Bridging the Gap: Overcoming Java Developers' React Relay Hurdles
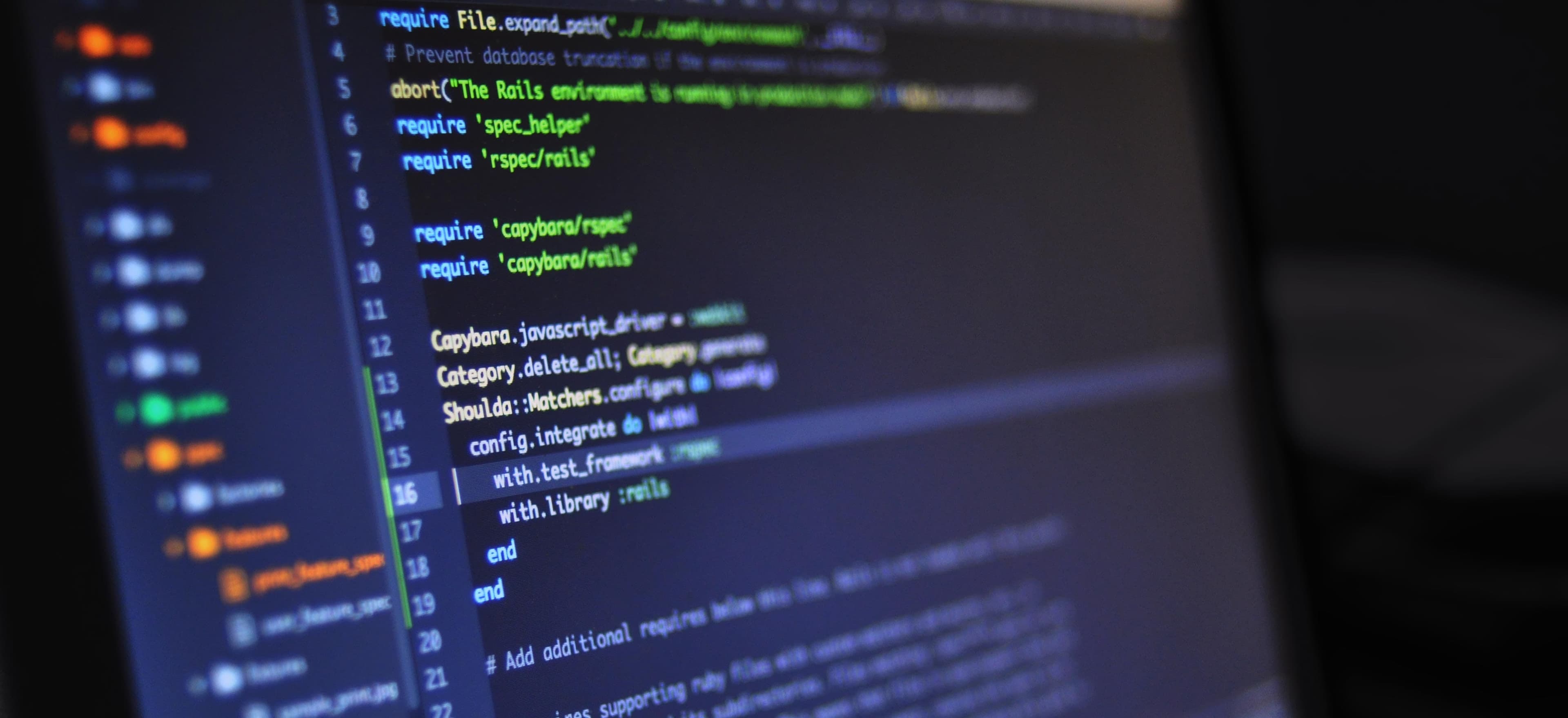
- Published on
Bridging the Gap: Overcoming Java Developers' React Relay Hurdles
As the landscape of web development continues to evolve, many Java developers find themselves navigating through the complexities of modern front-end technologies. A prime example of this shift is seen with React Relay, a framework that streamlines data fetching for React applications. While the benefits are undeniable, developers often face learning curves that can be frustrating. As noted in the article "Why React Relay's Learning Curve Still Frustrates Developers" at infinitejs.com/posts/react-relay-learning-curve-frustrations, understanding Relay is not just about knowing React; it involves grasping a unique set of concepts and paradigms.
This article aims to provide Java developers with insights into React Relay and practical tips on how to bridge the gap effectively. We’ll address common hurdles while highlighting the core principles that make Relay such a powerful tool in a developer's arsenal.
Understanding React Relay
Relay is built on top of React to manage data fetching and state management more efficiently. It introduces concepts such as:
- Declarative Data Fetching: Fetch data as you render components, letting Relay determine the most efficient way to retrieve it.
- GraphQL Integration: Relay works seamlessly with GraphQL, providing a structure for querying data. This is crucial for effective interaction between the front-end and back-end.
- Container Components: These components encapsulate the data dependencies needed by your React components, streamlining data management.
Why Relay is Useful
- Performance: Relay eliminates inefficient data fetching by batching network requests.
- Simplicity: Once understood, Relay reduces the complexity of a component's lifecycle and data management.
- Scalability: Applications using Relay are easier to maintain and scale, particularly with the GraphQL schema.
The Learning Curve
While Relay offers significant benefits, the initial steep learning curve can lead to frustration. Here are the main challenges Java developers face:
Challenge 1: New Paradigms
The declarative nature of Relay, intertwined with GraphQL, contrasts with traditional Java paradigms, resulting in a conceptual shift. Java developers are accustomed to imperative programming and may resist these new paradigms.
Tip: Embrace the shift. Invest time in understanding how declarative data fetching works. Relay’s tooling allows the structure of data requests to become as integral to the application’s design as the components themselves.
Challenge 2: GraphQL Complexity
If you are coming from a Java background, you may have extensive experience with RESTful APIs. Transitioning to GraphQL can feel convoluted due to its query language and structure.
Tip: Start by practicing basic GraphQL queries independently. Understand how queries are structured and how GraphQL differs fundamentally from REST API calls. Here’s an example of a simple query:
{
user(id: "1") {
name
email
}
}
This query fetches the user with an ID of 1
, retrieving only the specified fields, unlike REST which returns entire objects.
Challenge 3: Advanced Tooling
Familiarity with tools and libraries like Babel, Webpack, or Relay's environment can be daunting for developers used to a more traditional Java setup.
Tip: Consider using tools like Create React App, which simplifies project initialization. Start by creating small test applications to get acquainted with different tools without the overwhelm of a full-scale project.
Practical Steps to Master Relay
As you begin integrating Relay into your projects, here are actionable steps to help you gain mastery.
Step 1: Set Up the Environment
To work with Relay in your React project, you'll need to set up your development environment. Here’s a sample code snippet of how you might start:
npx create-react-app my-relay-app
cd my-relay-app
npm install relay-runtime react-relay graphql graphql-tag
This command creates a new React app and installs essential libraries for Relay. These libraries are critical for querying and managing data in your project.
Step 2: Create a Relay Environment
You need to establish a Relay environment to manage the data. Here's an example of how to do this:
// relayEnvironment.js
import { Environment, Network, RecordSource, Store } from 'relay-runtime';
function fetchQuery(operation, variables) {
return fetch('https://myapi.com/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
query: operation.text,
variables,
}),
}).then(response => {
return response.json();
});
}
const environment = new Environment({
network: Network.create(fetchQuery),
store: new Store(new RecordSource()),
});
export default environment;
In this code, we create a network layer that Relay uses to interact with GraphQL. The environment is key for managing data interactions effectively.
Step 3: Create Relay Containers
Relay’s architecture often leverages container components to specify data dependencies. Here’s an example:
import React from 'react';
import { createFragmentContainer, graphql } from 'react-relay';
const UserProfile = ({ user }) => {
return (
<div>
<h1>{user.name}</h1>
<p>{user.email}</p>
</div>
);
};
export default createFragmentContainer(UserProfile, {
user: graphql`
fragment UserProfile_user on User {
name
email
}
`,
});
In this snippet, we use createFragmentContainer
to define a UserProfile component that declares its data requirements using GraphQL fragments. This approach ensures that only the necessary data is fetched, enhancing performance.
Key Takeaways
For many Java developers, transitioning to React Relay may pose challenges, yet the rewards are well worth the effort. By adopting a proactive approach to learning—embracing new paradigms, familiarizing yourself with GraphQL, and utilizing Relay’s powerful features—you’ll find that the initial hurdles become manageable obstacles.
As the web development ecosystem continues to grow, mastering tools like React Relay equips you with flexibility and performance to tackle modern challenges. Despite the learning curve noted in the article "Why React Relay's Learning Curve Still Frustrates Developers," Java developers can thrive with consistent practice and resource usage, ultimately bridging the gap between traditional and modern development paradigms.
Further your understanding by exploring Relay’s official documentation here, and don’t hesitate to share insights and experiences with your developer community. Happy coding!
Checkout our other articles