Harnessing Java Frameworks for Mindfulness and Productivity
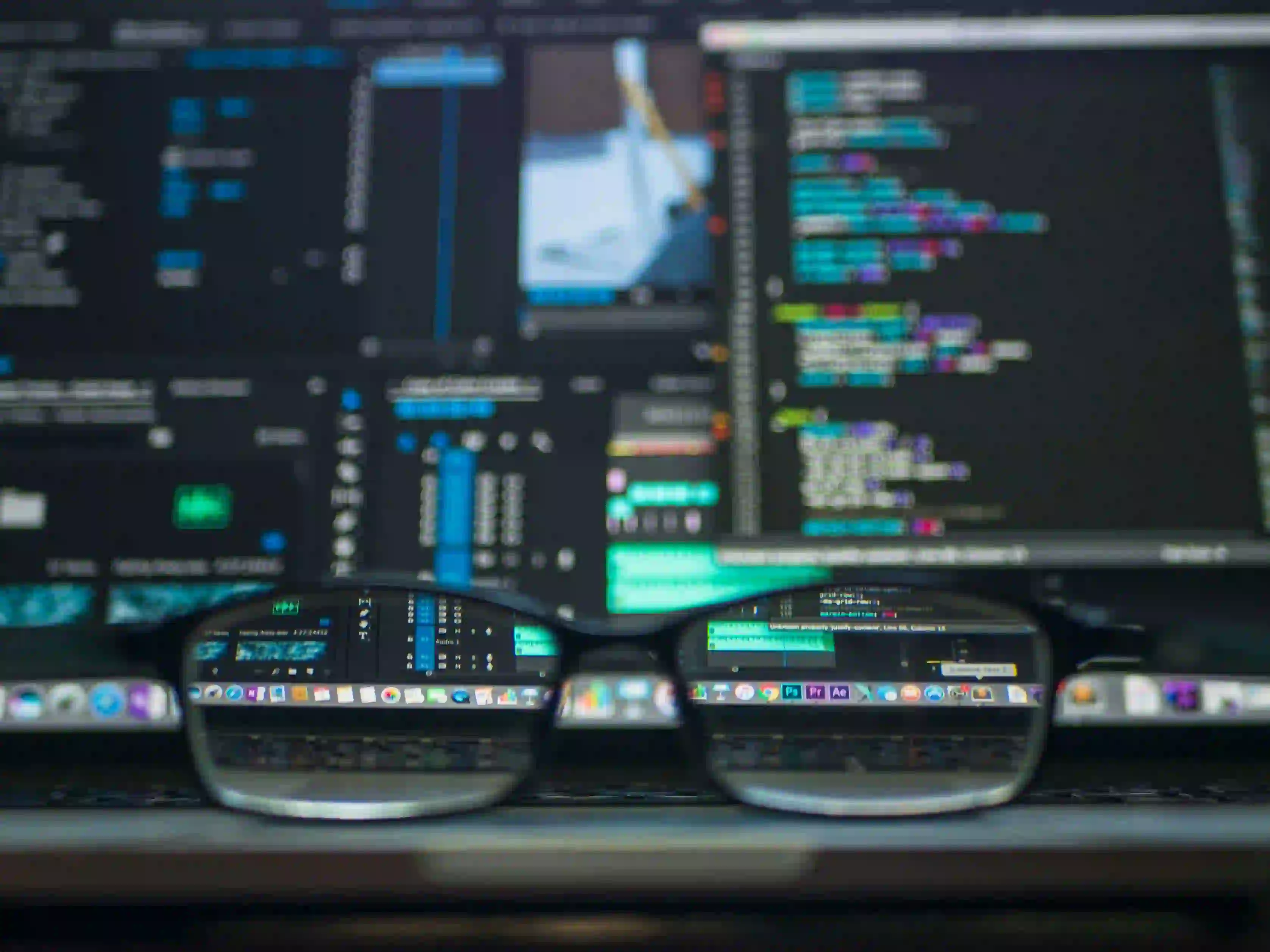
Harnessing Java Frameworks for Mindfulness and Productivity
In today’s fast-paced world, finding a balance between productivity and mindfulness can feel like a tightrope walk. For software developers, navigating complex codebases, tight deadlines, and the influx of new technologies can add to this pressure. However, leveraging Java frameworks can offer not just technical advantages but also a way to cultivate mindfulness within your work habits. Let’s explore how Java frameworks can enhance productivity and how, through mindful development practices, you can boost your efficiency while maintaining mental clarity.
Understanding Mindfulness in Software Development
Mindfulness is the practice of being present in the moment and fully engaging with your current task. Within the software development realm, this could mean focusing on writing clean code or effectively collaborating with your team. By spotlighting this practice, developers can reduce stress and increase productivity. Just as meditation can be a tool for mindfulness, Java frameworks can be employed to streamline development processes, making our work more manageable.
a. The Benefits of Using Java Frameworks
Java frameworks like Spring, Hibernate, and JavaServer Faces (JSF) are designed to simplify complex tasks, enabling developers to achieve more with less effort. Here’s a brief overview of their benefits:
-
Code Reusability: Frameworks encourage code reuse, allowing you to focus on creating unique features instead of rewriting boilerplate code.
-
Improved Productivity: With less time spent on mundane tasks, developers can focus on problem-solving, thereby enhancing productivity.
-
Community Support: Well-established frameworks often have robust community support, enabling quick problem resolution through forums and documentation.
Diving into Key Java Frameworks
Let’s take a closer look at some of the most popular Java frameworks and how they can be harnessed to improve productivity.
Spring Framework
The Spring Framework is one of the most used frameworks in Java development. It's flexible, powerful, and can be used for a variety of applications.
Code Example: Simple Spring Boot Application
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Why use Spring?
This simple code snippet demonstrates the power of Spring Boot, a module within the Spring Framework. The @SpringBootApplication
annotation makes it easy to set up a Spring application. It combines several configurations into one, significantly reducing the amount of setup needed.
Hibernate
Hibernate is an object-relational mapping (ORM) framework that simplifies database interactions in Java applications. Instead of dealing with SQL queries directly, developers interact with Java objects.
Code Example: Basic Hibernate Configuration
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateUtil {
private static final SessionFactory sessionFactory = buildSessionFactory();
private static SessionFactory buildSessionFactory() {
try {
return new Configuration().configure().buildSessionFactory();
} catch (Throwable ex) {
throw new ExceptionInInitializerError(ex);
}
}
public static SessionFactory getSessionFactory() {
return sessionFactory;
}
}
Why use Hibernate?
In this example, you see how easy it is to set up a session factory that manages database operations with minimal configuration. This reduces the need to write repetitive SQL code, allowing developers to focus on application logic instead.
JavaServer Faces (JSF)
JSF is a powerful framework for building user interfaces for Java applications. It allows developers to create reusable UI components.
Code Example: JSF Managed Bean
import javax.faces.bean.ManagedBean;
@ManagedBean
public class HelloWorld {
private String message;
public HelloWorld() {
message = "Hello, World!";
}
public String getMessage() {
return message;
}
}
Why use JSF?
This simple managed bean represents a typical JSF component. By keeping UI interactions separated from business logic, you can easily maintain and test each component, promoting a cleaner codebase and enhancing the mindfulness of your workflow.
Finding Mindfulness Through Development Practices
While frameworks can assist with productivity, it’s essential to incorporate mindfulness into your coding practices. Here are a few strategies:
-
Set Clear Goals: Each day, define what you aim to achieve. This helps maintain your focus.
-
Regular Breaks: Schedule short breaks to recharge. Returning to your work with a refreshed mind can lead to better problem-solving.
-
Limit Multitasking: Focus on one task at a time. This aligns with the principles discussed in “Strom der Stille: Energiegewinn durch Meditation” (auraglyphs.com/post/strom-stille-energiegewinn-meditation), emphasizing how a single-minded focus can yield better results.
-
Practicing Review: At the end of each work session, review what you accomplished and how you can improve. This practice promotes a mindset of continuous growth.
My Closing Thoughts on the Matter
In conclusion, Java frameworks not only enhance productivity through their powerful features but also serve as a medium to cultivate mindfulness in software development. By employing frameworks like Spring, Hibernate, and JSF, developers can reduce repetitive tasks and focus on higher-level problem solving.
Combining technical efficiency with mindful practices can lead to a more fulfilling and less stressful development experience. As we navigate the evolving programming landscape, let us not forget the importance of balance between work and mindfulness.
As you integrate these frameworks into your workflow, remember the advice from the article “Strom der Stille: Energiegewinn durch Meditation.” Cultivating mindfulness will elevate not just your coding skills but also your overall quality of life in the tech world.
Do you have favorite Java frameworks or practices that help you maintain productivity? Share your thoughts in the comments below!