Mastering Exception Handling: Common Pitfalls to Avoid
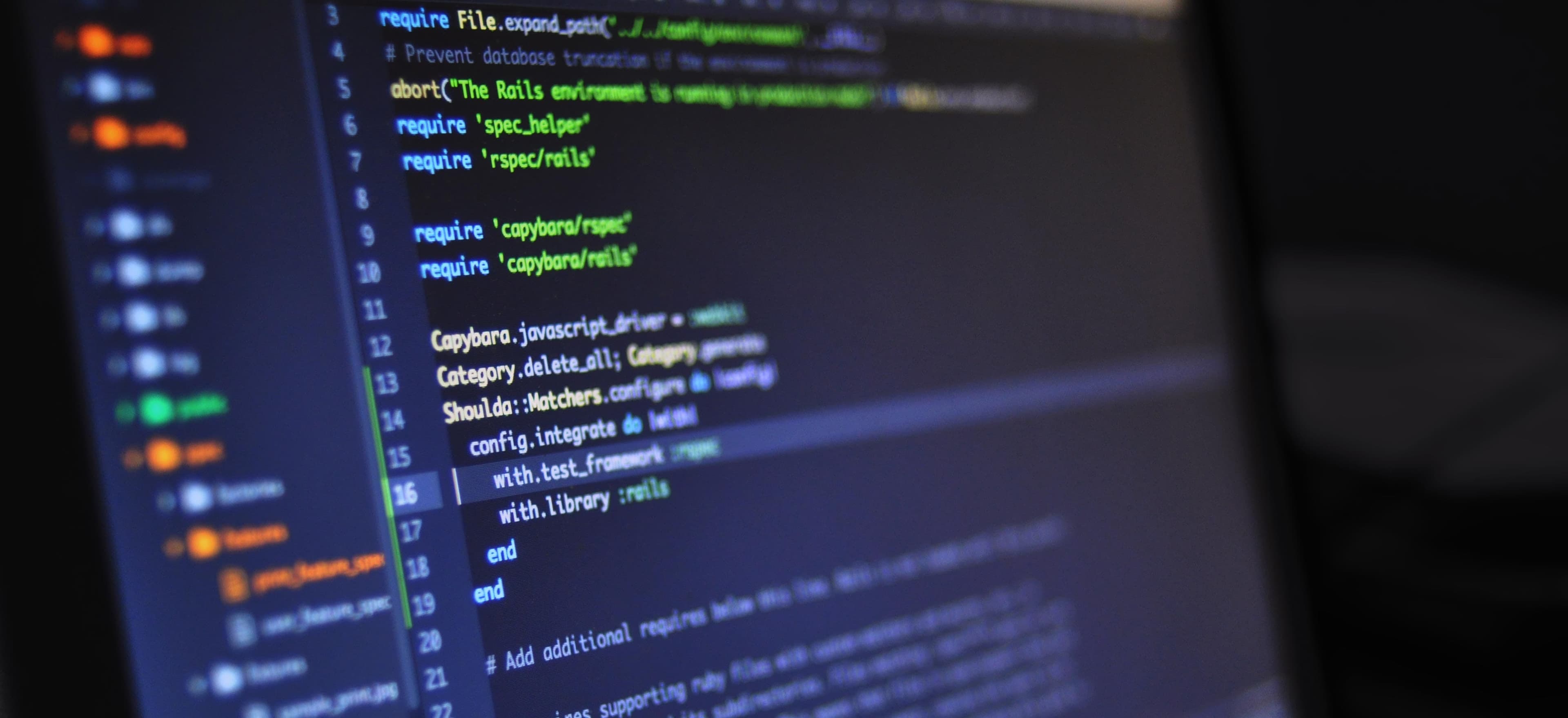
- Published on
Mastering Exception Handling: Common Pitfalls to Avoid
In the realm of Java programming, exception handling is both an art and a science. Mastering it ensures that your applications run smoothly, even when unexpected errors occur. But alongside its importance, there are numerous common pitfalls that developers can encounter. This blog will unravel these pitfalls, provide clarity, and ensure you grip the concept of exception handling firmly.
What is Exception Handling?
Before diving deep into the common pitfalls, let’s revisit the concept itself. Exception handling in Java is a powerful mechanism that allows developers to manage runtime errors, so the normal flow of application execution can continue. Java uses try
, catch
, and finally
blocks to help manage exceptions.
Basic Structure of Exception Handling
Here's a simple example that demonstrates the basic usage of exception handling in Java:
public class ExceptionHandlingExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
try {
// Attempt to access an invalid index
System.out.println(numbers[5]);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Caught an exception: " + e.getMessage());
} finally {
System.out.println("This block executes regardless of an exception.");
}
}
}
In this example, when attempting to access an invalid index of the array, an ArrayIndexOutOfBoundsException
is thrown. The catch
block captures the exception, allowing the program to continue gracefully. The finally
block runs regardless of whether an exception occurred or not, solidifying clean-up processes if needed.
Common Pitfalls in Exception Handling
Now, let's delve into some common pitfalls developers encounter while working with exceptions in Java, and how to avoid them.
1. Overusing Exceptions
The Pitfall
Using exceptions for regular control flow is a common mistake. Exceptions should only be used for exceptional conditions, meaning scenarios that aren’t part of the normal operation of your program.
The Example
public class OverusingExceptions {
public static void main(String[] args) {
try {
for (int i = 0; i < 10; i++) {
// This is incorrect. Using exceptions instead of regular flow control
if (i == 5) {
throw new Exception("Created an exception to break the loop.");
}
System.out.println(i);
}
} catch (Exception e) {
// Handle exception here
}
}
}
The Consequence
Overusing exceptions can lead to performance degradation. Since creating an exception object is costly in terms of performance, your code may become sluggish if exceptions are thrown frequently.
Best Practice: Use standard flow control mechanisms (like loops and conditionals) for regular operations. Save exceptions for truly unexpected events.
2. Swallowing Exceptions
The Pitfall
Developers sometimes catch exceptions without taking any action, often leaving the catch block empty. This is known as "swallowing" exceptions.
public class SwallowingExceptions {
public static void main(String[] args) {
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
// Exception swallowed, nothing done here
}
}
}
The Consequence
By ignoring the exception, you lose the opportunity to understand what went wrong, making debugging a nightmare.
Best Practice: Always log the exception or rethrow it. This fosters better maintenance and debugging.
catch (ArithmeticException e) {
System.err.println("Error occurred: " + e.getMessage());
throw e; // rethrow to propagate the exception
}
3. Not Using Finally Closures
The Pitfall
Forgetting to include a finally
block when necessary can lead to resources not being released appropriately, such as file handles or database connections.
The Example
public class FinallyBlockExample {
public static void main(String[] args) {
FileInputStream fis = null;
try {
fis = new FileInputStream("test.txt");
// Operations on the file
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
// File input stream is not closed, leading to resource leakage
}
}
The Consequence
Failing to properly close resources can lead to memory leaks and other undesired behaviors in your application.
Best Practice: Always close resources in a finally
block or, preferably, use the try-with-resources statement.
try (FileInputStream fis = new FileInputStream("test.txt")) {
// Operations on the file
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
4. Catching Generic Exceptions
The Pitfall
Catching generic exceptions, like Exception
or Throwable
, can mask issues and lead to poorer debugging experiences.
The Example
public class GenericExceptionHandling {
public static void main(String[] args) {
try {
String str = null;
System.out.println(str.length());
} catch (Exception e) {
// Too generic. What exception is actually caught here?
e.printStackTrace();
}
}
}
The Consequence
You may unwittingly hide specific errors, which can complicate troubleshooting and make the code less maintainable.
Best Practice: Always catch specific exceptions that you intend to handle.
catch (NullPointerException e) {
System.out.println("Caught a Null Pointer Exception: " + e.getMessage());
}
5. Ignoring Exception Hierarchies
The Pitfall
Not understanding exception hierarchies can lead to misuse of catch blocks and unnecessary code complexity.
The Example
public class ExceptionHierarchyExample {
public static void main(String[] args) {
try {
throw new IOException();
} catch (Exception e) {
// Captures everything through the Exception class and is not specific
System.out.println("An exception occurred: " + e.getMessage());
}
}
}
The Consequence
Using a broad catch-all exception might handle an error but it lacks clarity on what went wrong.
Best Practice: Familiarize yourself with the exception hierarchy in Java and catch the most specific possible exception.
6. Failing to Provide Context or Meaningful Error Info
The Pitfall
When logging exceptions or throwing them up the stack, many developers fail to include context or relevant information.
void process() {
try {
// Some process that can fail
} catch (IOException e) {
throw new RuntimeException(e); // No context provided
}
}
The Consequence
When debugging or looking through logs, you may not have enough context to understand what led to the failure, which can complicate issue resolution.
Best Practice: Always provide context in your exception messages.
catch (IOException e) {
throw new RuntimeException("Failed to process due to IO error: " + e.getMessage());
}
A Final Look
Mastering exception handling is crucial for robust Java applications. By avoiding these common pitfalls—overusing exceptions, swallowing them, neglecting proper cleanup, using generic exceptions, ignoring hierarchies, and skipping context—you can enhance the reliability and maintainability of your code.
Additional Resources
- Official Java Documentation on Exceptions
- Effective Java by Joshua Bloch
- Java Exception Handling Best Practices
By applying the practices outlined in this blog, you will be well on your way to becoming not just a competent Java developer, but a capable one skilled in the nuances of exception handling. Happy coding!
Checkout our other articles