Overcoming Challenges in Tasklet Step Execution in Spring Batch
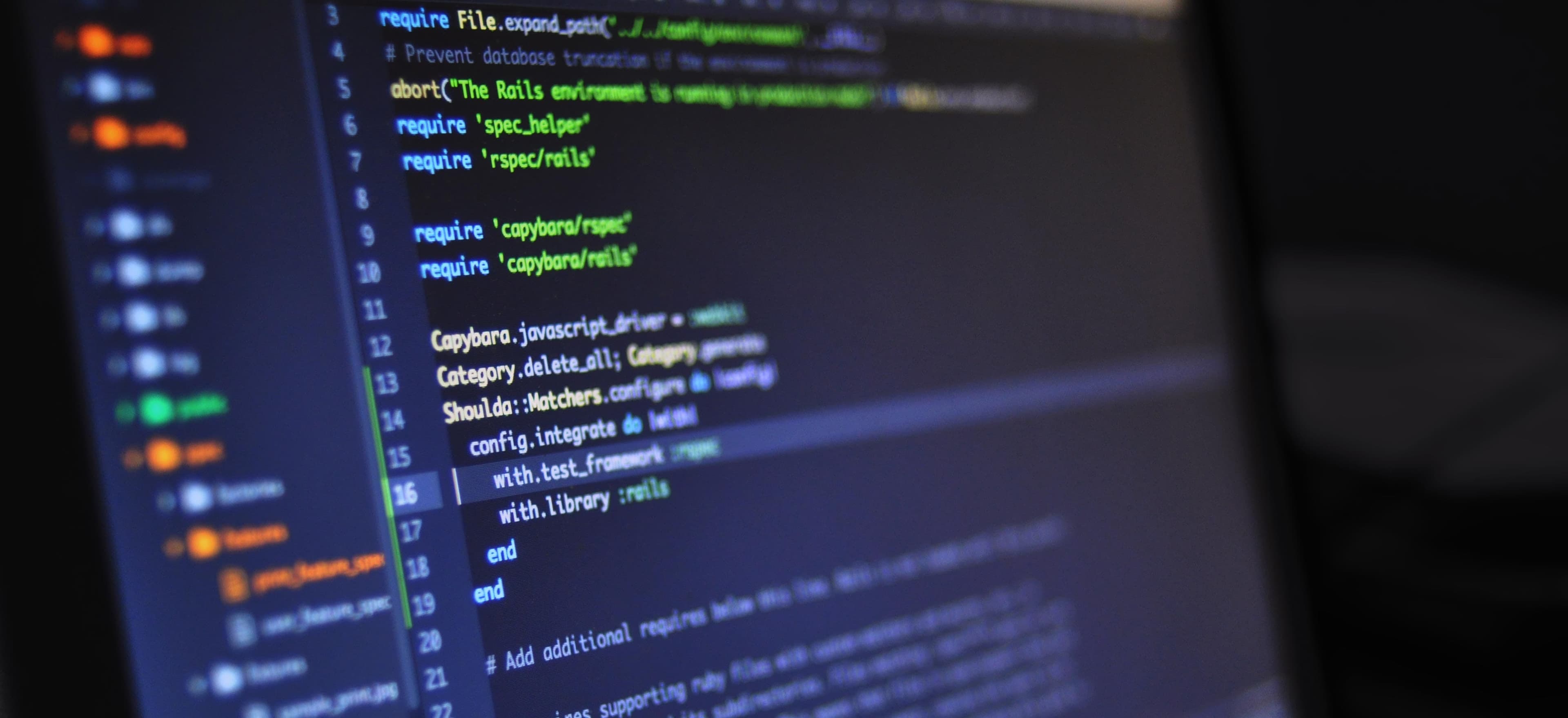
- Published on
Overcoming Challenges in Tasklet Step Execution in Spring Batch
Spring Batch is a powerful framework designed to facilitate batch processing in Java applications. With the growth of data processing needs in economically diverse areas like finance, health, and e-commerce, understanding how to utilize Spring Batch efficiently becomes imperative. In this blog post, we'll focus on the Tasklet
step execution and address common challenges developers face, along with ways to overcome them.
What is a Tasklet in Spring Batch?
A Tasklet
in Spring Batch represents a single step of work to be executed. It is typically used for executing custom business logic rather than chunk-oriented processing, which processes data in chunks.
public class MyTasklet implements Tasklet {
@Override
public RepeatStatus execute(StepContribution contribution, ChunkContext chunkContext) throws Exception {
System.out.println("Executing Tasklet!");
// Your business logic goes here
return RepeatStatus.FINISHED;
}
}
This simple example demonstrates a class implementing the Tasklet
interface. The execute
method is where the main logic happens, and the method returns a RepeatStatus
indicating whether to proceed to the next step or not.
Common Challenges in Tasklet Step Execution
- Handling Exceptions Gracefully
- Managing Transactional Integrity
- Complex Business Logic Implementation
- Monitoring and Logging
- Performance Optimization
Let's delve deeper into each challenge and discuss possible solutions.
1. Handling Exceptions Gracefully
Exception handling is crucial in any application but becomes even more vital in batch processing. If a Tasklet
throws an unchecked exception, the whole job may fail.
Solution: Use RetryTemplate
for Recoverable Exceptions
Using the RetryTemplate
allows you to define retry policies and handle exceptions without failing the job entirely. You can set it up in your configuration:
@Bean
public RetryTemplate retryTemplate() {
RetryTemplate retryTemplate = new RetryTemplate();
FixedBackOffPolicy backOffPolicy = new FixedBackOffPolicy();
backOffPolicy.setBackOffPeriod(2000);
retryTemplate.setBackOffPolicy(backOffPolicy);
retryTemplate.setRetryPolicy(new SimpleRetryPolicy(3));
return retryTemplate;
}
In your Tasklet
, you can use this RetryTemplate
to retry the operation automatically:
public class MyTasklet implements Tasklet {
@Autowired
private RetryTemplate retryTemplate;
@Override
public RepeatStatus execute(StepContribution contribution, ChunkContext chunkContext) throws Exception {
retryTemplate.execute(retryContext -> {
// Task logic here
if (someConditionFails()) {
throw new SomeRecoverableException();
}
return null;
});
return RepeatStatus.FINISHED;
}
}
2. Managing Transactional Integrity
When dealing with a Tasklet
, it is often essential to ensure that the logic executed is transactionally safe. If part of your task fails, you may want to roll back any partial changes to avoid data inconsistency.
Solution: Leverage Spring's Transaction Management
To manage transactions, you can annotate your Tasklet
with @Transactional
so that Spring handles transaction boundaries accordingly.
@Transactional
public class MyTransactionalTasklet implements Tasklet {
@Override
public RepeatStatus execute(StepContribution contribution, ChunkContext chunkContext) throws Exception {
// Business logic that requires transactional management
return RepeatStatus.FINISHED;
}
}
This way, if an exception is thrown within the execute
method, any changes made to the database will be rolled back automatically.
3. Complex Business Logic Implementation
As business requirements become more complex, handling logic within a single Tasklet
can become cumbersome.
Solution: Break Logic into Separate Components
It is best practice to break your logic into manageable and reusable components. Using Spring's dependency injection, you can manage code complexity effectively.
@Component
public class BusinessLogicService {
public void complexLogic() {
// Implement complex business logic here
}
}
Then, inject this service into your Tasklet
:
public class MyTasklet implements Tasklet {
@Autowired
private BusinessLogicService businessLogicService;
@Override
public RepeatStatus execute(StepContribution contribution, ChunkContext chunkContext) throws Exception {
businessLogicService.complexLogic();
return RepeatStatus.FINISHED;
}
}
4. Monitoring and Logging
It is critical to monitor your batch jobs for performance and errors. However, logging too much information can also clog your logging infrastructure.
Solution: Spring Batch Listeners
Utilize listeners to capture job and step execution events efficiently without cluttering your tasklet code.
public class JobCompletionNotificationListener extends JobExecutionListenerSupport {
private static final Logger log = LoggerFactory.getLogger(JobCompletionNotificationListener.class);
@Override
public void afterJob(JobExecution jobExecution) {
if (jobExecution.getStatus() == BatchStatus.COMPLETED) {
log.info("Job completed successfully!");
} else {
log.error("Job failed with status: {}", jobExecution.getStatus());
}
}
}
Register the listener in your job configuration:
@Bean
public Job myJob(JobBuilderFactory jobBuilderFactory, Step myStep) {
return jobBuilderFactory.get("myJob")
.incrementer(new RunIdIncrementer())
.listener(new JobCompletionNotificationListener())
.flow(myStep)
.end()
.build();
}
5. Performance Optimization
Batch jobs can handle large amounts of data, making performance a primary concern.
Solution: Use Chunk Processing Where Suitable
If your use case allows, consider using chunk processing instead of a Tasklet
. Chunk-oriented processing fetches multiple items at once, reduces the number of transactions, and leads to improved performance.
@Bean
public Step chunkStep(StepBuilderFactory stepBuilderFactory, ItemReader<MyItem> reader,
ItemProcessor<MyItem, MyProcessedItem> processor,
ItemWriter<MyProcessedItem> writer) {
return stepBuilderFactory.get("chunkStep")
.<MyItem, MyProcessedItem>chunk(10)
.reader(reader)
.processor(processor)
.writer(writer)
.build();
}
My Closing Thoughts on the Matter
Using Spring Batch with Tasklet
steps can unlock powerful capabilities for your batch processing needs. However, understanding your challenges—like exception handling, transactional integrity, complex logic, monitoring, and performance—is vital for a successful implementation.
By applying the solutions discussed, you can overcome these challenges and create robust batch jobs. For more information, consult Spring's official documentation here.
Be sure to explore these concepts and experiment with them in your own applications. Mastery of Spring Batch can greatly enhance your ability to handle large-scale data processing efficiently.