Optimize Java Application Performance with Effective Profiling
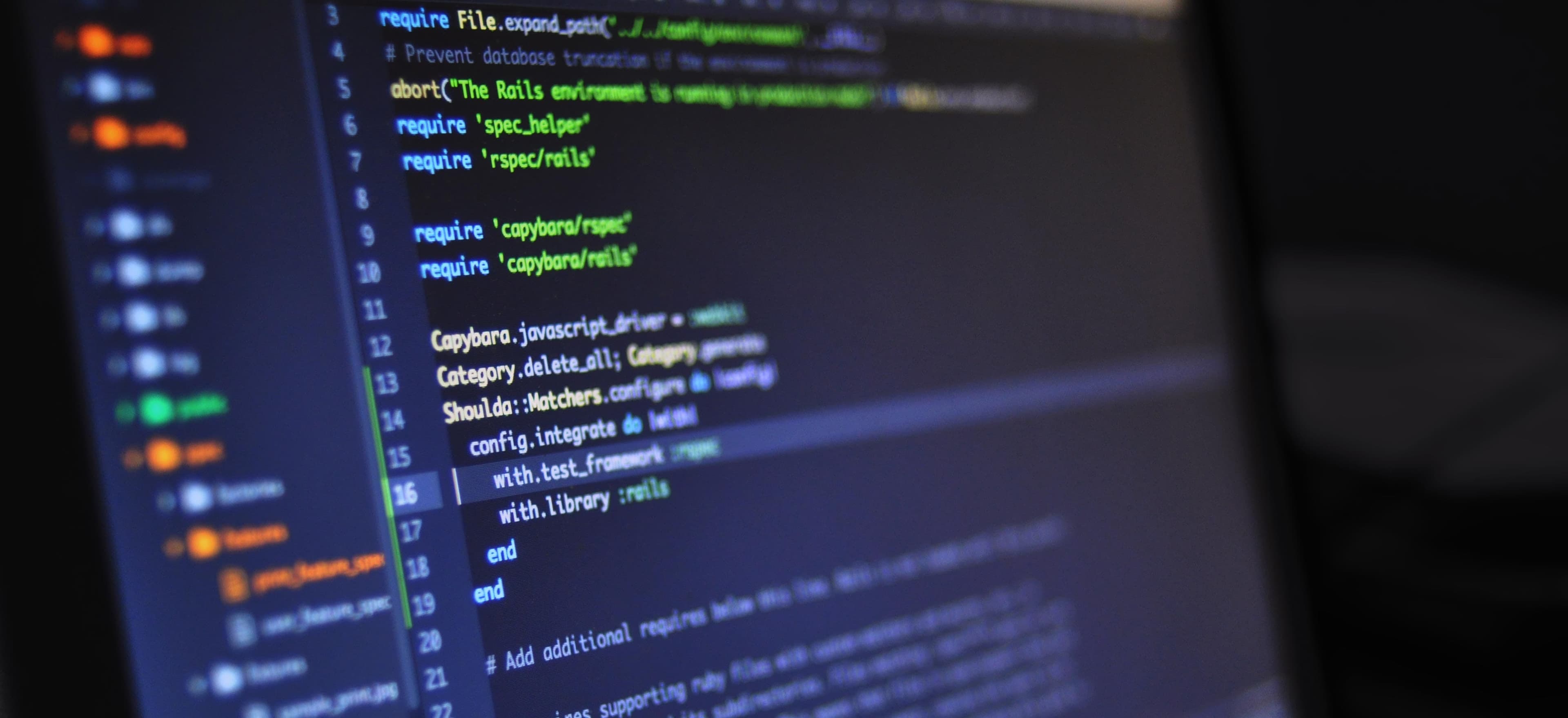
- Published on
Optimize Java Application Performance with Effective Profiling
Java is one of the most widely used programming languages, thanks to its versatility, maintainability, and the robust ecosystem surrounding it. However, as any developer knows, optimal performance is key to a successful Java application. Profiling is one of the most powerful tools that developers can employ to assess and improve performance. In this blog post, we will explore the concept of profiling in Java and how it can be used to enhance your application’s performance.
What is Profiling?
Profiling is the process of analyzing an application’s performance in order to identify bottlenecks and areas for improvement. It involves collecting data on various metrics such as CPU usage, memory consumption, and thread activity while the application is running. The collected data helps developers determine which parts of the code consume the most resources and need optimization.
Why is Profiling Important?
Profiling is crucial for several reasons:
-
Identify Bottlenecks: Profiling helps you discover slow parts of your code that take longer to execute or consume more memory.
-
Understand Resource Usage: You can understand how your application utilizes resources, allowing you to optimize accordingly.
-
Improves User Experience: An optimized application leads to faster response times, resulting in a better experience for users.
-
Facilitates Better Decision Making: With accurate profiling data, developers can make informed decisions on which parts of the code to optimize first.
Java Profilers: An Overview
There are several tools available for profiling Java applications:
-
Java VisualVM: A free tool that provides detailed information about the performance and memory consumption of Java applications running on the JVM.
-
YourKit: A commercial profiler that offers powerful features for performance monitoring and memory analysis.
-
JProfiler: Another commercial tool that combines CPU, memory, and thread analysis in a single interface.
-
Eclipse Memory Analyzer (MAT): A powerful tool for analyzing memory consumption and identifying memory leaks in Java applications.
In this post, we’ll focus on Java VisualVM, as it is readily available and easy to use.
Getting Started with Java VisualVM
Installation
Java VisualVM is included in the JDK, so if you have the JDK installed, you likely already have VisualVM. You can launch it from the command line by executing:
jvisualvm
Setting Up Profiling
To profile a running Java application:
- Launch Java VisualVM.
- Locate your Java application in the left panel under “Local”.
- Double-click your application to open it.
Let's discuss some vital profiling techniques you can use.
Key Profiling Techniques
1. CPU Profiling
CPU profiling helps you identify how much CPU time the various methods are using. To start CPU profiling in VisualVM:
- Open your application in VisualVM.
- Go to the "Profiler" tab.
- Click on "CPU".
This will start monitoring CPU usage for all methods. After some time, you can stop profiling and analyze the collected data.
Example Code Snippet
public void computeFibonacci(int n) {
if (n <= 1) {
return n;
}
return computeFibonacci(n - 1) + computeFibonacci(n - 2);
}
In the method computeFibonacci
, the algorithm has exponential time complexity. If profiling shows that this method is consuming a considerable amount of CPU cycles, you can replace it with a more efficient approach, like using memoization or an iterative solution.
2. Memory Profiling
Memory profiling reveals how much memory your application is consuming and where it is being used. To profile memory:
- In the VisualVM tool, navigate to the "Profiler" tab.
- Click on the "Memory" dropdown.
This will allow you to see objects that are allocating high memory usage. You can also perform heap dumps for further analysis.
Example Code Snippet
public List<String> generateData(int size) {
List<String> data = new ArrayList<>();
for (int i = 0; i < size; i++) {
data.add("Item " + i);
}
return data;
}
If this method is generating a list of strings that grows too large, consider re-evaluating your data structure or using a more memory-efficient approach (e.g., using a StringBuilder
).
3. Thread Profiling
Thread profiling allows you to understand how threads are behaving in your application. You can identify thread contention and deadlocks:
- Open the "Threads" tab in the profiler.
- You will see a list of current threads, their state, and how long they have been running.
Using Thread Dump
You can take a thread dump to analyze the states of threads at a specific time. This is useful for diagnosing deadlocks.
Example Code Snippet
public void task() {
synchronized (lock) {
// Critical section
}
}
If multiple threads are waiting on the same lock, profiling will show you contention. You might refactor the code to reduce locking or use a ConcurrentHashMap
instead of synchronized blocks.
4. Custom Profiling with Instrumentation
For more specific profiling, you can write custom instrumentation. This allows you to gather metrics such as method execution time manually.
Example Code Snippet
public void criticalMethod() {
long startTime = System.currentTimeMillis();
// Method logic here
long endTime = System.currentTimeMillis();
System.out.println("Execution time: " + (endTime - startTime) + " ms");
}
Using System.currentTimeMillis() at the beginning and end of your method provides insights into the method's execution time.
Best Practices for Effective Profiling
-
Profiling in a Production-like Environment: Always try to profile in an environment that simulates production to get accurate data.
-
Measure First: Run your application before making any changes. Identify the hotspots and areas of concern before optimizing.
-
Optimize Incrementally: Make changes gradually and profile after each significant change to determine the effects.
-
Review Regularly: Profiling should not be a one-time activity. Regular profiling can help catch performance issues early.
Final Considerations
Profiling is a fundamental step in optimizing Java applications. By using tools like Java VisualVM and applying effective profiling techniques, you can significantly improve the performance of your applications. From CPU and memory profiling to identifying threading issues, these insights allow you to make informed decisions that enhance performance and user experience.
Explore more about performance optimization in Java through the Java Performance Tuning guide. With continued practice and profiling, you can elevate your Java applications to new heights. Happy profiling!
Checkout our other articles