Why Getters and Setters Can Sabotage Your Code Quality
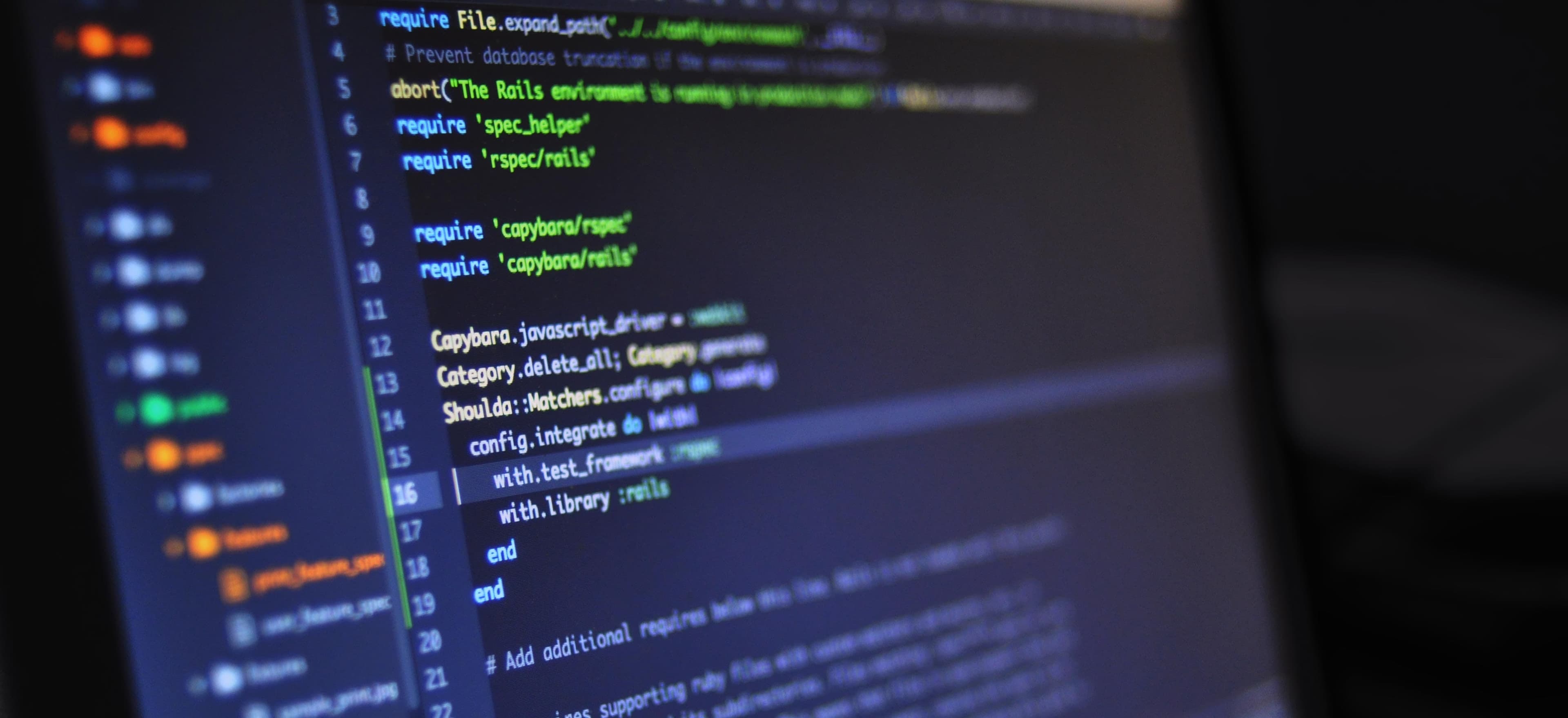
- Published on
Why Getters and Setters Can Sabotage Your Code Quality
In the world of object-oriented programming (OOP), the concepts of getters and setters have established themselves as foundational practices. These methods provide a way to encapsulate the internal state of an object and control access to its fields. However, while they are often seen as a best practice, they can also lead to serious pitfalls that could sabotage your code quality. In this post, we'll explore these drawbacks, discuss alternatives, and provide insight into achieving better design in your Java applications.
Understanding Getters and Setters
Before diving into the pitfalls, it's crucial to define what getters and setters are.
Getting Started with Getters and Setters
A getter is a method that retrieves the value of a specific field of an object. Conversely, a setter allows you to modify that value. Here's a basic example in Java:
public class Person {
private String name;
// Getter
public String getName() {
return name;
}
// Setter
public void setName(String name) {
this.name = name;
}
}
In this code snippet, the getName
method returns the name
attribute, while the setName
method allows the user to set the name.
While getters and setters provide visibility and mutability, they can lead to complications in several ways. Let's discuss these issues.
The Drawbacks of Getters and Setters
1. Exposure of Internal State
By relying heavily on getters and setters, you expose the internal state of your objects, leading to a tightly coupled design.
- Example: If you expose a setter for every field, consumers of your class can make changes to the object's state without any validation or business logic. This leads to potential inconsistencies.
2. Increased Complexity Without Value
Using getters and setters can increase the complexity of code unnecessarily. They might create what is often termed as "anemic domain models," where the only responsibility of a class is to hold data. Such classes lack behavior, which can degrade the whole design.
3. Break Encapsulation
Encapsulation is one of the four pillars of OOP. When you expose fields via getters and setters, you break encapsulation by allowing external code to change the object’s state in arbitrary ways.
- Example: Consider a class representing a bank account:
public class BankAccount {
private double balance;
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
this.balance = balance;
}
}
Here, anyone can set the balance directly, potentially leading to negative balances or other violations of business rules. Instead, consider using methods that enforce the rules of your domain:
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
public void withdraw(double amount) {
if (amount > 0 && amount <= balance) {
balance -= amount;
}
}
4. Overusing Getters and Setters
Over-relying on these methods can cause a snowball effect in your codebase. When code is clean and encapsulated, less is often more. If you provide mutator methods for every field, your class becomes susceptible to modification at any point in the application.
Alternatives to Getters and Setters
To mitigate these pitfalls, consider the following practices that would yield better code quality:
1. Encapsulate Behavior
Instead of accessing fields directly via getters and setters, encapsulate the behavior that operates on these fields. This preserves both the integrity of the object and encourages a richer domain model.
Example:
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void rename(String newName) {
if (newName != null && !newName.isEmpty()) {
this.name = newName;
}
}
}
Here, the rename
method encapsulates the logic required to change the name. It ensures that only valid names are set, thereby enforcing business rules.
2. Use Constructor for State Initialization
When creating objects, safeguard against invalid states by initializing required fields in the constructor, rather than relying on setters after instantiation.
Example:
public class Order {
private final List<Item> items;
public Order(List<Item> items) {
this.items = new ArrayList<>(items);
}
}
This ensures that an Order
can only be created with a valid list of items.
3. Utilize Immutable Objects
Making classes immutable can provide thread safety and a level of guarantee that an object’s state won’t change unexpectedly.
Example:
public final class ImmutablePerson {
private final String name;
public ImmutablePerson(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Once an ImmutablePerson
object is created, its state cannot change, which can prevent unintended side effects.
Final Thoughts
While getters and setters have their place in Java programming, their overuse can lead to compromised code quality, a lack of encapsulation, and unnecessary complexity. By focusing on behavior encapsulation, employing constructors to initialize state, or using immutable objects, you can foster a clean, manageable, and robust codebase.
If you are looking to learn more about effective OOP practices, visit Oracle's Java Tutorials for more insights.
Developers should strive for a balance—using getters and setters wisely when needed, but not letting them become the crutch that weighs down your design. Embracing better design patterns will improve not only your code quality but also the development experience for your team. Happy coding!
Checkout our other articles