Mastering Legacy Code: The Art of Effective Renaming
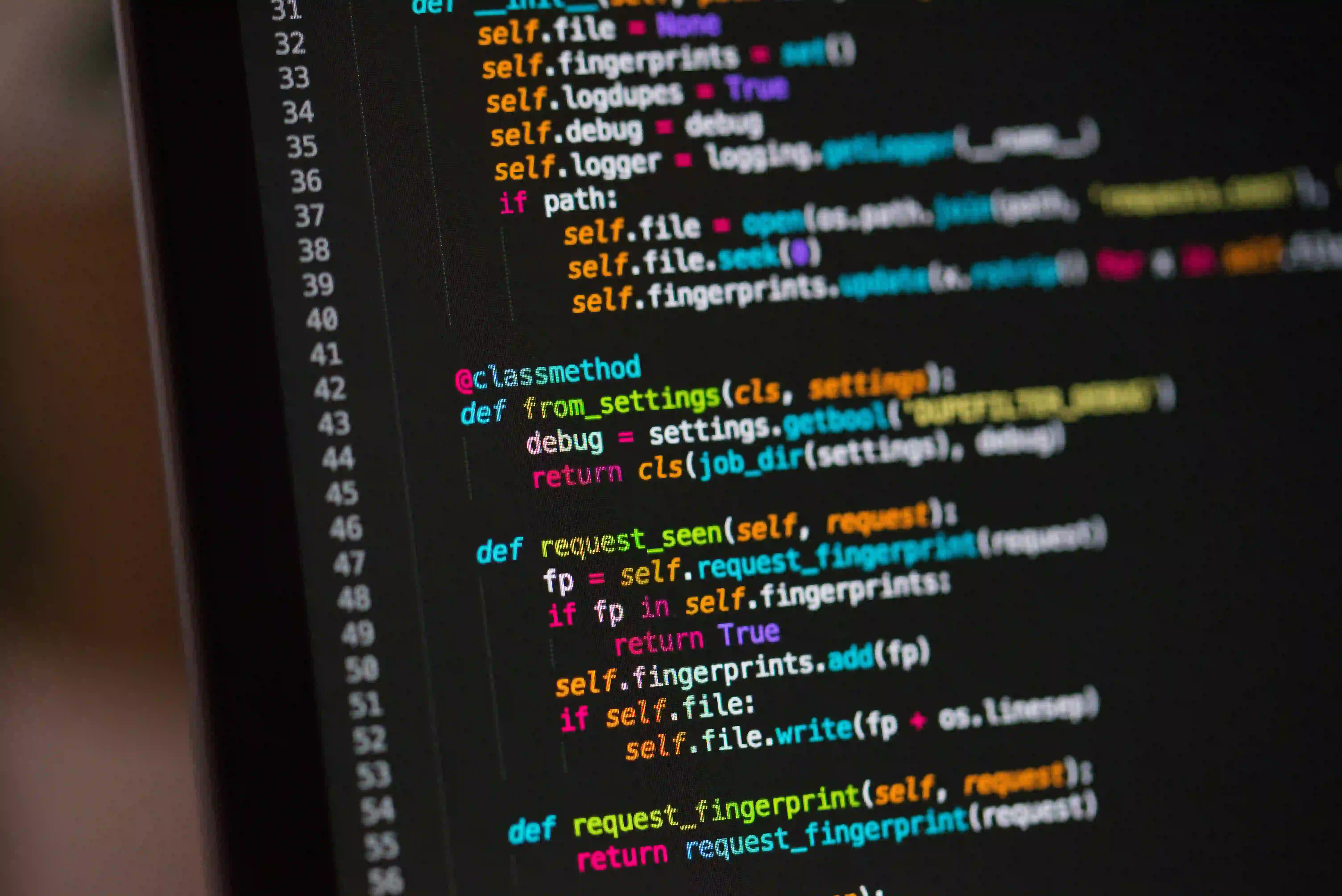
Mastering Legacy Code: The Art of Effective Renaming
Renaming variables, classes, or methods in legacy code can often feel like a daunting task, fraught with potential pitfalls. However, mastering the art of effective renaming can make a significant difference in code readability, maintainability, and overall quality. In this post, we will explore the reasons behind renaming, best practices, and practical techniques for executing renaming safely and effectively.
Understanding Legacy Code
Legacy code, by definition, refers to code that has been inherited from previous developers or teams that may no longer be in use. Often, this code can be outdated, poorly documented, or written in ways that no longer align with current programming practices.
Why Renaming Matters
1. Clarity
Renaming variables, functions, or classes helps provide clarity. Clear names convey their intent, reducing the cognitive load on the next developer who has to work with it. For example:
// Poorly named variable
int a; // What's "a"? It's unclear.
Renaming it to something meaningful could look like this:
int totalItems; // Now it's clear what this variable represents.
2. Consistency
A consistent naming convention across your codebase helps maintain coherence. This becomes particularly essential in large projects where different developers may have varied naming styles. A consistent approach simplifies navigation through the code.
3. Code Maintenance
When code is clearer and more consistent, it is easier to maintain. This reduces the time and effort needed for future code changes, which is essential for the longevity of any software.
Best Practices for Renaming
Renaming in legacy code requires a thoughtful approach. Here are some best practices to keep in mind:
1. Establish Naming Conventions
Decide on a naming convention before you start renaming. Whether it’s camelCase, PascalCase, or snake_case, consistency is key. Always document the chosen conventions for clarity across your team.
2. Use Meaningful Names
Ensure that the names convey the purpose of the variable or function. Avoid vague names like temp
, data
, or value
, as they offer no context to other developers.
3. Batch Renaming with Version Control
Use version control systems like Git to manage your changes. Renaming in small, isolated batches can reduce the risk of introducing bugs.
git checkout -b feature/rename-variable
4. Conduct Code Reviews
After renaming, conducting thorough code reviews helps catch any lingering issues. Peer input can provide new perspectives and ensure that the new names are indeed clearer.
5. Automated Refactoring Tools
Take advantage of integrated development environment (IDE) features and tools for renaming. Tools like IntelliJ IDEA, Eclipse, and Visual Studio provide safe, automated refactoring options. This can help avoid errors when renaming.
Practical Techniques for Renaming
Let’s walk through the renaming process with a practical example.
Initial Assessment
Before renaming, assess your code. Consider a Java class for handling user emails:
public class EmailHandler {
public void sendMail(String mail) {
// Implementation to send mail
}
}
Here, the parameter mail
is unclear. Should it be email
, emailAddress
, or something else? We need a clearer name.
Renaming Process
-
Identify the Context: Understand the broader role of the variable or method.
-
Choose a Meaningful Name: Decide a more explicit name. In this instance, we can change
mail
toemailAddress
. -
Refactor the Code: Use your IDE’s renaming tool to safely rename the variable.
public class EmailHandler {
public void sendMail(String emailAddress) {
// Implementation to send the email
}
}
-
Update All References: Ensure that all references to
mail
are updated across the codebase. -
Run Tests: After making the changes, run your tests to confirm that everything still functions correctly.
Example with Automated Tools
IDEs like IntelliJ IDEA offer renaming functionality that updates all instances automatically:
- Right-click the variable name.
- Select "Refactor" -> "Rename".
- Enter the new name.
This highlights the importance of leveraging technology to improve renaming efficiency while reducing human error.
Ensuring Valid Changes
Lastly, it is essential to ensure that the new names are indeed improvements. This could involve gathering feedback from peers and soliciting opinions from those who use or maintain the code.
In Conclusion, Here is What Matters
Renaming legacy code is not merely about changing names; it's about enhancing the clarity, maintainability, and effectiveness of your codebase. By following best practices and employing the right techniques, developers can significantly improve the quality of legacy code. Remember, the goal of renaming is to create code that is easy to read, understand, and modify – ultimately leading toward a more productive development cycle.
Effective renaming is an ongoing effort in the software development process, contributing to a cleaner codebase that can be easily understood by future developers. For more on best practices in software maintenance, you can check out Martin Fowler’s Refactoring and explore other resources related to legacy code management.
By mastering the art of effective renaming, you take significant strides toward transforming your legacy code into a well-documented, comprehensible work of software engineering art. Happy coding!