Maximizing Code Quality: Tackling Low Test Coverage Issues
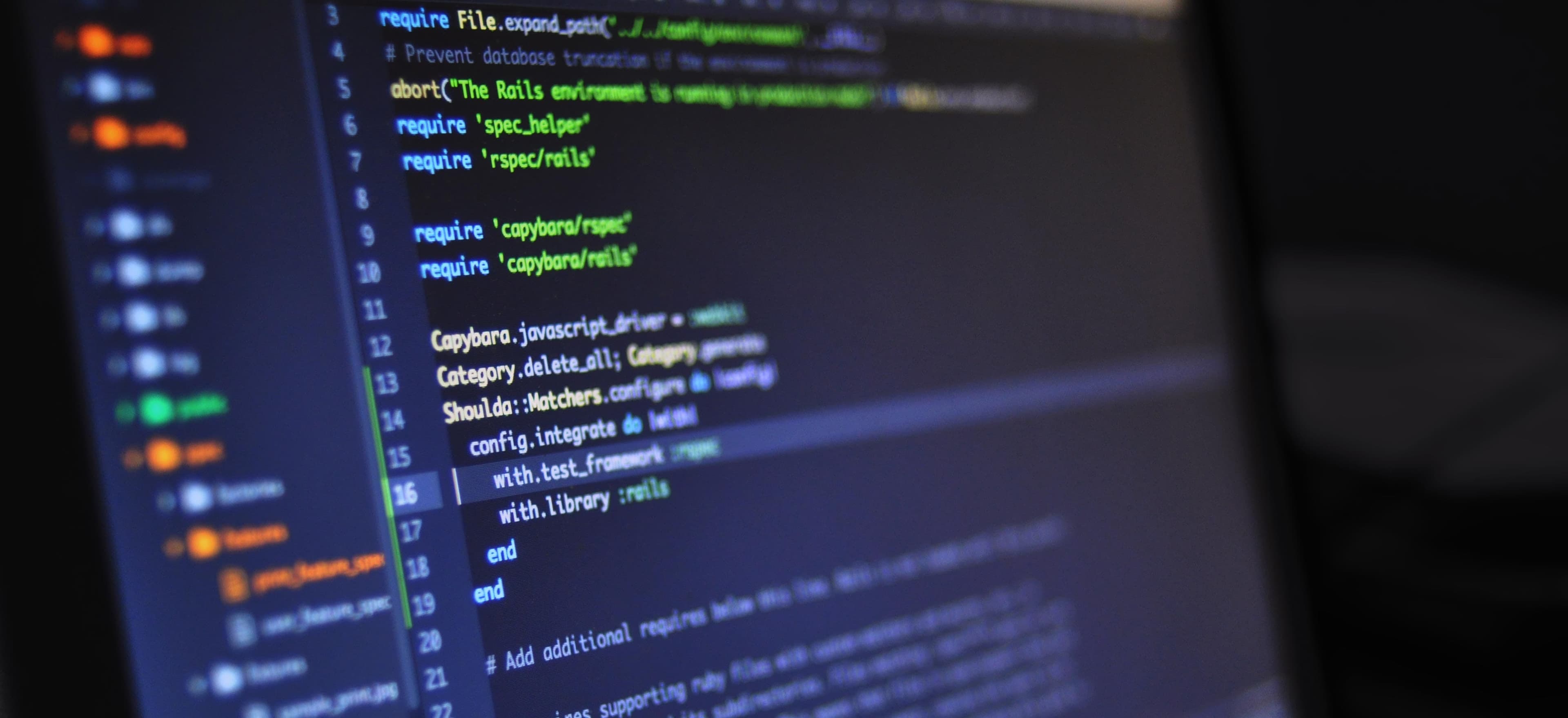
- Published on
Maximizing Code Quality: Tackling Low Test Coverage Issues
In the world of software development, code quality is paramount. One of the significant indicators of this quality is test coverage. High test coverage often correlates with fewer bugs, easier maintenance, and an overall higher-quality product. However, many teams struggle with low test coverage issues. This blog post explores strategies to maximize code quality by addressing low test coverage, providing actionable insights and code snippets to illustrate key concepts.
Understanding Test Coverage
Before we dive into solutions, let's clarify what test coverage means.
Test coverage is a measure used to gauge the amount of code in a program that is tested by automated tests. It typically includes metrics such as:
- Statement Coverage: Percentage of code statements executed during tests.
- Branch Coverage: Percentage of decision points tested.
- Function Coverage: Percentage of functions that have been called during tests.
Having a solid test coverage report helps teams identify parts of the codebase that might become problematic. While 100% coverage isn’t always practical, aiming for at least 70-80% is a commendable standard.
Reasons for Low Test Coverage
Low test coverage can stem from multiple issues:
- Complex Code: Code that is convoluted can make it challenging to write tests.
- Lack of Time: Teams may prioritize feature development over test writing.
- Inadequate Testing Culture: Organizations with a low emphasis on testing often find themselves with insufficient coverage.
- Unfamiliarity with Testing Tools: Lack of knowledge about testing frameworks can hinder effective testing.
Example Analysis of Low Coverage
Consider the following Java code snippet:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int divide(int a, int b) {
return a / b;
}
}
Assuming this code is part of a larger application, if our tests focus only on the add
method, our coverage will be below 50%. Not only does this leave potential bugs in untested areas, but if the divide operation fails, it can lead to runtime issues.
Strategies to Improve Test Coverage
In this section, we will delve into specific strategies to boost test coverage while maintaining code quality.
1. Educate Your Team on Testing Tools
Start with training sessions or workshops on unit testing tools and frameworks. Encourage best practices and introduce concepts such as:
- JUnit: The de facto standard for unit testing in Java.
- Mockito: A mocking framework that can isolate the code you want to test.
JUnit Example
Here is an example of how to write a unit test using JUnit:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
private final Calculator calculator = new Calculator();
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
@Test
public void testDivide() {
assertEquals(2, calculator.divide(6, 3), "6 / 3 should equal 2");
}
}
In this example, we cover both functions in the Calculator
class, raising our test coverage significantly.
2. Emphasize the Importance of Testing in Code Reviews
Make testing a critical part of your code review process. Ensure that new features come with sufficient tests. Here’s how:
- Open each pull request with a checklist that includes “tests created for new features.”
- Incentivize proper test coverage during performance evaluations.
3. Aim for Incremental Improvements
Set achievable goals. For example, if your test coverage is currently at 40%, aim for 60% within a few sprints.
Coverage Tools
Utilize tools like JaCoCo to measure code coverage:
<dependency>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.7</version>
</dependency>
Incorporating a coverage tool helps visualize areas needing more tests, guiding your team's focus effectively.
4. Refactor Complex Code
Complex code often leads to low test coverage. Refactoring can simplify this code and enable better testability. Apply the following principles:
- Single Responsibility Principle: Ensure that each class or method serves a single purpose.
- Separation of Concerns: Split code into different components that each handle a specific piece of functionality.
Refactored Code Example
Here’s how we can refactor the Calculator
class:
public class MathOperations {
public int add(int a, int b) {
return a + b;
}
}
public class DivisionOperations {
public int divide(int a, int b) {
if (b == 0) throw new IllegalArgumentException("Cannot divide by zero");
return a / b;
}
}
Now, we have clearer responsibilities, which not only improves readability but also makes it easier to write tests for each operation.
5. Build Test Cases for Edge Cases
Pay particular attention to edge cases and potential error conditions. For instance, handling division by zero elegantly is crucial:
@Test
public void testDivideByZero() {
Exception exception = assertThrows(IllegalArgumentException.class, () -> {
calculator.divide(1, 0);
});
assertEquals("Cannot divide by zero", exception.getMessage());
}
6. Automate Your Testing Pipeline
Integrate testing into your CI/CD pipeline. Tools like Jenkins and GitHub Actions can help automate the running of tests with every code change. This automation ensures:
- Bugs are caught early.
- Developers are encouraged to implement tests, knowing that they will be run automatically.
7. Monitor and Adjust
Continuously measure your test coverage. Regularly revisit your efforts and adjust based on new features or complexities added to your codebase. Employ dashboards that visualize coverage changes over time.
Closing Remarks
Maximizing code quality through effective testing strategies is essential in today's software development landscape. By recognizing the importance of test coverage and addressing the factors leading to its decline, development teams can create robust applications.
Here’s a quick recap of the strategies discussed:
- Educate your team on effective testing tools and practices.
- Incorporate testing into your code review process.
- Set incremental improvement goals.
- Refactor complex code into simpler components.
- Build test cases for edge conditions.
- Automate the testing process within your CI/CD pipeline.
- Monitor your progress and adjust as needed.
By following these guidelines, you will not only increase test coverage but also build a culture that values high-quality code—leading to fewer bugs, happier customers, and ultimately, a more successful product.
Additional Resources
For further reading, check out these resources for deeper insights into testing in Java:
Maximizing code quality is a journey, not a destination. Start today, and watch your team flourish!
Checkout our other articles