Overcoming Challenges with Transparent Patch Support in JAX-RS 2.0
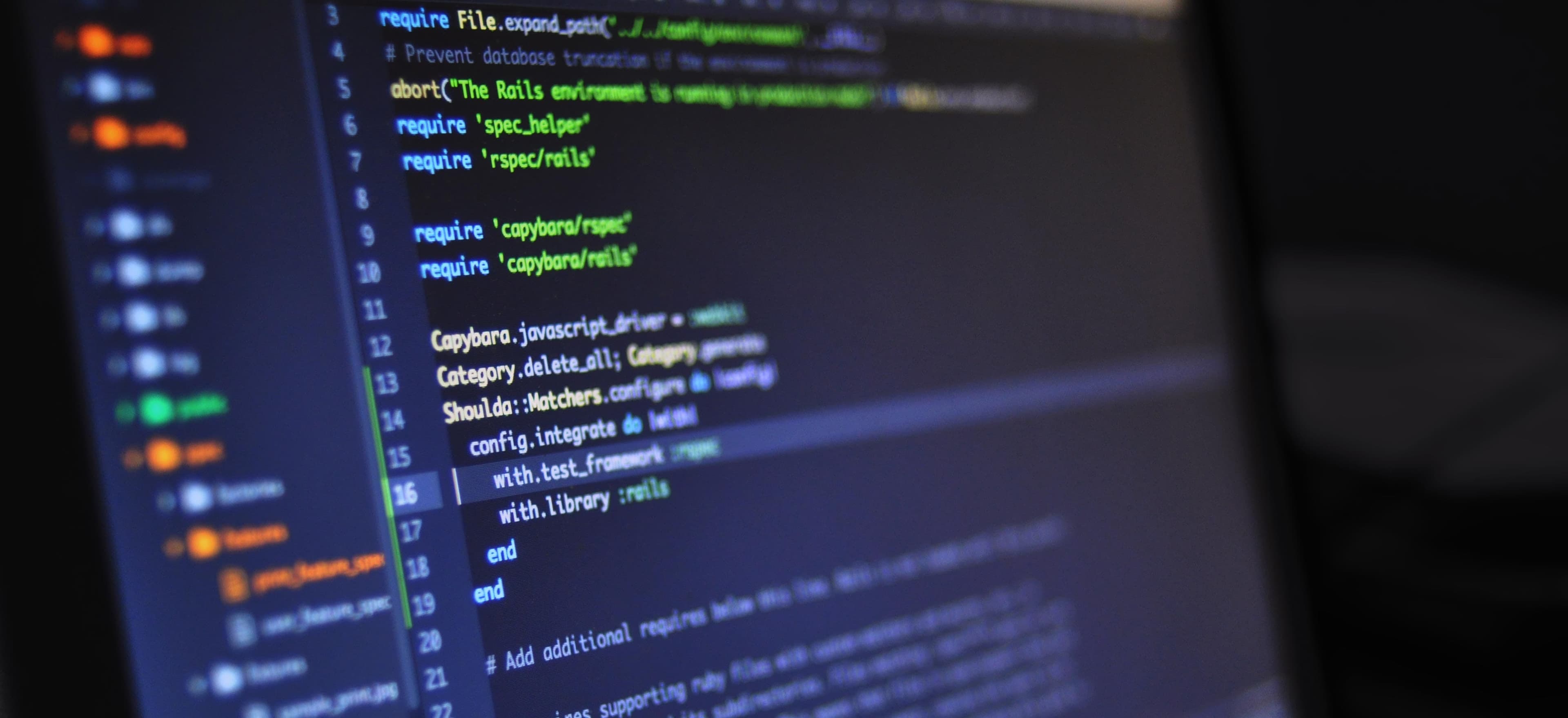
- Published on
Overcoming Challenges with Transparent Patch Support in JAX-RS 2.0
Getting Started
JAX-RS (Java API for RESTful Web Services) 2.0 allows developers to build robust APIs quickly and efficiently. An important aspect of creating a quality RESTful service lies in implementing various HTTP methods such as GET, POST, PUT, and PATCH. One of the more challenging methods to implement is PATCH, especially when aiming for transparency in partial resource updates. In this post, we will dive into the concept of Transparent Patch Support in JAX-RS 2.0, explore its challenges, and provide practical solutions along with code snippets that illustrate how to tackle these issues.
What is Transparent Patch Support?
Transparent Patch Support refers to the ability of a RESTful API to handle partial updates gracefully. This means that when a client sends a PATCH request, only the specified changes to the resource must be applied without altering other existing properties. The goal is to create an API that responds to changes intelligently, minimizing overhead while maintaining data integrity.
The Challenges of Implementing PATCH
1. Handling JSON Patches
One of the most common formats for PATCH requests is JSON Patch, which utilizes a standard format defined by RFC 6902. This standard allows clients to specify an array of operations to apply to a resource. However, implementing support for this standard can be complex, as it involves modifying existing resource representations rather than creating entirely new ones.
2. Merging Nested Objects
APIs can often represent complex data structures with nested objects. When updating a nested object, determining the correct properties to merge can be tricky. An incorrectly implemented merge can lead to losing existing data or failing to apply updates.
3. Validation of Updates
Ensuring data validity after a PATCH request can be particularly challenging. It requires a clear validation mechanism to check if the incoming modifications are valid in the context of the existing resource.
Implementing Transparent PATCH Support in JAX-RS 2.0
Step 1: Basic Setup
First, ensure you have JAX-RS 2.0 configured in your project. You can use popular frameworks like Jersey or RESTEasy. For this example, we will implement a simple PATCH operation using Jersey.
Add the required dependencies to your pom.xml
:
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.35</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
<version>2.35</version>
</dependency>
Ensure you set up your resource class correctly:
@Path("/users")
public class UserResource {
private Map<String, User> userDB = new HashMap<>();
// Initialize some users in the database
@POST
@Consumes(MediaType.APPLICATION_JSON)
public Response createUser(User user) {
userDB.put(user.getId(), user);
return Response.status(Response.Status.CREATED).build();
}
}
Step 2: Creating the PATCH Method
Now, let's add a method to handle PATCH requests. The method will use incoming JSON to update existing user attributes:
@PATCH
@Path("/{id}")
@Consumes(MediaType.APPLICATION_JSON)
public Response updateUser(@PathParam("id") String id, JsonPatch patch) {
User existingUser = userDB.get(id);
if (existingUser == null) {
return Response.status(Response.Status.NOT_FOUND).build();
}
// Apply the patch to the existing user
User updatedUser;
try {
updatedUser = applyPatch(patch, existingUser);
} catch (Exception e) {
return Response.status(Response.Status.BAD_REQUEST).entity(e.getMessage()).build();
}
userDB.put(id, updatedUser);
return Response.ok(updatedUser).build();
}
// Method to apply the JSON Patch
private User applyPatch(JsonPatch patch, User targetUser) {
ObjectMapper objectMapper = new ObjectMapper();
JsonNode patched = patch.apply(objectMapper.convertValue(targetUser, JsonNode.class));
return objectMapper.treeToValue(patched, User.class);
}
Why this implementation?
- We first check if the user exists, which is crucial for data integrity.
- The
applyPatch
method processes the JAX-RSJsonPatch
object. This method converts our existing user into aJsonNode
tree that can be manipulated by the patch, allowing a clean application of the specified changes. - Error handling is key. If the patch fails, we must return a meaningful error message to the client.
Step 3: Testing the PATCH Implementation
Once our PATCH implementation is set up, we can test it using tools like Postman or cURL. Below is an example of a JSON patch request:
[
{"op": "replace", "path": "/name", "value": "John Doe"},
{"op": "remove", "path": "/age"}
]
This request will replace the user’s name and remove their age from the resource representation.
Step 4: Implementing Merging of Nested Objects
In many applications, users have nested structures. Consider the following User class:
public class User {
private String id;
private String name;
private Address address;
// Constructors, getters, and setters
}
public class Address {
private String street;
private String city;
// Constructors, getters, and setters
}
We can enhance our applyPatch
method to deeply merge nested objects. This ensures that we do not overwrite entire objects inadvertently:
private User applyPatch(JsonPatch patch, User targetUser) {
ObjectMapper objectMapper = new ObjectMapper();
JsonNode targetNode = objectMapper.convertValue(targetUser, JsonNode.class);
// Apply the patch
JsonNode patchedNode = patch.apply(targetNode);
// Convert back to User
User updatedUser = objectMapper.treeToValue(patchedNode, User.class);
// Further merge nested objects here if needed
return updatedUser;
}
Step 5: Validating Changes
Finally, it’s essential to ensure that any changes made via PATCH requests still conform to your business rules.
private void validateUser(User user) {
if (user.getName() == null || user.getName().isEmpty()) {
throw new IllegalArgumentException("Name cannot be empty.");
}
// Additional validation logic for properties
}
You can integrate this method into the PATCH handling logic to ensure that all updates pass validation checks before being persisted.
Closing the Chapter
Transparent Patch Support in JAX-RS 2.0 is an effective way to enable efficient and reliable partial updates to resources. By leveraging JSON Patch operations, deep merging of nested objects, and validation, we can meet both client and server expectations for data integrity and efficiency.
For a deeper dive into managing JSON structures effectively with JAX-RS, refer to the official JAX-RS documentation and learn more about best practices in RESTful service design.
Embracing the techniques discussed in this post will only enhance your RESTful services, making them more adaptable and easier to maintain. Happy coding!
Checkout our other articles