Common Mockito Pitfalls: Avoiding Mocking Mistakes
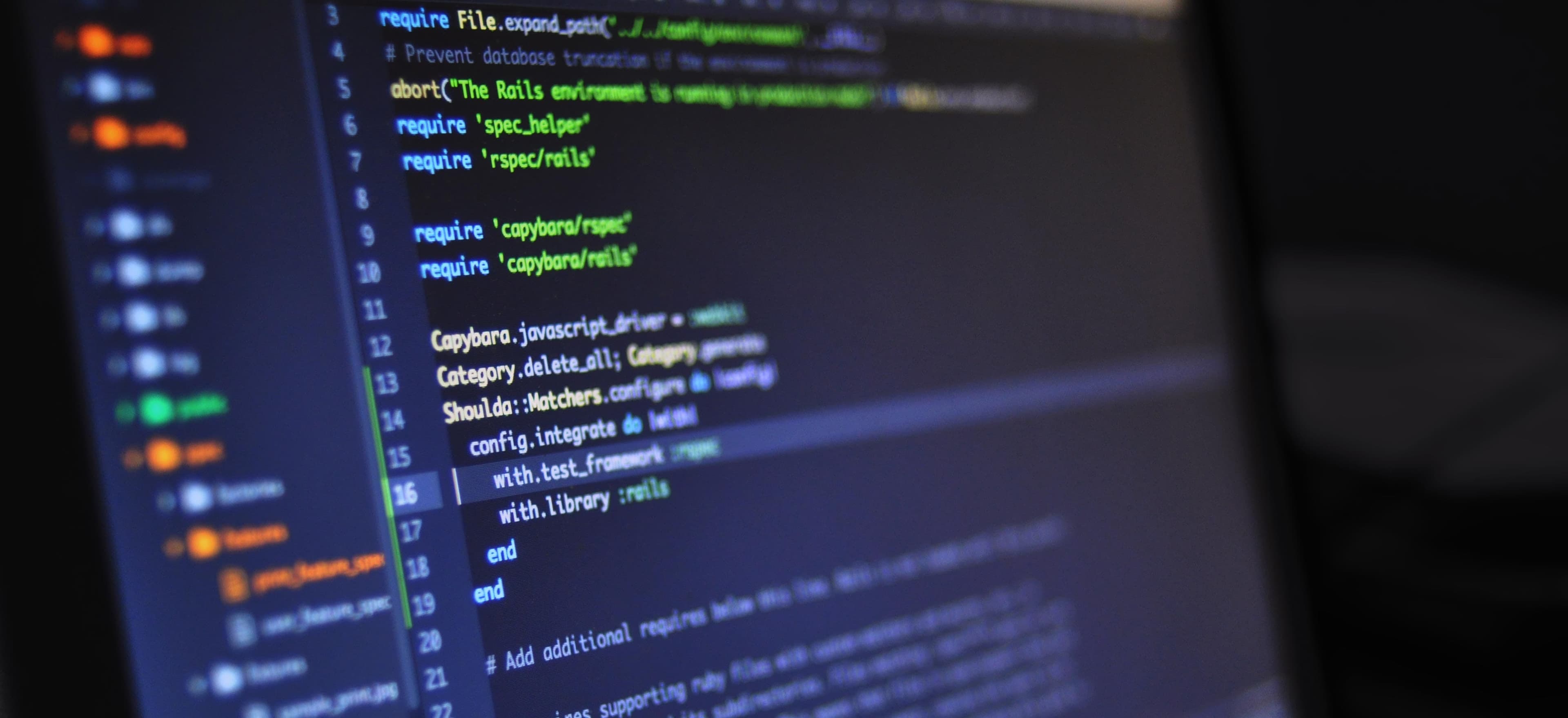
- Published on
Common Mockito Pitfalls: Avoiding Mocking Mistakes
Mockito is a powerful framework for Java developers that allows for the creation of mock objects for unit testing. However, it comes with its own set of pitfalls that can lead to misleading results or hard-to-maintain tests. In this blog post, we will explore some common Mockito pitfalls and provide practical solutions to avoid them.
Table of Contents
Understanding Mocking
Mocking is a way to simulate the behavior of complex objects in your code. By using mock objects, developers can separate unit tests from dependencies, making it easier to test small parts of their codebase. Mockito enables you to create mocks, stubs, and spies seamlessly.
import static org.mockito.Mockito.*;
// Create a mock object of a List
List<String> mockedList = mock(List.class);
// Using the mock
mockedList.add("one");
mockedList.add("two");
// Verify the behavior
verify(mockedList).add("one");
verify(mockedList).add("two");
In the above example, we simulate a List
object to verify its behavior without needing an actual list. This is the power of mocking: focusing only on the functionality we want to test.
Common Pitfalls
1. Over-Mocking
One of the most common pitfalls is over-mocking, where developers create too many mocks that complicate tests.
@Test
public void testOverMocking() {
// Over-mocking with unnecessary complexity
MyService service = mock(MyService.class);
AnotherService anotherService = mock(AnotherService.class);
// This can lead to complex test setup
when(service.process()).thenReturn("result");
when(anotherService.getInfo()).thenReturn("info");
// Actual test logic
}
Solution:
Use mocks only when necessary. If a method does not contain any external calls, there’s no need to mock it. Strive for simplicity in your tests.
2. Lack of Verification
Creating mocks without verifying their interactions can lead to false positives.
@Test
public void testWithoutVerification() {
MyService mockService = mock(MyService.class);
// The execution of the method without verification
mockService.process();
// No verification here means we can't trust this test
}
Solution:
Always verify interactions to ensure your mocks are functioning as needed.
verify(mockService).process();
3. Using Static Methods
Mockito does not directly support mocking static methods. Attempting to do so can lead to unexpected behaviors.
@Test
public void testStaticMethod() {
// This will not work as expected
SomeUtilityClass mockUtility = mock(SomeUtilityClass.class);
when(mockUtility.staticMethod()).thenReturn("mocked result");
}
Solution:
For static methods, consider using PowerMockito or refactor your code to avoid statically dependent methods.
// Bad example of static method
String result = SomeUtilityClass.staticMethod();
Instead, extract static calls into instance methods.
4. Not Resetting Mocks
Failing to reset mocks can lead to residual state that might affect subsequent tests.
@Test
public void testNotResetting() {
MyService mockService = mock(MyService.class);
// First test case
mockService.process();
verify(mockService).process();
// Second test case will fail due to retained state
}
@Test
public void anotherTestWithoutReset() {
verify(mockService).process(); // might fail unexpectedly
}
Solution:
Use reset()
to clear mocks between tests:
reset(mockService);
However, a better approach is to avoid shared mutable state entirely by creating mocks within each test method.
5. Ignoring Exception Handling
Neglecting exception handling can cause tests to appear successful when they're not.
when(mockService.process()).thenThrow(new RuntimeException("Failed"));
try {
mockService.process();
} catch (RuntimeException e) {
// Not verifying the throw can lead to misleading test outcomes
}
Solution:
Be explicit in your tests about expected exceptions.
@Test(expected = RuntimeException.class)
public void testExceptionHandling() {
when(mockService.process()).thenThrow(new RuntimeException("Failed"));
mockService.process(); // this should throw the exception
}
Best Practices in Mockito
- Keep mocks simple: Only mock what you absolutely need.
- Use meaningful names: Use clear names for mocks and verifications to enhance readability.
- Keep a single purpose: Each test should focus on one aspect of behavior.
- Limit shared state: Avoid sharing mocks across tests to minimize side effects.
- Phase out legacy code: Whenever possible, strive for non-static utility classes to simplify your tests.
Final Thoughts
Mockito can dramatically improve the quality of your Java unit testing when used correctly, but avoiding common pitfalls is essential for maintaining clarity and effectiveness in your tests. By following the solutions and best practices outlined in this post, you’ll not only improve your testing skills but also streamline your development workflow.
For more information on Mockito, check out the official documentation. Happy testing!
Checkout our other articles