Mastering ZonedDateTime to Date Conversion in Java
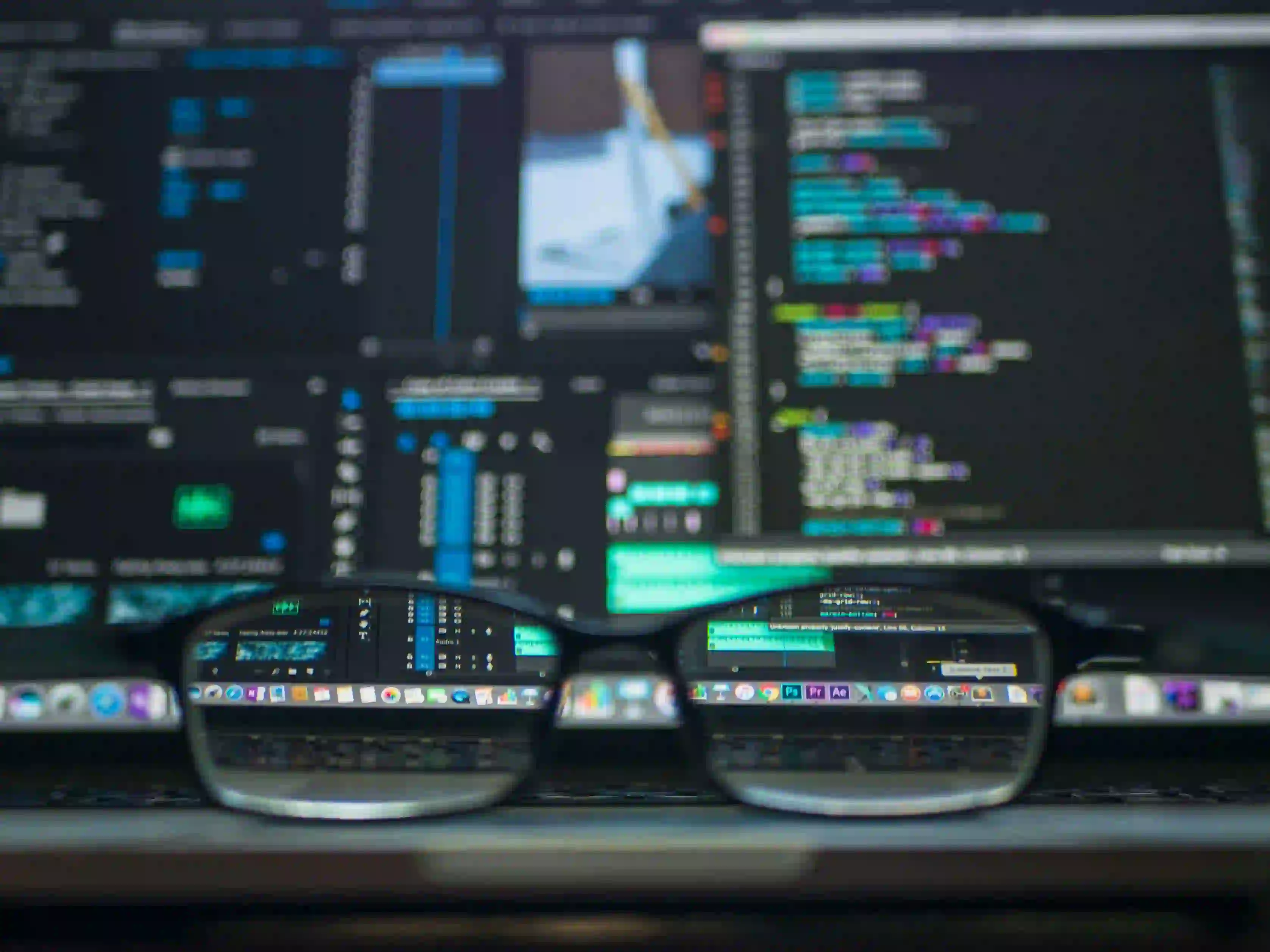
Mastering ZonedDateTime to Date Conversion in Java
In the world of Java programming, handling date and time can often be a tricky endeavor. With the introduction of the java.time
package in Java 8, managing dates became a lot easier. One of the most significant classes in this package is ZonedDateTime
. It represents a date-time with a time zone in the ISO-8601 calendar system. Understanding how to effectively convert ZonedDateTime
to Date
can enhance your applications by better handling time-sensitive functionalities.
In this blog post, we will dive into the details of ZonedDateTime
conversion. We will explore its structure, understand why you might need to convert it to the older Date
class, and provide concrete code examples to illustrate the conversion process.
What is ZonedDateTime?
ZonedDateTime
is a date-time with a time zone. It stores both the date-time and the time zone information offering a more precise representation than the traditional java.util.Date
class. This makes it particularly useful for applications that deal with global time zones.
Here’s how a ZonedDateTime
looks:
ZonedDateTime zonedDateTime = ZonedDateTime.now();
System.out.println(zonedDateTime);
Output Example
2023-10-01T15:30:00+05:30[Asia/Kolkata]
Why Convert ZonedDateTime to Date?
The Date
class is part of the Java standard library that existed long before Java 8 and isn't aware of time zones. People still use Date
for legacy reasons, or perhaps to interface with older APIs that haven't been updated.
For instance, if you are working with frameworks such as JDBC, Hibernate, or simply interacting with legacy code, converting ZonedDateTime
to Date
is often unavoidable.
How to Convert ZonedDateTime to Date
Let’s step through the conversion process. The primary steps include:
- Convert
ZonedDateTime
toInstant
. - Use
Instant
to create aDate
.
Step 1: Convert ZonedDateTime to Instant
Instant
is a point in time on the time-line, represented in seconds and nanoseconds.
ZonedDateTime zonedDateTime = ZonedDateTime.now();
Instant instant = zonedDateTime.toInstant();
System.out.println(instant);
Step 2: Create Date from Instant
The final piece involves creating an instance of Date
:
Date date = Date.from(instant);
System.out.println(date);
Complete Code Example
Here’s the complete conversion code:
import java.time.Instant;
import java.time.ZonedDateTime;
import java.util.Date;
public class ZonedDateTimeConversion {
public static void main(String[] args) {
// Create a ZonedDateTime instance
ZonedDateTime zonedDateTime = ZonedDateTime.now();
// Convert to Instant
Instant instant = zonedDateTime.toInstant();
// Convert Instant to Date
Date date = Date.from(instant);
// Output results
System.out.println("ZonedDateTime: " + zonedDateTime);
System.out.println("Converted Date: " + date);
}
}
Output Example
ZonedDateTime: 2023-10-01T15:30:00+05:30[Asia/Kolkata]
Converted Date: Sun Oct 01 09:00:00 UTC 2023
Important Considerations
1. Time Zone Awareness
When converting between ZonedDateTime
and Date
, keep in mind the loss of time zone information. The original time zone in ZonedDateTime
is not retained in the Date
.
2. Use of Instant
Converting through Instant
ensures that the point in timeline (UTC) is maintained. It’s crucial when dealing with applications that may require UTC-based date/time.
3. Legacy Code Handling
In situations where legacy code is written around Date
, this conversion proves vital. Always ensure proper handling to avoid unexpected behaviors.
Summary
In conclusion, converting ZonedDateTime
to Date
is an operation that every Java programmer should master. The methodology is straightforward, leveraging the Instant
class to bridge the gap between ZonedDateTime
and Date
.
Exploring such capabilities not only enhances your applications but also prepares you for modern Java best practices. Understanding these constructs helps in globalization efforts and ensures that applications behave consistently across different regions.
If you're interested in learning more about Java's date and time API, consider checking out the official Java Documentation for deeper insights.
Follow Us
Stay tuned for more articles on Java programming, including best practices, tips, and more advanced topics. Don’t forget to subscribe for notifications about our latest posts!
Happy coding!
This blog post serves as a quick guide to converting ZonedDateTime
to Date
while explaining the rationale behind these conversions and the relevant code examples. You can utilize this knowledge in larger applications where time-handling is a critical component.