Mastering Java Streams: The Yield-Like Functionality You Need
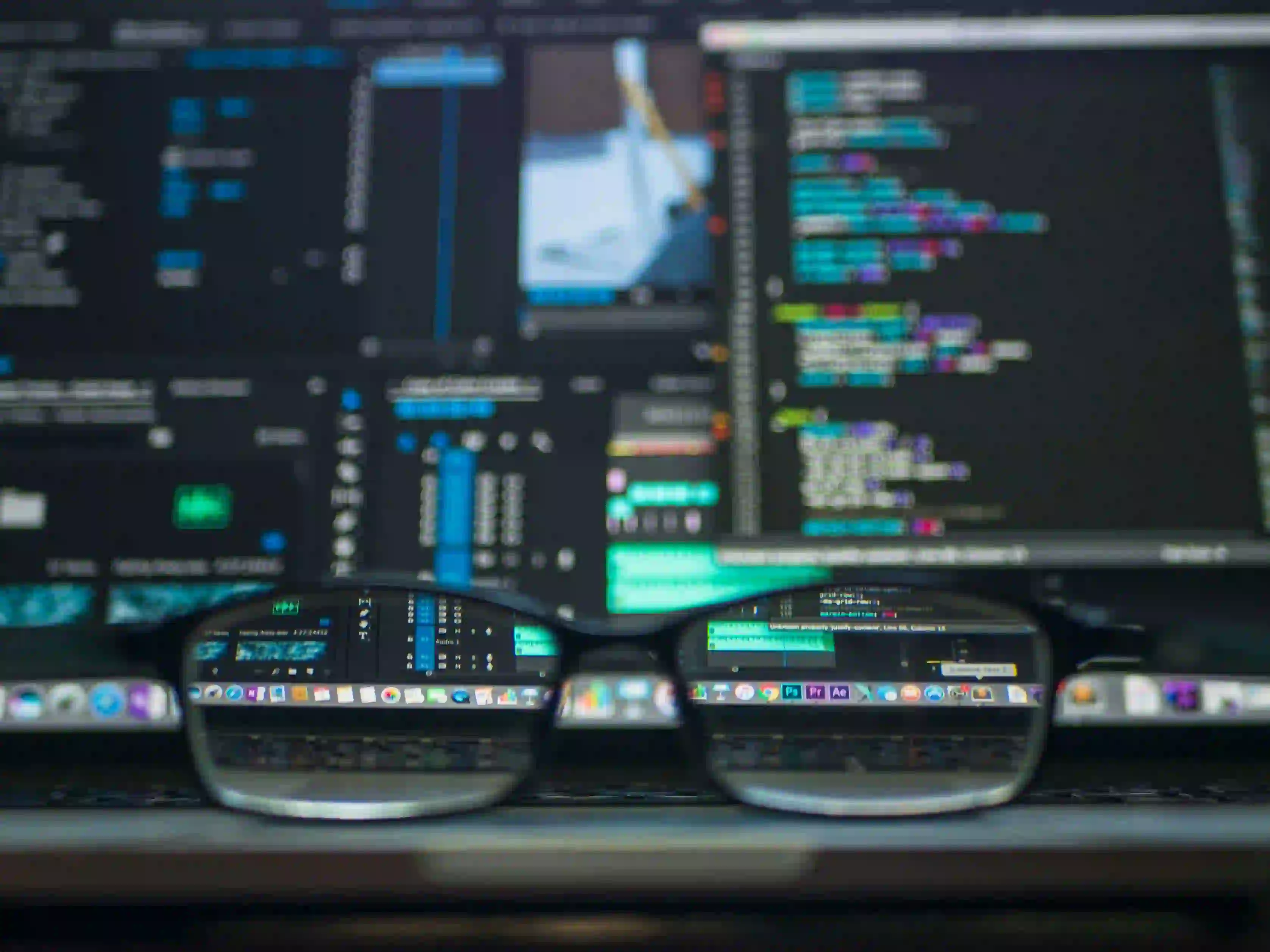
Mastering Java Streams: The Yield-Like Functionality You Need
Java has continuously evolved since its inception, incorporating new features that simplify and enhance its functionality. One of the most significant additions in Java 8 is the Stream API, which allows developers to process collections of objects in a functional programming style. This blog post will dive deep into Java Streams, exploring their yield-like functionality and demonstrating how you can leverage this feature to write cleaner, more efficient code.
Understanding Java Streams
Java Streams provide a means to process sequences of elements (like collections) in a declarative manner. Unlike traditional iteration methods that require mutable iterations, Streams focus on the "what" instead of the "how." This helps in producing code that is easier to understand and maintain.
Benefits of Using Streams
- Conciseness: Stream operations can often be expressed in fewer lines of code.
- Parallel Processing: Streams can be processed in parallel effortlessly, utilizing multi-core architectures.
- Chaining: Stream operations can be chained in a readable manner, promoting a flow of operations.
Basic Structure of Streams
At its core, a Stream is a sequence of elements that supports various operations to process those elements. Java Stream API provides methods to create a stream, perform intermediate operations (like filtering), and terminal operations (like collecting).
Yield-Like Functionality with Streams
In many programming languages, the yield
keyword plays a crucial role in producing values from a generator function. While Java Streams do not have a yield
construct, the combination of map
, filter
, and flatMap
operations can mimic its behavior effectively.
The map Function
The map
function is used to transform each element in a Stream into another form. For instance, suppose you have a list of integers that you want to square:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squares = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
// Result: [1, 4, 9, 16, 25]
Why Use Map?: The map
function is beneficial because it allows you to apply a function to each element, transforming the entire Stream in a readable and concise way.
The filter Function
The filter
method allows you to select elements based on a specific condition. For example, here's how to filter out even numbers:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> oddNumbers = numbers.stream()
.filter(n -> n % 2 != 0)
.collect(Collectors.toList());
// Result: [1, 3, 5]
Why Use Filter?: Filtering is vital for refining a dataset based on specific criteria, enabling you to focus on the elements that are relevant to your application.
Combining Map and Filter
You can combine map
and filter
to produce a new stream that processes data like a generator with yield functionality.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> squaredOddNumbers = numbers.stream()
.filter(n -> n % 2 != 0)
.map(n -> n * n)
.collect(Collectors.toList());
// Result: [1, 9, 25]
This combination demonstrates how you can produce a filtered set of transformed elements without worrying about mutable state.
FlatMap for Flattening Collections
The flatMap
function provides a method for transforming elements into multiple elements, similar to yielding multiple values. FlatMap is essential when dealing with nested collections, such as a list of lists.
Consider the example of a list of sentences:
List<String> sentences = Arrays.asList("Hello world", "Java Streams are fun", "Stream processing is powerful");
List<String> words = sentences.stream()
.flatMap(sentence -> Arrays.stream(sentence.split(" ")))
.collect(Collectors.toList());
// Result: [Hello, world, Java, Streams, are, fun, Stream, processing, is, powerful]
Why Use FlatMap?: It effectively handles scenarios where each input can correspond to multiple outputs, flattening them into a single stream.
Parallel Processing with Streams
One of the most powerful features of Java Streams is the ability to process data in parallel. By simply converting a Stream to a parallel stream, you can exploit multiple CPU cores without worrying about thread management:
List<Integer> numbers = IntStream.rangeClosed(1, 1_000_000)
.boxed()
.parallel()
.map(n -> n * n)
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
Why Use Parallel Streams?: They allow you to perform operations significantly faster, especially with large datasets.
When Not to Use Streams
Though Java Streams offer numerous advantages, they are not a panacea. Here are a few cases where Streams might not be suitable:
- Complex Logic: If the logic for processing is too complex, using traditional looping can improve readability.
- Side Effects: Streams should be side-effect-free. If your logic needs to modify state, standard for-loops might be a better choice.
- Small Datasets: For small collections, performance overhead from stream creation may outweigh the benefit.
Lessons Learned
Java Streams present a powerful and elegant way to handle collections in a functional manner. Their yield-like behavior through map
, filter
, and flatMap
allows developers to produce readable and efficient code, significantly improving productivity and maintenance.
For further reading on Java Streams, check out the official Java documentation for an in-depth understanding of this feature. If you're interested in exploring functional programming further, the Java 8 in Action book is an excellent resource.
Incorporating Java Streams into your projects not only streamlines your code but also enhances performance, especially in data processing tasks. Start experimenting with Streams today and unlock the full potential of your Java applications!