Overcoming Technical Debt: Agile Strategies That Work
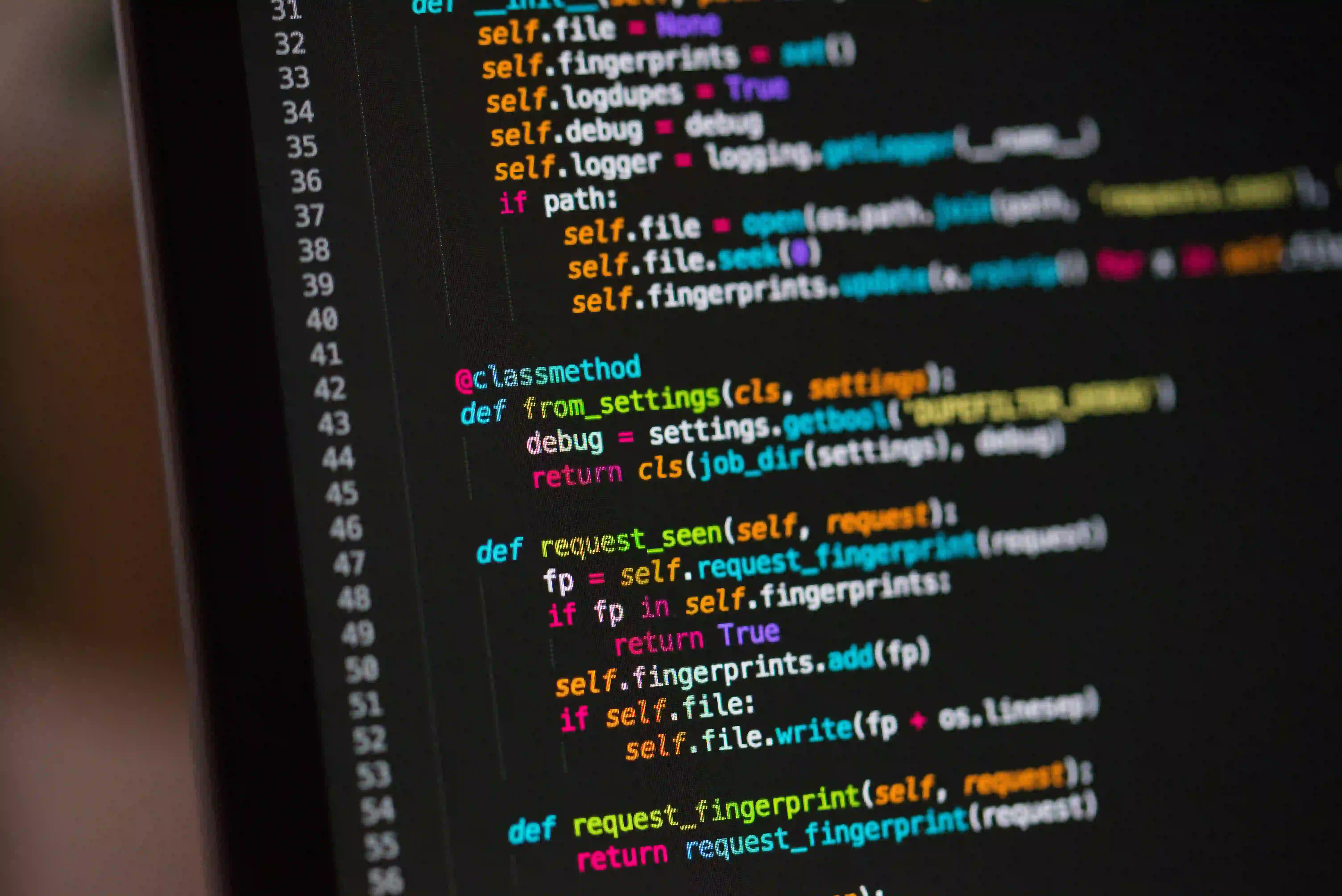
Overcoming Technical Debt: Agile Strategies That Work
In today's fast-paced development environment, technical debt can be a persistent problem. Organizations often trade long-term quality for short-term speed. However, neglecting this debt may lead to escalating costs, performance issues, and a decline in product quality. This blog post explores effective Agile strategies to overcome technical debt, providing you with actionable insights and exemplary code snippets to incorporate in your projects.
Understanding Technical Debt
Before exploring strategies for overcoming technical debt, let’s clarify what it means. Technical debt refers to the shortcuts taken during software development—like choosing an easy solution instead of a better one—resulting in suboptimal code. This concept was initially introduced by Ward Cunningham in 1992, indicating that “if you take a shortcut, you’ll have to pay it back later.”
Why Does Technical Debt Accumulate?
- Rushed Deadlines: Product launches often pressure teams to deliver quickly.
- Lack of Documentation: Failing to document decisions can lead to misunderstandings.
- Changing Requirements: Agile methodologies introduce iterative development; changes can cause residual impacts on the codebase.
Addressing these issues requires a systematic approach—in short, strategies borrowed from Agile methodologies.
Strategies to Overcome Technical Debt
Although technical debt might feel overwhelming, several Agile practices can help teams manage and reduce it effectively.
1. Prioritize Technical Debt in Your Backlog
Agile emphasizes the importance of prioritizing work in a backlog, and technical debt should not be an exception.
Consider adding technical debt items alongside feature requests and bugs. Use clear definitions and prioritize these tasks based on their impact.
// Sample prioritization strategy
public void prioritizeBacklog(List<Task> backlog) {
Collections.sort(backlog, Comparator.comparing(Task::getPriority)
.thenComparing(Task::getType));
}
Commentary:
This simple Java method sorts tasks based on their priority and type. By applying this strategy, teams can ensure that technical debt does not accumulate unnoticed.
2. Implement Refactoring Sessions
Regular refactoring sessions are essential in addressing technical debt. Agile encourages continuous improvement, and dedicating time explicitly for refactoring can lead to a cleaner codebase.
// Sample refactoring code snippet
public void calculateTax(List<Item> items) {
double tax = items.stream()
.mapToDouble(Item::getPrice)
.sum() * TAX_RATE;
applyTax(tax);
}
Commentary:
In this snippet, we're leveraging Java Streams to simplify our tax calculation. Refactoring code not only improves readability but also makes it easier for developers to maintain and understand.
3. Adopt a Definition of Done
A clear definition of done (DoD) helps ensure that all aspects of the task, including maintenance and code quality, are completed. Include criteria related to technical debt in your DoD.
// Example definition of done
public boolean isDone(Task task) {
return task.isCodeComplete() && task.isTested() && task.isReviewed() && !task.hasTechnicalDebt();
}
Commentary:
This method assesses whether a task meets all criteria. By integrating a check for technical debt in your DoD, teams ensure that new code does not introduce additional debt.
4. Foster a Culture of Continuous Learning
Agile emphasizes collaboration and knowledge sharing. Encourage your team to learn about best practices and new technologies to avoid accumulating technical debt. Invest in regular training sessions or workshops.
- Use resources like Coursera or Udemy to find relevant courses.
5. Schedule Regular Technical Debt Remediation Sprints
Creating dedicated sprints focusing purely on technical debt can help alleviate accumulation.
Consider allocating a percentage of each sprint for addressing technical debt, refining practices that led to accumulation in the first place.
### Sample Agile Sprint Structure
- **80% Feature Development**
- **20% Technical Debt Management**
During each sprint, teams can identify and rectify outstanding debt using collective knowledge.
6. Utilize Metrics to Monitor Technical Debt
Incorporating metrics can help visualize the impact of technical debt over time. Consider using tools like SonarQube or CodeClimate to analyze code quality. Metrics can involve:
- Code coverage
- Code complexity
- Code smells
Regularly review these metrics to make informed decisions regarding technical debt.
// Example of monitoring call stacks for complexity
import static org.apache.commons.lang3.Validate.*;
public void monitorComplexity(Method method) {
int complexity = calculateComplexity(method);
ensure(complexity < MAX_COMPLEXITY, "High complexity detected!");
}
Commentary:
Here, we incorporate complexity checks on a method. Monitoring complexity constantly encourages developers to keep their code simple and manageable.
7. Review and Communicate Changes
Maintaining open communication within the team is crucial. Regular reviews of the technical debt portfolio can help raise awareness. Implement regular stand-up meetings that allow developers to highlight areas of concern.
- Tools like Jira can aid in tracking and communicating technical debt.
8. Leverage Pair Programming
Pair programming encourages collaboration and knowledge-sharing between developers, which can lead to higher-quality code and reduced technical debt.
In pair programming, two developers work together at one workstation, often leading to immediate feedback and better coding practices.
// Example of utilizing pair programming
public void processOrders(List<Order> orders) {
for (Order order : orders) {
processOrder(order);
}
}
Commentary:
This code illustrates a straightforward order processing system that can be enhanced through pair programming. Working together enables developers to critique and improve each other's code in real time.
Closing the Chapter
Technical debt is a challenge in modern software development, particularly within Agile methodologies. By incorporating prioritized practices, defining clear criterions, and fostering a culture of continuous learning, teams can effectively manage and reduce technical debt.
Adoption of Agile strategies doesn’t just enhance the immediate project but sets a solid foundation for long-term development success. Remember, addressing technical debt today reduces complications and costs tomorrow.
For a deeper dive into Agile methodologies, visit Agile Alliance or consider enrolling in Agile-centric courses on platforms like Pluralsight. By enhancing your team's Agile practices, you’ll build a more robust, efficient, and high-quality software solution.
Feel free to explore these strategies and adapt them to your development process. Your future code will thank you!