Improving Performance: Fixing Entity Cache Issues in Apps
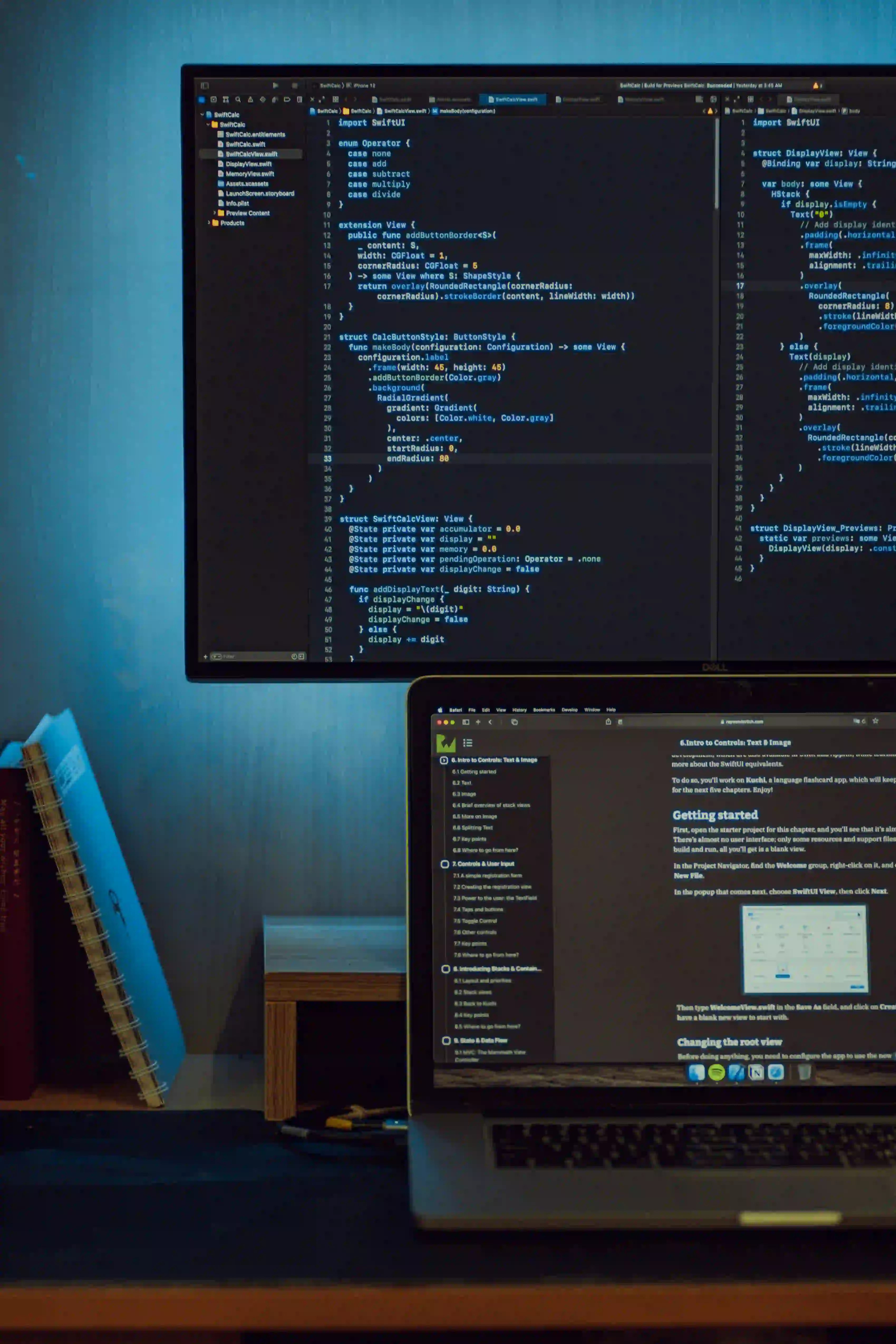
Improving Performance: Fixing Entity Cache Issues in Apps
In the world of software development, application performance is paramount. One of the essential aspects contributing to an app's performance is caching. Caching allows your application to store frequently accessed data in memory, significantly speeding up response times and reducing the load on your database. However, when used improperly, entity caching can lead to performance bottlenecks. In this blog post, we will explore entity caching, common pitfalls, and strategies to resolve caching issues to improve your application's performance.
What is Entity Caching?
Entity caching refers to the practice of storing instances of database entities - such as user profiles or product information - in memory for quick retrieval. In object-relational mapping (ORM) frameworks like Hibernate or Spring Data JPA, entity caches enable developers to avoid repeated database calls for the same entity within a given session or transaction.
By keeping data in memory, developers can provide faster access to frequently needed information. However, ineffective management of this cache can result in stale data, excessive memory usage, and increased complexity.
Why Entity Cache Issues Matter
-
Performance Implications: If your application retrieves stale or incorrect data because of cache issues, it leads to a poor user experience. In some cases, it may even cause critical failures.
-
Increased Memory Usage: Although caching is meant to speed up performance, a poorly configured entity cache can lead to excessive memory consumption, which can slow down your application instead.
-
Complexity and Maintenance: Managing entity caches can introduce additional complexity in code. Improper handling may require more time and resources for debugging and maintenance.
Common Entity Cache Issues
Let's break down some common issues associated with entity caching:
Stale Data
Caching stale data occurs when your application retrieves outdated information despite having updated in the database. This can happen when cached entities are not refreshed after a modification. For example, if you update a user’s profile but the repository still serves up the cached version, users will not see their updates.
Memory Leaks
If your application caches too many entities without proper eviction policies, you might face memory leaks. As more entities are cached over time, your application could run out of memory leading to crashes or slow performance.
Inefficient Cache Strategies
Using caching mechanisms that do not suit your application's needs can lead to bottlenecks. For instance, using a single cache layer for all types of entities can hinder performance. A more efficient strategy would involve segregating different types of entities into separate caches.
How to Improve Entity Caching
1. Use Proper Cache Invalidation
One of the best practices for maintaining a robust entity cache is implementing proper cache invalidation strategies. Here’s how you could handle it:
- Explicit Invalidation: When performing create, update, or delete operations, explicitly invalidate the corresponding cache entries.
@Transactional
public void updateUser(User user) {
userRepository.save(user);
entityManager.getEntityManagerFactory().getCache().evict(User.class, user.getId());
}
Why: This snippet ensures that the cache entry for a user is removed whenever the user is updated.
- Time-Based Invalidation: Alternatively, you can set a time-to-live (TTL) for your cached entities. After a specified duration, the cached data will automatically be evicted.
2. Implement Proper Cache Strategies
Adopt a caching strategy tailored to your application requirements. For example, consider using different caches for read-heavy and write-heavy applications.
Example Strategy: Read-Through Cache
public User getUserById(Long id) {
User cachedUser = userCache.get(id);
if (cachedUser != null) {
return cachedUser; // Return from cache
}
// If not found in cache, retrieve from database
User user = userRepository.findById(id);
if (user != null) {
userCache.put(id, user); // Cache it
}
return user;
}
Why: This strategy ensures that if the user entity does not exist in the cache, it fetches it from the database and stores it for future requests.
3. Tune Cache Configuration
Every ORM and caching library provides configurations that you can tune. For example, if you are using Hibernate, you can configure the second-level cache using various providers like Ehcache, Infinispan, or even Redis. The following example shows how to configure Hibernate with Ehcache:
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
<property name="hibernate.cache.use_query_cache">true</property>
Why: This configuration enables the second-level cache in your Hibernate application, which can greatly improve performance for read operations.
4. Monitor Performance Metrics
Keeping an eye on your application's performance is necessary for tuning and improving entity caches. Use libraries such as Micrometer or Spring Boot Actuator to monitor cache hit rates, memory usage, and database query performance.
5. Review Entity Relationships
Entity relationships can complicate caching, especially with bi-directional relationships. Analyze your relationships carefully to determine the suitable cache strategy.
-
One-to-Many Relationships: Consider caching parent entities but not always the child entities, especially if they can change frequently.
-
Many-to-Many Relationships: Be cautious with caching as changes in one entity could involve multiple updates in related entities. You may prefer to cache the results of specific queries instead.
6. Use Distributed Caches Where Necessary
In a microservices architecture, consider employing distributed caching solutions like Redis or Memcached. These options provide global caching capabilities accessible by multiple services.
@Bean
public RedisTemplate<String, User> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, User> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
return template;
}
Why: This configuration allows you to cache user entities distributed across different instances of your application, allowing more scalable performance improvement.
My Closing Thoughts on the Matter
Entity caching can dramatically enhance the performance of your applications when done correctly. Understanding the common pitfalls and implementing strategies such as cache invalidation, proper configurations, and distributed caches can help you make the most of caching mechanisms.
Incorporating these best practices into your application development process not only improves performance but also results in a better user experience. Monitoring performance metrics and adjusting your caching strategies based on real-time data will ensure that your application continues to perform optimally as it evolves.
If you want more information on managing caches with specific frameworks, consider checking the following resources:
- Hibernate Caching Documentation
- Spring Cache Documentation
- Redis Caching Guide
By applying these strategies, you will not only improve your application’s performance but also build a more maintainable and user-friendly system. Let's make caching work for you!