Mastering Java File Uploads: Avoiding Azure Blob Storage Pitfalls
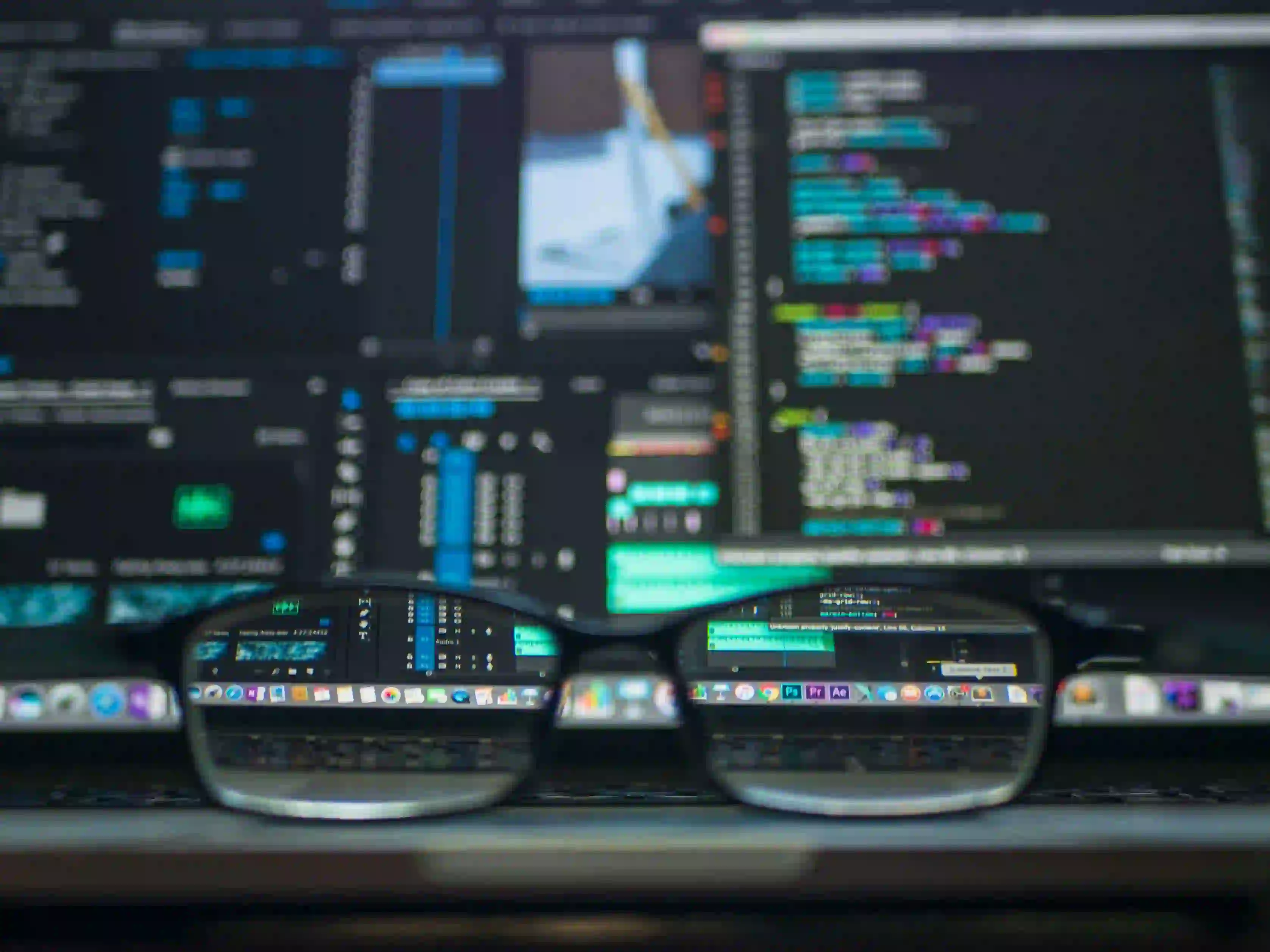
Mastering Java File Uploads: Avoiding Azure Blob Storage Pitfalls
File uploads are a core functionality in many web applications, enabling users to share and store files in the cloud. When utilizing platforms like Azure Blob Storage for file uploads, developers may encounter specific challenges. In this article, we will explore how to effectively manage file uploads in Java while steering clear of common pitfalls. We will reference insights from our existing article, "Common pitfalls to avoid when hosting on Azure Blob Storage".
Understanding Azure Blob Storage
Azure Blob Storage is a service for storing large amounts of unstructured data. The Blob in Azure Blob Storage stands for "Binary Large Object," and it can include text, images, videos, and other files. This service is highly scalable and designed for efficient access and management of large files.
We can classify blob storage into three types:
-
Block Blobs: Ideal for text and binary data. Great for uploading files like documents and images.
-
Append Blobs: Optimized for append operations. Suitable for scenarios where data is constantly being written, like logging.
-
Page Blobs: Designed for random access and suited for virtual hard disks (VHDs).
When choosing which type of blob to use for file uploads, consider the file size and intended access patterns.
Setting Up Your Java Project
Before we get into the code, ensure you have the required dependencies. If you're using Maven, add these to your pom.xml
:
<dependency>
<groupId>com.azure</groupId>
<artifactId>azure-storage-blob</artifactId>
<version>12.14.0</version>
</dependency>
This library provides necessary features to work with Azure Blob Storage in Java.
Establishing a Connection to Azure Blob Storage
Creating a Blob Service Client
To begin uploading files, you need to establish a connection to Azure Blob Storage. Here’s how to create a service client:
import com.azure.storage.blob.*;
import com.azure.storage.blob.models.BlobContainerItem;
public class AzureBlobService {
private static final String CONNECTION_STRING = "<Your_Connection_String>";
private BlobServiceClient blobServiceClient;
public AzureBlobService() {
blobServiceClient = new BlobServiceClientBuilder()
.connectionString(CONNECTION_STRING)
.buildClient();
}
public BlobServiceClient getBlobServiceClient() {
return blobServiceClient;
}
}
Explanation of Code
- The
AzureBlobService
class initializes aBlobServiceClient
using your connection string. This client serves as the primary interface for working with blobs and containers in Azure. - Be sure to replace
<Your_Connection_String>
with your actual Azure Blob Storage connection string.
Creating a Blob Container
Before uploading files, ensure the blob container exists. If not, create one:
public void createContainer(String containerName) {
BlobContainerClient containerClient = blobServiceClient.getBlobContainerClient(containerName);
if (!containerClient.exists()) {
containerClient.create();
System.out.println("Container created: " + containerName);
} else {
System.out.println("Container already exists: " + containerName);
}
}
Key Points
getBlobContainerClient(containerName)
retrieves a reference to the specified container.exists()
checks if the container already exists, preventing unnecessary creations and potential errors.
Uploading Files to Blob Storage
Here is the core functionality when it comes to file uploads:
import java.nio.file.Path;
import java.nio.file.Paths;
public void uploadFile(String containerName, String filePath) {
BlobContainerClient containerClient = blobServiceClient.getBlobContainerClient(containerName);
String blobName = Paths.get(filePath).getFileName().toString();
BlobClient blobClient = containerClient.getBlobClient(blobName);
blobClient.uploadFromFile(filePath);
System.out.println("File uploaded: " + blobName);
}
Code Explanation
Paths.get(filePath).getFileName().toString()
retrieves just the filename from the provided path, which will serve as the name of the blob in storage.uploadFromFile(filePath)
is used to upload the specified file directly to the blob container.
Handling Errors and Exceptions
When dealing with external services like Azure, error handling is crucial. Here is an approach to capture exceptions during uploads:
public void uploadFileWithValidation(String containerName, String filePath) {
try {
uploadFile(containerName, filePath);
} catch (BlobStorageException e) {
System.err.println("Error uploading file: " + e.getMessage());
} catch (Exception e) {
System.err.println("An unexpected error occurred: " + e.getMessage());
}
}
The Importance of Error Handling
BlobStorageException
captures Azure service-related issues, such as networking errors and invalid credentials.- Catching general exceptions ensures your application can handle unforeseen issues gracefully.
Best Practices for File Uploads to Azure Blob Storage
In the course of using Azure Blob Storage, developers often face certain hurdles. Here are some best practices to follow:
-
Limit File Size: Azure Blob Storage has limits on file sizes. Defining an upper limit helps prevent upload attempts that will fail.
-
Set Timeouts: Establishing timeout configurations ensures your application doesn’t hang indefinitely. This can be done through the client builder.
-
Use Asynchronous APIs: Leveraging asynchronous methods improves responsiveness, especially in web applications where blocking calls can lead to a poor user experience.
-
Implement Retry Logic: Azure Blob Storage may have transient failures. Implement retry logic for robustness against these potential failures.
-
Secure Sensitive Data: Always encrypt files before upload when they contain sensitive information. Azure supports customer-managed keys for encryption.
-
Monitor Performance: Utilize Azure Monitor to keep track of request performance metrics.
For more insights on Azure and potential pitfalls, refer to the article, "Common pitfalls to avoid when hosting on Azure Blob Storage" which dives deeper into aspects like performance, security, and costs.
Lessons Learned
Uploading files to Azure Blob Storage with Java is a straightforward process, but it's essential to approach it with best practices and caution. Implementing error handling, file validation, and efficient coding patterns will equip you to avoid common pitfalls associated with Azure storage solutions.
By mastering these techniques, you can build robust applications that meet your users' needs while ensuring optimal interaction with Azure's services. Whether you're a beginner or an experienced developer, following these guidelines will help you manage file uploads effectively in your Java applications.