Common Spring Testing Pitfalls and How to Avoid Them
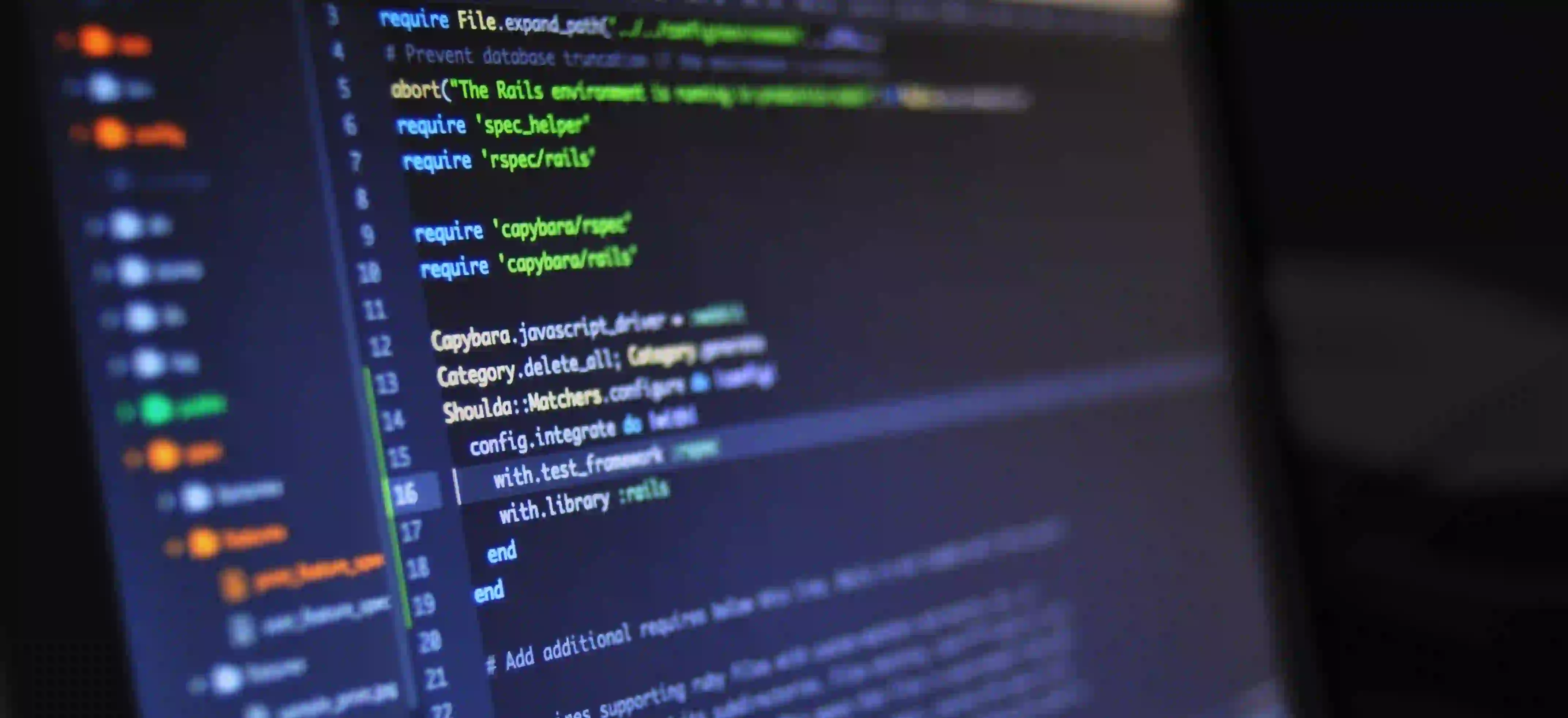
Common Spring Testing Pitfalls and How to Avoid Them
Spring Framework is widely used for building enterprise-level applications in Java. With its comprehensive testing support, developers can ensure that their applications work as intended. However, there are several common pitfalls that developers encounter while testing Spring applications. This blog post will discuss these pitfalls and provide strategies to avoid them.
Understanding the Importance of Testing in Spring
Testing is crucial in software development. It not only helps to identify bugs but also ensures that the system works as expected. In the context of Spring applications, testing allows developers to validate the configuration, dependency injection, and behavior of components under various conditions.
Why is Spring Testing Different?
Spring testing is different due to its reliance on the Spring container for dependency resolution and management. Ensuring that these dependencies are correctly injected and function as expected can sometimes lead to complex testing scenarios.
Common Pitfalls
Let’s delve into some common pitfalls and how to avoid them, along with illustrative code snippets.
1. Not using @SpringBootTest Properly
The @SpringBootTest
annotation allows you to bootstrap the entire application context for integration testing. However, overusing this annotation can lead to slow tests and increased complexity.
Avoidance Strategy:
- Use testing slices like
@WebMvcTest
,@DataJpaTest
, or@MockBean
when possible, as they provide a more focused context for your tests.
Example: Using @WebMvcTest
@RunWith(SpringRunner.class)
@WebMvcTest(YourController.class)
public class YourControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private YourService yourService;
@Test
public void testYourEndpoint() throws Exception {
when(yourService.getData()).thenReturn(new YourData());
mockMvc.perform(get("/your-endpoint"))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON));
}
}
Why: Using @WebMvcTest
limits the context to only web-related components, improving the performance and focus of your tests.
2. Ignoring Profile-Specific Tests
Spring supports multiple profiles to configure different environments. However, many developers forget to specify the appropriate profile during testing, leading to unexpected failures.
Avoidance Strategy:
- Use the
@ActiveProfiles
annotation to load the correct profile when running tests.
Example: Specifying Active Profiles
@RunWith(SpringRunner.class)
@SpringBootTest
@ActiveProfiles("test")
public class YourServiceTest {
...
}
Why: This ensures that your tests run under the correct configurations, avoiding inconsistencies that could cause failures.
3. Lack of Isolation in Tests
Usually, tests should be isolated from one another to avoid side effects. Failing to isolate tests can lead to failures that are difficult to trace back to specific causes.
Avoidance Strategy:
- Use
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
to create a new instance of your test class for each test case, and ensure proper cleanup.
Example: Isolating Tests
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
public class YourServiceTest {
@Autowired
private YourService yourService;
@BeforeEach
public void setup() {
// setup operations, such as resetting mocks or initializing test data
}
@Test
public void testMethodA() {
// Assertions for method A
}
@Test
public void testMethodB() {
// Assertions for method B
}
}
Why: This helps maintain a clean state for each test, improving reliability and readability.
4. Not Using Mocking Frameworks
When tests interact with external services, it is crucial to isolate these interactions. Not using mocking frameworks such as Mockito, can lead to flaky tests and dependency on external systems.
Avoidance Strategy:
- Use
@MockBean
to mock external dependencies.
Example: Mocking External Services
@RunWith(SpringRunner.class)
@WebMvcTest(YourController.class)
public class YourControllerTest {
@MockBean
private ExternalService externalService;
@Test
public void testEndpointWithMock() throws Exception {
when(externalService.fetchData()).thenReturn(new DataResponse());
mockMvc.perform(get("/endpoint"))
.andExpect(status().isOk());
}
}
Why: This removes the dependency on the actual service, providing a more controlled testing environment and increasing test speed.
5. Neglecting Asynchronous Code Testing
Spring provides support for asynchronous processing with @Async
annotations. Developers often overlook how to test such operations, leading to missed bugs.
Avoidance Strategy:
- Use the CompletableFuture and MockMvc to test async endpoints.
Example: Testing Asynchronous Methods
@GetMapping("/async")
public CompletableFuture<ResponseEntity<String>> asyncMethod() {
return CompletableFuture.supplyAsync(() -> "Hello");
}
@Test
public void testAsyncMethod() throws Exception {
mockMvc.perform(get("/async"))
.andExpect(status().isOk());
}
Why: This ensures you are verifying the asynchronous behavior properly, confirming that your application's flow remains intact.
6. Poorly Named Test Cases
Clear naming conventions for test methods are often disregarded. Poorly named tests can lead to confusion when diagnosing issues.
Avoidance Strategy:
- Follow a clear naming pattern that reflects the purpose and the expected outcome of the test.
Example: Naming Conventions
@Test
public void shouldReturn404WhenUserNotFound() {
// Test code to assert 404 status for not found user
}
Why: Clear naming makes your test cases self-descriptive, aiding readability and maintainability for other developers.
Wrapping Up
Testing in Spring is essential yet fraught with common pitfalls that can undermine your application’s reliability. Understanding these pitfalls and employing strategies to avoid them ensures that your testing efforts yield maximum benefits.
Whether you are preparing tests for a controller, service, or repository, keeping these considerations in mind will help you create a robust testing strategy that enhances the quality of your Spring application.
For further reading, check out the Spring Testing Documentation and explore advanced testing techniques with Mockito.
By being vigilant about these common pitfalls and actively working to avoid them, you can create more effective tests that not only catch bugs but also ensure that your Spring applications deliver high performance and reliability.