Common Pitfalls in Integration Testing of REST APIs with Spring Boot
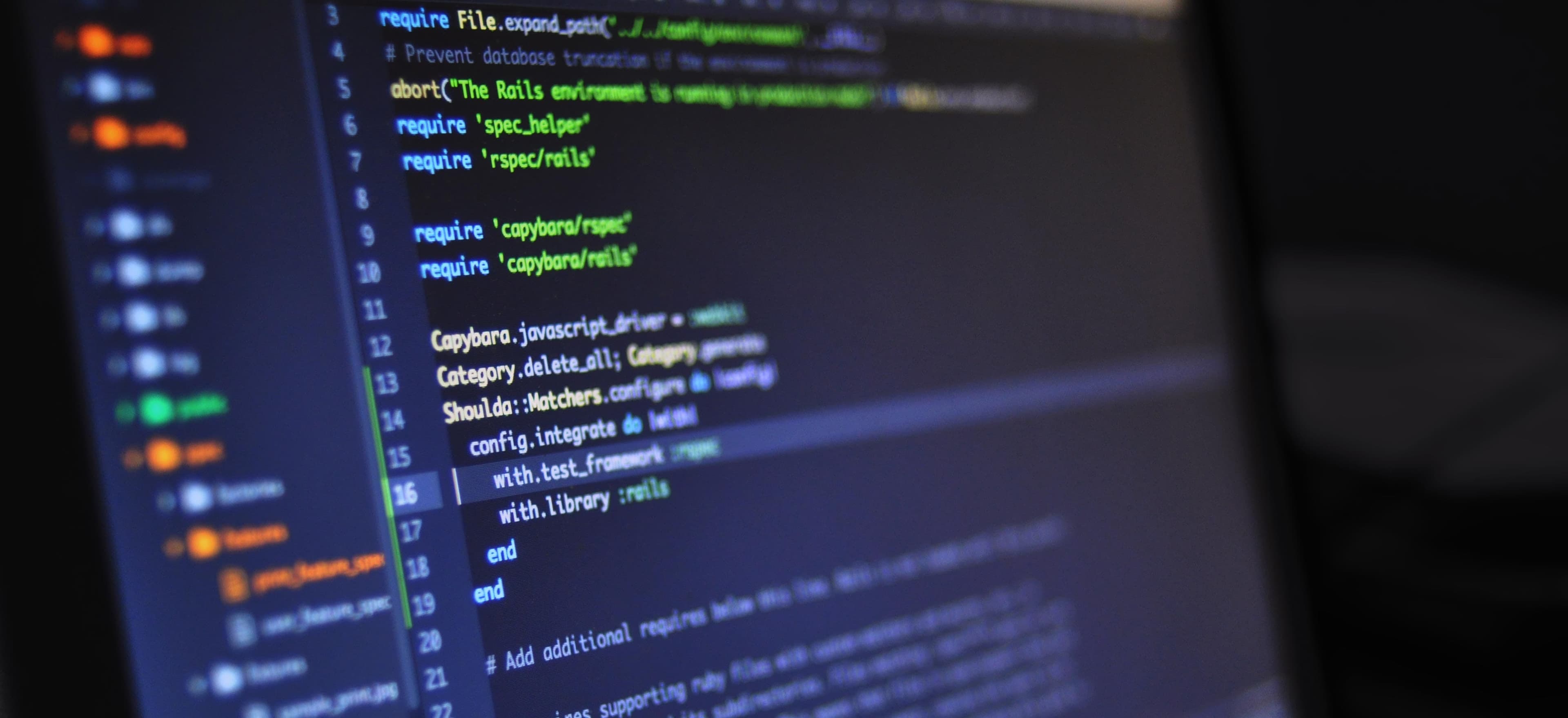
- Published on
Common Pitfalls in Integration Testing of REST APIs with Spring Boot
Integration testing is a critical part of the software development lifecycle, particularly for REST APIs. When you build applications using Spring Boot, it's essential to ensure that different components work together as intended. However, integration testing can often present various challenges. This post highlights some common pitfalls encountered during integration testing of REST APIs with Spring Boot and offers practical solutions to avoid them.
Understanding Integration Testing in Spring Boot
Integration tests validate the behavior of various components in your application, ensuring that they work together seamlessly. In a Spring Boot application, these could include controllers, services, and repositories. Unlike unit tests, which isolate part of the code, integration tests cover larger sections of the application.
Spring Boot provides excellent support for integration testing with its built-in testing libraries. You can use annotations like @SpringBootTest
, @MockBean
, and @WebMvcTest
to configure your tests effectively.
Example: Basic Integration Test
Let's start with a simple integration test for a REST endpoint. Consider the following controller that handles user data:
@RestController
@RequestMapping("/api/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.findUserById(id);
return ResponseEntity.ok(user);
}
}
Integration Test Code Snippet
Here's how an integration test for the above controller might look:
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
public class UserControllerIntegrationTest {
@Autowired
private TestRestTemplate restTemplate;
@MockBean
private UserService userService;
@BeforeEach
void setup() {
User mockUser = new User(1L, "John Doe", "johndoe@example.com");
Mockito.when(userService.findUserById(1L)).thenReturn(mockUser);
}
@Test
void testGetUser() {
ResponseEntity<User> response = restTemplate.getForEntity("/api/users/1", User.class);
assertEquals(HttpStatus.OK, response.getStatusCode());
assertEquals("John Doe", response.getBody().getName());
}
}
In this example, we're using @SpringBootTest
to load the application context and TestRestTemplate
to simulate HTTP requests.
Common Pitfalls in Integration Testing
1. Not Isolating Tests
Summary: Integration tests can unintentionally rely on the state left behind from other tests.
Solution: Ensure that your integration tests run in isolation. Spring's lifecycle annotations such as @DirtiesContext
allow you to reset the application context after a test class or method runs, preventing states from influencing other tests.
2. Database Dependencies
Summary: Integration tests often rely on a real database. This requires managing data and states effectively.
Solution: Use an in-memory database like H2 for testing. You can configure your application to use H2 during tests and real databases in production. Furthermore, employ database rollback methods after test execution to maintain clean states.
# application-test.yml for H2 database configuration
spring:
datasource:
url: jdbc:h2:mem:testdb
driver-class-name: org.h2.Driver
username: sa
password:
platform: h2
3. Inadequate Assertions
Summary: It’s easy to forget that integration tests should validate not just the status code but also the body of the response.
Solution: Perform thorough assertions. Apart from checking HTTP response status, ensure that the returned data matches expected results.
assertEquals("john.doe@example.com", response.getBody().getEmail());
4. Ignoring HTTP Methods
Summary: It’s crucial to test different HTTP methods (GET, POST, PUT, DELETE) and how they impact state.
Solution: Create separate tests for each HTTP method with appropriate scenarios. For instance, a POST test should verify that a new resource is created correctly.
Example: Testing POST Method
@Test
void testCreateUser() {
User newUser = new User(null, "Jane Doe", "janedoe@example.com");
ResponseEntity<User> response = restTemplate.postForEntity("/api/users", newUser, User.class);
assertEquals(HttpStatus.CREATED, response.getStatusCode());
}
5. Forgetting Error Handling Paths
Summary: Comprehensive testing includes exploring how your API handles erroneous requests.
Solution: Ensure to write tests that send invalid data to your API. Assert that the appropriate error messages and HTTP status codes are returned.
@Test
void testGetNonExistentUser() {
ResponseEntity<String> response = restTemplate.getForEntity("/api/users/999", String.class);
assertEquals(HttpStatus.NOT_FOUND, response.getStatusCode());
}
6. Not Using Mocking Appropriately
Summary: Using real services instead of mocks can lead to slow test execution and complexity.
Solution: Use @MockBean
for service-layer dependencies to avoid hitting the database or any external services. This keeps your tests fast and focused, ensuring they only validate the integration of components you're interested in.
Additional Tools for REST API Testing
For additional robustness in testing REST APIs built with Spring Boot, consider using tools like Postman or RestAssured. These tools provide powerful features for testing APIs, from basic requests to complex scenarios.
RestAssured Example
Here's an example of how you can write integration tests using RestAssured.
import static io.restassured.RestAssured.given;
@Test
public void testGetUserWithRestAssured() {
given()
.when()
.get("/api/users/1")
.then()
.statusCode(HttpStatus.OK.value())
.body("name", equalTo("John Doe"));
}
Final Thoughts
Integration testing REST APIs in Spring Boot can present various pitfalls, but by being aware of them, you can write more effective tests. Always ensure that each test is isolated, utilize an in-memory database for state management, perform thorough assertions, and handle error paths. Additionally, using services like Postman and RestAssured can greatly enhance your testing capabilities.
By proactively addressing these common pitfalls, you will develop a more resilient and well-tested API, ensuring its reliability in a production environment. Happy testing!
Checkout our other articles