering code execution across Java frameworks is vital for developing high-performance, maintainable applications. By understanding and leveraging concepts such as Dependency Injection, Aspect-Oriented Programming, and ORM through Hibernate, you can streamline your development process and improve your code quality.
Implementing testing strategies such as unit and integration tests will help you catch issues early, ensuring that your application remains robust and efficient.
For further reading on code execution and its nuances, refer to the article Taming the Section Expansion: Controlling Code Execution. Whether you're a beginner or an experienced developer, there's always something new to learn, and mastering these concepts will undoubtedly enhance your Java skills.
Happy coding!