Common Pitfalls in Init-D Scripts for Play Framework Apps
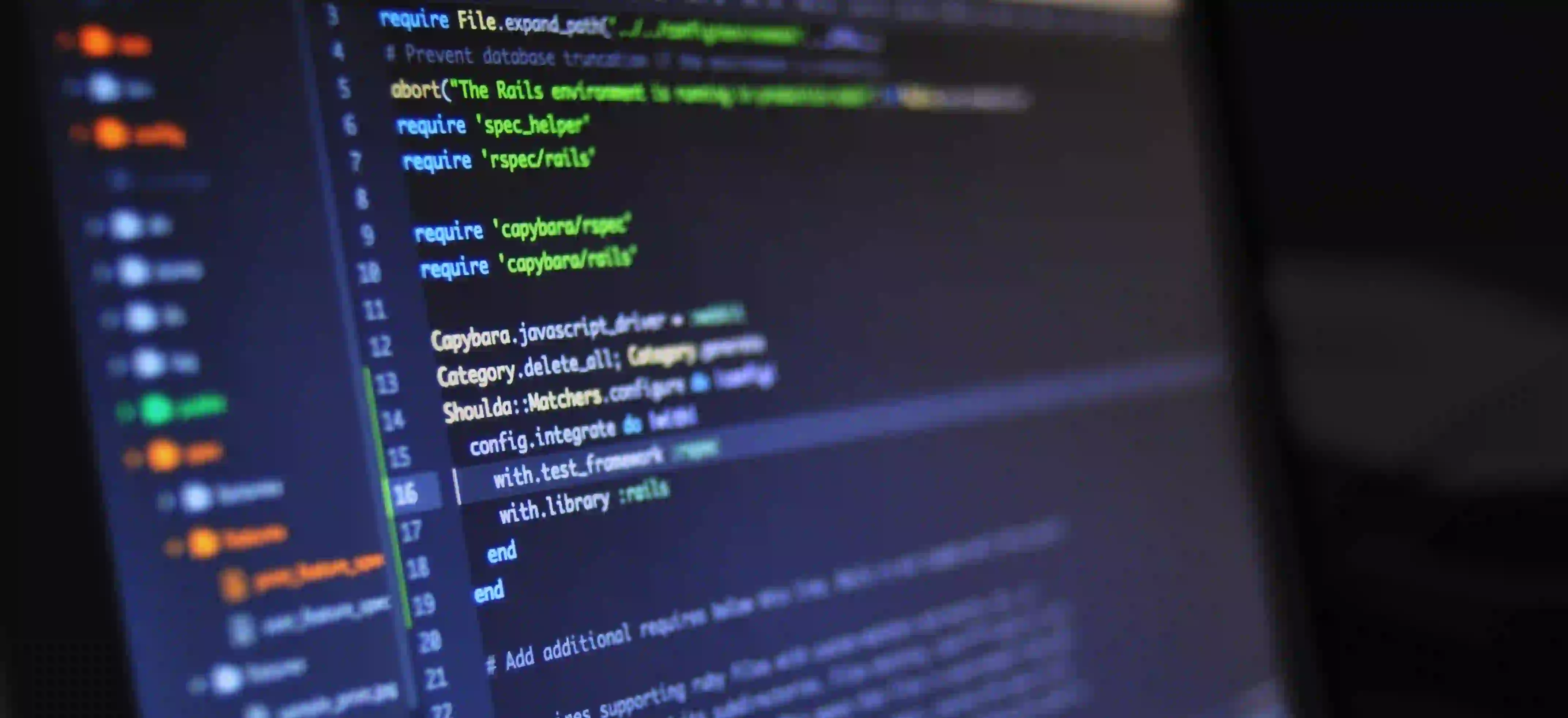
Common Pitfalls in Init-D Scripts for Play Framework Apps
When deploying Play Framework applications, one of the critical areas developers often overlook is the initialization scripts (Init-D scripts) on Linux systems. These scripts orchestrate the startup and shutdown of your applications, and if not correctly configured, they can lead to a variety of issues, including failed deployments and inefficient resource usage.
In this blog post, we will explore common pitfalls in Init-D scripts specifically tailored for Play Framework applications, discuss best practices, and provide exemplary code snippets. By the end, you'll have a solid understanding of how to avoid these pitfalls, leading to more robust and reliable deployments.
Understanding Init-D Scripts
Init-D scripts are shell scripts stored in the /etc/init.d/
directory on Unix-based systems. They are responsible for starting, stopping, and managing services on the system, and they can handle different run levels as designated by the system administrator.
For Play Framework applications, which typically run on the Java Virtual Machine (JVM), Init-D scripts can be particularly useful for handling lifecycle management.
Common Pitfalls
-
Not Using the Correct Java Version
One of the foundational aspects of deploying a Play Framework app is ensuring the correct Java version is in use. The Play Framework requires Java 8 or higher, depending on the version you are using.
Code Snippet:
🔧snippet.sh#!/bin/bash # Check for Java version JAVA_VERSION=$(java -version 2>&1 | awk -F '"' '/version/ {print $2}') if [[ "$JAVA_VERSION" < "1.8" ]]; then echo "Java 8 or higher is required." exit 1 fi
Why? This snippet checks the installed Java version and prevents the script from proceeding if the version is below the required standard. By ensuring you're using the right Java version, you can avoid runtime errors and application failures.
-
Hardcoding Paths
Another common mistake is hardcoding paths within the scripts. This can lead to deployment issues, especially when migrating between environments (development, testing, production).
Code Snippet:
🔧snippet.sh#!/bin/bash APP_DIR="/opt/my-play-app" JAVA_CMD="/usr/bin/java" start() { cd $APP_DIR nohup $JAVA_CMD -Dconfig.file=$APP_DIR/conf/application.conf -Dhttp.port=9000 -jar $APP_DIR/my-play-app.jar &> $APP_DIR/logs/my-app.log & echo $! > $APP_DIR/my-app.pid }
Why? By defining
APP_DIR
andJAVA_CMD
variables, the script becomes more dynamic. You can easily change these values as needed, enhancing the flexibility and maintainability of your code. -
Not Handling Ulimits
Java applications, especially those running on Play Framework, can require a significant number of file descriptors. Failing to set proper limits (
ulimits
) can cause your application to fail under load.Code Snippet:
🔧snippet.sh#!/bin/bash ulimit -n 4096 # Increase the limit for open file descriptors start() { # Start the application ... }
Why? Increasing the open file descriptors ensures your application can handle multiple connections without running into resource limits. This is especially important in production environments where concurrent connections can spike.
-
Ignoring Logging Management
Proper logging management is crucial for troubleshooting and monitoring applications. Not capturing logs can make diagnosing problems significantly harder.
Code Snippet:
🔧snippet.sh#!/bin/bash LOG_FILE="/var/log/my-play-app.log" start() { nohup $JAVA_CMD ... &> $LOG_FILE & echo "Started my-play-app, logging to $LOG_FILE" }
Why? Redirecting output to a log file allows you to track application behavior over time. It provides critical insights into errors, warnings, and normal operations, aiding in debugging and maintenance efforts.
-
Failing to Stop Applications Gracefully
Not managing the shutdown process can lead to incomplete requests and resource leaks. Always ensure that your scripts handle stopping the application gracefully.
Code Snippet:
🔧snippet.shstop() { if [ -f "$APP_DIR/my-app.pid" ]; then kill $(cat $APP_DIR/my-app.pid) echo "Stopped my-play-app" rm $APP_DIR/my-app.pid else echo "my-play-app is not running" fi }
Why? This snippet checks if the application is running by looking for a PID file, and only issues a stop command if it is. This reduces the risk of orphan processes and potential resource leaks.
Best Practices for Init-D Scripts
While we have highlighted common pitfalls and provided examples of handling them, here are additional best practices to employ when creating Init-D scripts for Play Framework apps:
-
Use a Process Manager: Instead of a basic Init-D script, consider using a process manager like systemd or supervisord. These tools provide more advanced features like automatic restarts, easier logging, and simpler management.
-
Environment Variables: Use environment variables for configuration values, especially sensitive data like database URLs or secrets.
-
Test Your Scripts: Make sure to test your scripts in a staging environment that closely resembles production. This will help identify potential issues before they affect your end users.
-
Document Your Scripts: Include comments within your scripts explaining the purpose of each section. Good documentation can save a lot of time for future developers.
-
Regular Updates: Keep your scripts updated according to best practices and changes in the Play Framework or JVM.
My Closing Thoughts on the Matter
Properly writing Init-D scripts tailored for Play Framework applications is crucial for successful deployments. By avoiding common pitfalls such as neglecting Java version checks, hardcoding paths, and failing to handle graceful shutdown procedures, you set your application up for reliability and performance.
Implementing best practices will help maintain the integrity of your application and make managing it in production much more straightforward. For those interested in diving deeper into Play Framework, the official documentation provides a wealth of information, and you can find it here.
By following the guidance laid out here, your Play Framework apps will be more robust, easier to manage, and less prone to errors.