Maximize Code Coverage: Tackling Unit vs Integration Tests
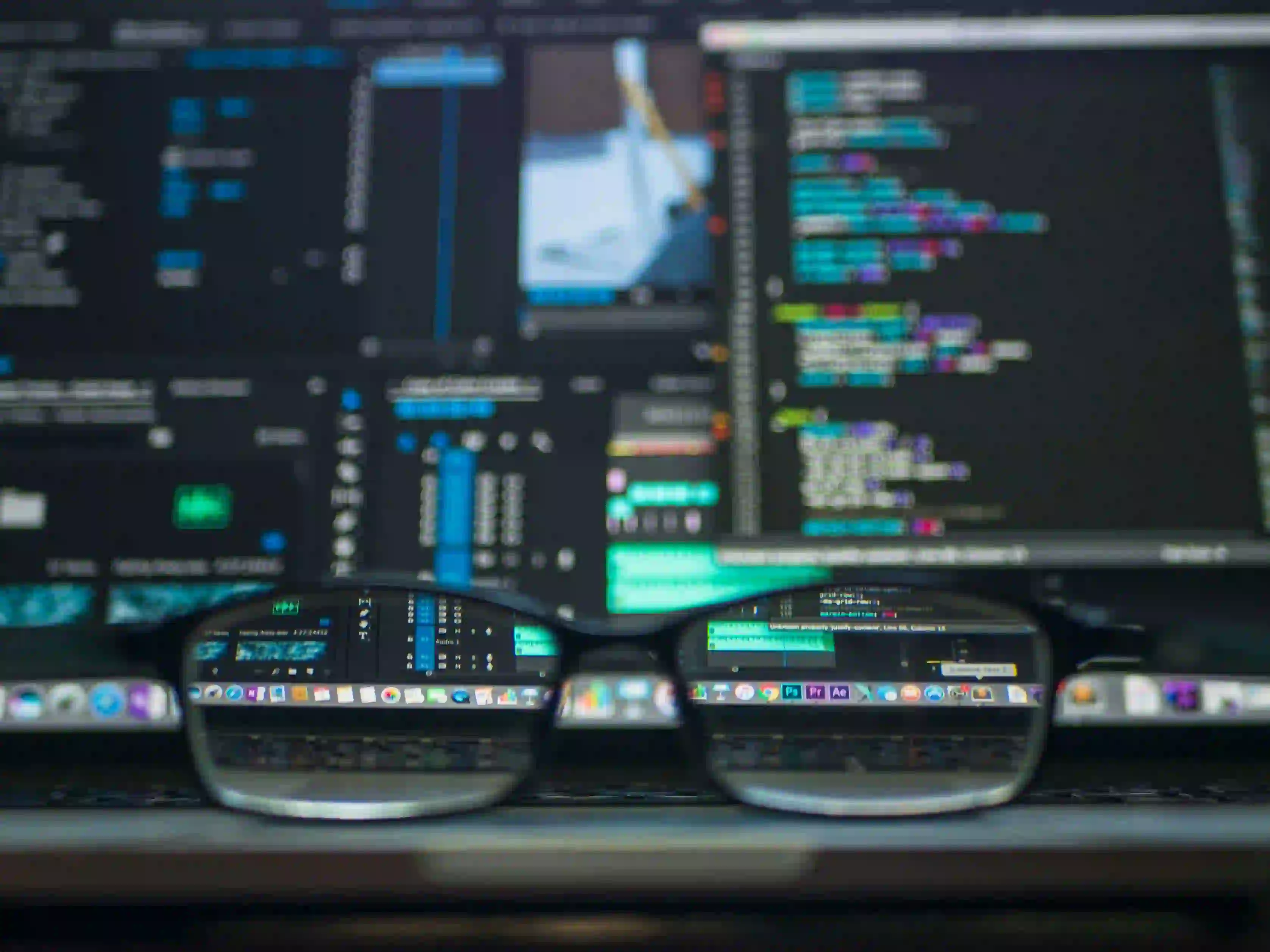
Maximize Code Coverage: Tackling Unit vs Integration Tests
In the world of software development, ensuring quality and reliability is paramount. One significant metric that developers often discuss is code coverage. Understanding how to maximize code coverage often leads to a healthy debate between two types of testing: unit tests and integration tests. This article dives deep into both, their purposes, and how developers can leverage them to achieve maximum code coverage.
What is Code Coverage?
Code coverage is a measure used to determine the extent to which your code has been executed when tests are run. It’s expressed as a percentage, indicating how much of your program is covered by tests. Higher code coverage is often associated with fewer bugs and better overall code quality.
Why is Code Coverage Important?
- Quality Assurance: Higher code coverage increases the likelihood that bugs are caught during testing.
- Refactoring Confidence: When you have comprehensive tests, you can refactor your code with assurance.
- Documentation: Tests serve as living documentation, helping new developers understand code functionality.
According to a recent survey, 85% of organizations believe skipping code coverage metrics leads to poor quality software.
Unit Tests: The Building Blocks of Testing
What are Unit Tests?
Unit tests are designed to test individual components or functions of your code in isolation. They ensure that each component behaves as expected.
Benefits of Unit Tests
- Faster Feedback: Unit tests are typically faster to run and execute than integration tests.
- Isolation: They focus on a single part of the application, making debugging easier.
Example of a Unit Test in Java
Here’s a simple Java example employing JUnit to test a method that adds two numbers:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
class CalculatorTest {
@Test
void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
Commentary
In the Calculator
class, the add
method is isolated and thoroughly tested.
- Why? By focusing on a single responsibility (adding two numbers), we can pinpoint issues without being influenced by other parts of the application.
The JUnit framework provides annotations like @Test
to mark test methods, allowing for easy execution and validation of results.
Best Practices for Unit Testing
- Write Tests First: Adopt Test-Driven Development (TDD) practices by writing tests before the actual code.
- Maintain Simplicity: Keep tests simple and straightforward.
- Use Mocks: Mock dependencies to focus on the unit itself.
Integration Tests: The Bigger Picture
What are Integration Tests?
Integration tests assess how various modules or services work together. They verify that different components of your application communicate correctly and the overall system functions as intended.
Benefits of Integration Tests
- Real-World Scenarios: They validate the interaction between components under realistic scenarios.
- Identify Interface Issues: Integration testing helps catch communication issues that may not be apparent in unit tests.
Example of an Integration Test in Java
Below is a simple integration test using Spring Boot’s testing capabilities:
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest;
import org.springframework.test.web.servlet.MockMvc;
@WebMvcTest(HelloController.class)
class HelloControllerIntegrationTest {
@Autowired
private MockMvc mockMvc;
@Test
void testHelloEndpoint() throws Exception {
mockMvc.perform(get("/hello"))
.andExpect(status().isOk());
}
}
Commentary
In this integration test of a Spring Boot application, we are validating that the /hello
endpoint responds appropriately.
- Why? This test ensures that the
HelloController
communicates with the web layer as expected, confirming the integration of multiple components.
Best Practices for Integration Testing
- Use Real Data: Whenever possible, use real data sets that reflect your production environment.
- Limit External Dependencies: Mock external services when feasible.
- Run Regularly: Ensure integration tests are part of your continuous integration pipeline to catch integration issues early.
Unit vs Integration Testing: The Balance
When to Use Unit Tests
- Early Development Phase: Use unit tests primarily in the early stages of development to validate individual functions.
- Small Changes: When modifications are confined to specific units.
When to Use Integration Tests
- After Unit Tests: Use integration tests once unit tests are in place to confirm that the system works cohesively.
- System Changes: When major changes that impact multiple components are made.
Strategies to Maximize Code Coverage
Combine Unit and Integration Tests
The best approach is to utilize both unit and integration tests effectively. While unit tests cover the granular details of each function, integration tests ensure that these functions work harmoniously within the larger system.
Start with Unit Tests
Focus initially on unit tests as they lay a solid foundation. Once confident that individual units function correctly, you can proceed to integration tests.
Use Coverage Tools
Utilize tools like JaCoCo or Cobertura to measure your code coverage rigorously. This will help you identify areas needing more tests.
- JaCoCo provides a seamless integration with the build process.
Continuous Integration
Incorporate your tests into a continuous integration (CI) pipeline. This process ensures that tests run every time code is pushed, identifying new failures promptly.
A Final Look
Maximizing code coverage requires a strategic combination of unit and integration testing. While unit tests ensure individual components function as intended, integration tests validate that these components work together seamlessly. Utilizing both testing strategies while adhering to best practices and regularly measuring coverage positions software projects for success.
Investing time in both testing types will elevate your codebase’s reliability, maintaining quality as your project grows. So, get started today, and ensure that your code is not just written, but thoroughly tested.
For further reading on testing best practices, check out Martin Fowler's Testing Pyramid which offers an insightful strategy for unit and integration testing!
By understanding and implementing comprehensive testing strategies, developers enhance their code quality, reduce bugs, and deliver more reliable software solutions.