Mastering Java: Control Expanding Sections in Your Code
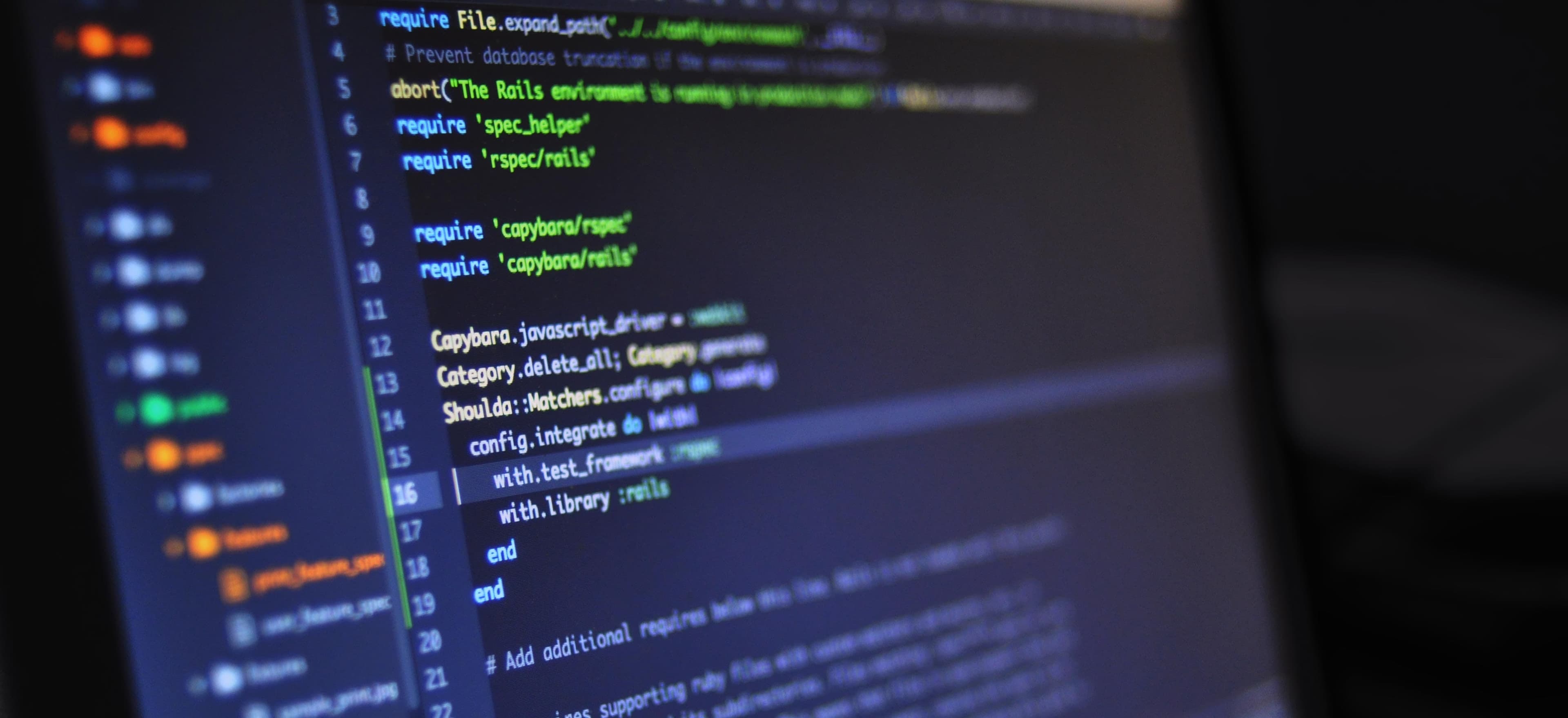
- Published on
Mastering Java: Control Expanding Sections in Your Code
Java, a programming language recognized for its verbosity and readability, offers a wide array of features that allow developers to create robust applications. However, managing complex code structures can sometimes lead to difficulties, especially when it comes to controlling code execution and organization within sections. In this guide, we will explore various techniques to enhance your Java code's structure and readability by addressing how to effectively manage expanding sections.
Why Care About Code Structure?
The foundation of any software project lies in its code structure. Well-structured code not only enhances readability but also makes maintenance and debugging easier. Here are several reasons why focusing on controlling expanding sections in Java is vital:
- Readability: Clear code is easier to read and understand.
- Maintainability: Well organized code reduces the complexity of future modifications.
- Efficiency: Proper structure can lead to better performance during runtime.
- Collaboration: In teams, structured code enhances collaboration by making each part of the logic comprehensible to others.
To further delve into the topic, take a look at an insightful article titled "Taming the Section Expansion: Controlling Code Execution". It discusses various techniques in which one can effectively manage section expansions in your code.
Structuring Your Java Code
Java provides several tools and practices to control how your code is structured. Below are some approaches you can adopt to handle expanding sections.
1. Using Methods for Code Abstraction
One effective way to control expanding sections in Java is through the use of methods. Methods allow you to break down complex logic into manageable parts.
Example:
public class TaxCalculator {
public double calculateTax(double income) {
double taxRate = getTaxRate(income);
return income * taxRate;
}
private double getTaxRate(double income) {
if (income < 10000) {
return 0.1; // 10%
} else if (income < 50000) {
return 0.2; // 20%
} else {
return 0.3; // 30%
}
}
}
Commentary:
In this example, the calculateTax
method abstracts the logic for tax calculation. It delegates the responsibility of determining the tax rate to the getTaxRate
method. This not only keeps the method focused on a single task but also allows for easier updates in the future.
2. Leveraging Comments and Annotations
Proper comments can act as road signs in your code, guiding others (or yourself in the future) through your logical thought process. Java supports annotations that can help to categorize various areas of your code.
Example:
public class UserService {
// This method fetches user information from the database
public User getUser(int userId) {
// Assume database connection established
return database.findUserById(userId);
}
}
Commentary: In the example above, the comment before the method explains its purpose. By using comments strategically, you can control the understanding of your code sections, thus preventing confusion that can arise from expanding sections of logic.
3. Utilizing Control Structures Wisely
Control structures such as if-else statements, switch cases, and loops can create sections that may expand excessively. Organizing them effectively can make your code cleaner and more readable.
Example:
public void printUserRole(User user) {
String role;
switch (user.getRole()) {
case ADMIN:
role = "Admin";
break;
case USER:
role = "User";
break;
default:
role = "Guest";
break;
}
System.out.println("The user role is: " + role);
}
Commentary: By using a switch case instead of multiple if-else statements, the control structure remains concise. This method allows for easy expansion in the future, as adding new cases is straightforward without cluttering the logic.
4. Encapsulating Code with Classes and Interfaces
Java's object-oriented paradigm allows you to encapsulate sections of code in classes or interfaces. Using this approach helps in controlling the expansion of code and understanding its relationships with other code sections.
Example:
public interface Payment {
void processPayment(double amount);
}
public class CreditCardPayment implements Payment {
@Override
public void processPayment(double amount) {
// Logic to process credit card payment
}
}
public class PayPalPayment implements Payment {
@Override
public void processPayment(double amount) {
// Logic to process PayPal payment
}
}
Commentary:
In the above example, implementing the Payment
interface allows for different payment methods without expanding the code excessively. Each payment method has its class, keeping its logic well-organized and separated.
5. Making Use of Design Patterns
Design patterns like Factory and Strategy can help control the flow and execution of code sections while keeping them modular.
Example:
public class PaymentFactory {
public static Payment getPaymentMethod(String paymentType) {
if (paymentType.equals("CREDIT_CARD")) {
return new CreditCardPayment();
} else if (paymentType.equals("PAYPAL")) {
return new PayPalPayment();
}
return null;
}
}
Commentary:
In the PaymentFactory
, logic is separate from payment method implementation. This reduces dependencies and controls the expansion of payment processing logic effectively.
Best Practices for Controlling Code Expansion
- Refactor Regularly: Make it a habit to revisit code and refactor it to maintain readability.
- Adopt a Coding Standard: Stick to a consistent coding standard across the project.
- Limit Lines of Code: Aim to keep methods small—about 20 lines or fewer can promote clarity.
- Avoid Deep Nesting: Too many nested structures can lead to confusion. Try to flatten your code.
- Design for Change: Always consider how you might need to expand code in the future and build flexibility into your design.
Final Thoughts
Mastering the structure and organization of your code can seem daunting at first, but by controlling expanding sections in Java, you can create more efficient, readable, and maintainable applications. Implementing methods, comments, control structures, encapsulation, and design patterns are powerful ways to achieve this.
Moreover, consistently refactoring and adhering to coding standards will keep your code clean and adaptable to future changes. Don’t forget to read more about managing your code effectively by checking out the relevant article, "Taming the Section Expansion: Controlling Code Execution".
By taking these practices to heart, you’ll not only improve your programming skills but also contribute positively to any project you are part of. Happy coding!
Checkout our other articles