Understanding Optional.orElse vs. Optional.orElseGet: A Pitfall
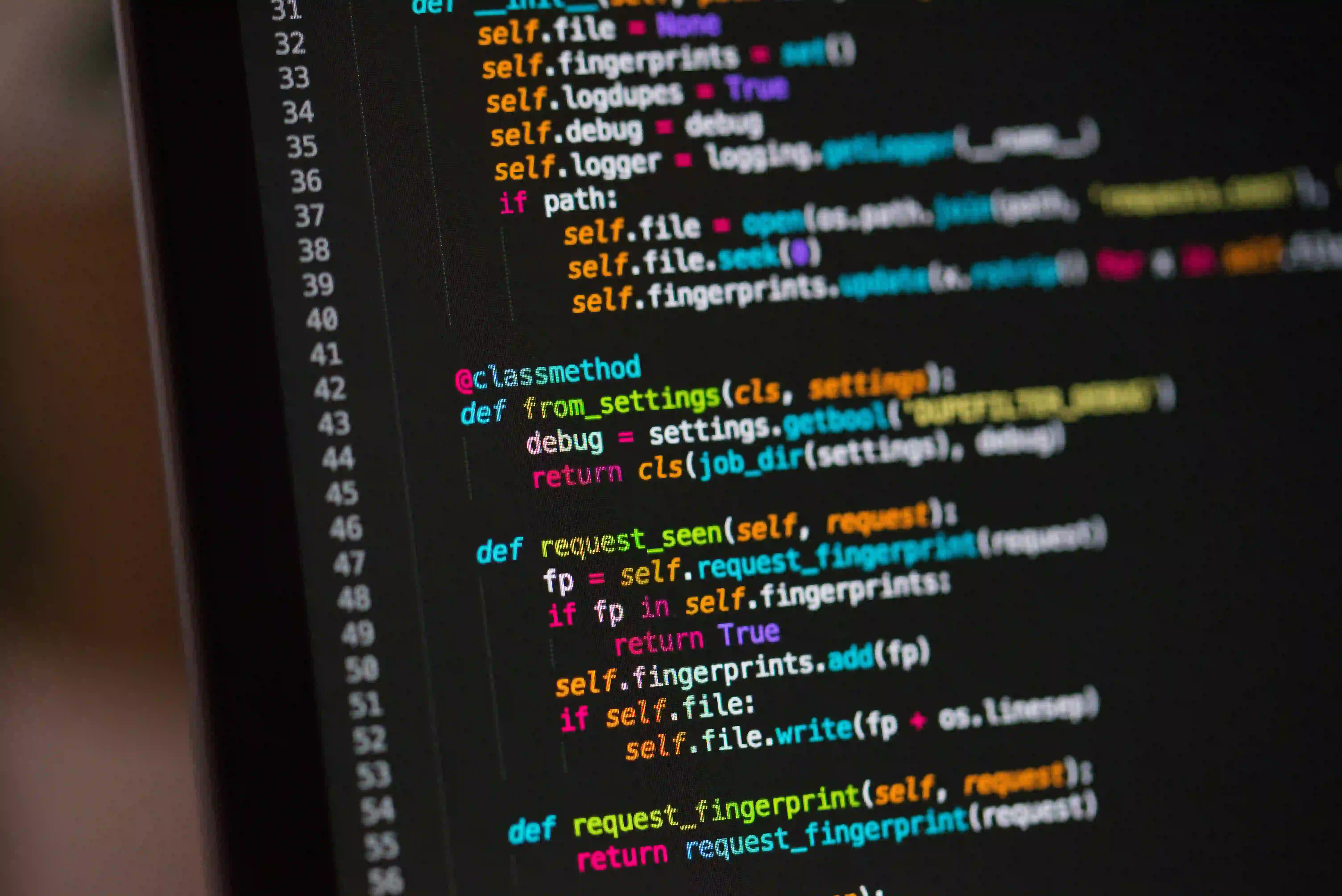
Understanding Optional.orElse vs. Optional.orElseGet: A Pitfall
In the world of Java programming, one of the challenges developers often face is null reference management. Enter Optional
, a container object introduced in Java 8 to address this very issue. One feature of Optional
that can sometimes cause confusion is the use of orElse
and orElseGet
. In this post, we'll dive into the differences between these two methods and highlight why making the right choice can impact both performance and clarity in your code.
What is Optional?
Before we jump into orElse
and orElseGet
, it's essential to understand what Optional
is designed for.
Optional
is a Java class that acts as a container for objects that might be absent. Its primary purpose is to reduce the incidence of NullPointerException
and to provide a clear intent that a value may or may not be present.
Optional<String> optionalName = Optional.ofNullable(getName());
In this example, getName()
could return a String
or it could return null
. By using Optional
, we make our intent explicit.
The Methods: orElse and orElseGet
Optional.orElse()
The orElse
method provides a fallback value when the Optional
is empty:
String name = optionalName.orElse("Default Name");
In the above code, if optionalName
does not contain a value, "Default Name" is returned.
Optional.orElseGet()
On the other hand, orElseGet
takes a Supplier
functional interface that generates the fallback value, only executing the provided logic when the Optional
is empty:
String name = optionalName.orElseGet(() -> generateDefaultName());
Here, generateDefaultName()
is called only if optionalName
is empty.
The Key Distinction
The crucial difference between orElse
and orElseGet
lies in when the fallback value is computed:
orElse
computes the fallback value immediately, regardless of whether it’s needed.orElseGet
computes the fallback value only when necessary.
Code Example Explained
Imagine you have a method that performs a time-consuming computation to generate a default name:
public String generateDefaultName() {
// Simulate delay
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Generated Name";
}
Now, consider the differences in performance:
public String getNameUsingOrElse() {
Optional<String> optionalName = Optional.ofNullable(getName());
// This will call generateDefaultName even if optionalName has a value
return optionalName.orElse(generateDefaultName());
}
In the above case, generateDefaultName()
is invoked unconditionally, which is inefficient if optionalName
already contains a value.
Conversely, with orElseGet
:
public String getNameUsingOrElseGet() {
Optional<String> optionalName = Optional.ofNullable(getName());
// generateDefaultName is called only if optionalName is empty
return optionalName.orElseGet(this::generateDefaultName);
}
Here, generateDefaultName()
is called only if optionalName
is empty, optimizing performance.
Performance Implications
Choosing orElse
over orElseGet
may not seem like a significant decision, but it can lead to performance pitfalls in specific scenarios, particularly when dealing with expensive operations.
When using orElse
, you might be executing a complex or resource-intensive operation even when it may not be necessary. Conversely, orElseGet
ensures that such logic is only executed if it's truly required, which can result in substantial performance savings in high-load applications.
Practical Guidelines for Implementation
-
Use
orElse
for Simple Values: If your fallback value is a constant or a simple non-complex value that is inexpensive to evaluate, you can safely useorElse
.☕snippet.javaString username = optionalUsername.orElse("guest"); // Simple and straightforward
-
Use
orElseGet
for Complex Logic: Whenever your fallback involves a method call or complex evaluation, preferorElseGet
.☕snippet.javaString username = optionalUsername.orElseGet(this::fetchDefaultUsername); // Efficient evaluation
-
Readability Matters: While performance is essential, always lean toward the choice that makes your code more readable and understandable. Sometimes, clarity trumps minor performance gains.
Closing Remarks
Understanding the difference between Optional.orElse
and Optional.orElseGet
not only contributes to cleaner and more robust Java code but also enhances performance, especially in cases involving time-consuming fallback logic.
In the world of software development, making informed decisions about such small nuances can lead to more maintainable and performant applications. Always aspire to write code that is not just functional, but also efficient and clear.
If you're interested in more best practices surrounding Optional
, consider exploring the Java documentation for additional details, examples, and deeper insights.
Happy coding!