Why AssertJ is Better Than Hamcrest for Unit Testing
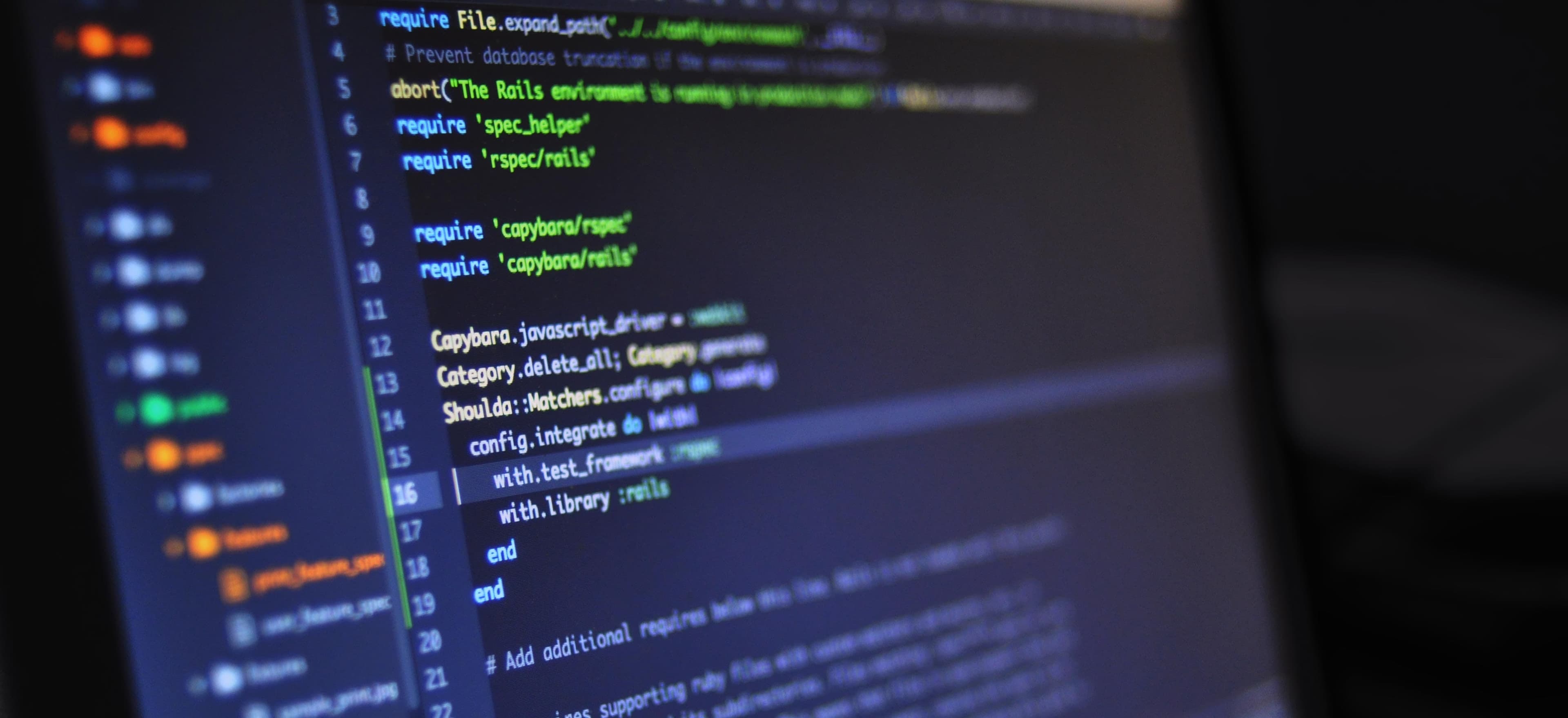
- Published on
Why AssertJ is Better Than Hamcrest for Unit Testing
Unit testing is the backbone of robust software development. While there are numerous testing frameworks available, two of the most popular libraries for assertions in Java are AssertJ and Hamcrest. This blog post dives deep into why AssertJ stands out as the preferred choice for many developers today.
Table of Contents
- Introduction to AssertJ and Hamcrest
- Fluent API and Readability
- Rich Set of Assertions
- Better Error Messages
- Integration with Java 8 Streams
- Conclusion
Starting Off to AssertJ and Hamcrest
Before delving deeper into their features and advantages, it is essential to understand what both libraries are.
Hamcrest
Hamcrest is a framework that provides matcher objects for writing tests. Using Hamcrest, you can create expressive tests that focus on assertions and avoid boilerplate code. A crucial aspect of Hamcrest is that it allows custom matchers.
AssertJ
AssertJ, on the other hand, is an advanced assertions library that builds upon the foundational strengths of Hamcrest. It provides a rich, fluent API for assertions, making it easier to create readable and maintainable unit tests.
Fluent API and Readability
One of the significant advantages of AssertJ over Hamcrest is its fluent API. The style of writing assertions in AssertJ is more natural and expressive.
AssertJ Example:
import static org.assertj.core.api.Assertions.assertThat;
public class UserServiceTest {
@Test
public void shouldReturnUser_whenUserExists() {
User user = userService.findUserById(1L);
assertThat(user).isNotNull()
.extracting("name", "email")
.containsExactly("John Doe", "john@example.com");
}
}
Hamcrest Example:
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.*;
public class UserServiceTest {
@Test
public void shouldReturnUser_whenUserExists() {
User user = userService.findUserById(1L);
assertThat(user, is(notNullValue()));
assertThat(user.getName(), is("John Doe"));
assertThat(user.getEmail(), is("john@example.com"));
}
}
Commentary: The AssertJ code is concise and clearly articulates the expected outcome. The expressiveness of using extracting
allows you to reveal more about the object in a single assertion. In contrast, Hamcrest's assertions are more verbose and less intuitive, which can reduce clarity.
Rich Set of Assertions
AssertJ comes with a comprehensive set of assertions that encompass a wide range of Java types, from collections to optional types. This richness makes it more versatile compared to Hamcrest.
Collections
With AssertJ, you can effortlessly perform complex assertions against collections.
AssertJ Example:
import static org.assertj.core.api.Assertions.assertThat;
public class UserServiceTest {
@Test
public void shouldReturnAllUsers() {
List<User> users = userService.findAllUsers();
assertThat(users).hasSize(3)
.extracting(User::getName)
.containsExactlyInAnyOrder("John Doe", "Jane Smith", "Alice Johnson");
}
}
Commentary: This unit test ensures the collection has the expected size and contains exactly the expected users. Hamcrest lacks the same level of control and specificity for collections, making testing groups of items cumbersome.
Better Error Messages
When capabilities are equal, it’s often the details that count. AssertJ provides more informative and user-friendly error messages when assertions fail.
AssertJ Example (Failure Scenario):
import static org.assertj.core.api.Assertions.assertThat;
public class UserServiceTest {
@Test
public void shouldReturnUser_whenUserExists() {
User user = userService.findUserById(1L);
assertThat(user).extracting("name")
.isEqualTo("Jane Doe"); // This will fail.
}
}
Error Message: The detailed error message will say something like: "Expected name to be 'Jane Doe' but was 'John Doe'".
Hamcrest Example (Failure Scenario):
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.*;
public class UserServiceTest {
@Test
public void shouldReturnUser_whenUserExists() {
User user = userService.findUserById(1L);
assertThat(user.getName(), is("Jane Doe")); // This will fail.
}
}
Error Message: The error message usually lacks specific context, leading to longer debugging times.
Integration with Java 8 Streams
AssertJ is tailored for modern Java practices, including seamless integration with Java 8 Streams. This makes it easier to write fluid, concise tests that benefit from functional programming’s expressiveness.
AssertJ Stream Example:
import static org.assertj.core.api.Assertions.assertThat;
public class UserServiceTest {
@Test
public void shouldHaveAllUserNamesUppercase() {
List<User> users = userService.findAllUsers();
assertThat(users)
.extracting(User::getName)
.allMatch(name -> name.equals(name.toUpperCase()));
}
}
Commentary: The above test leverages the flexibility of Streams and AssertJ’s assert methods to affirm that all names are uppercase. Hamcrest doesn't support this elegant style out-of-the-box.
To Wrap Things Up
Both AssertJ and Hamcrest have their own merits; however, AssertJ's fluency, richness of features, better error messages, and integration with modern Java practices gravity it ahead in the race for assertion libraries in Java.
If code readability, expressive error messages, and comprehensive assertions are critical for your unit testing strategy, assertion libraries like AssertJ are worth considering. Also, if you are new to these tools, you can check out AssertJ’s official documentation for further insights and examples.
Ensuring your tests are as clear and maintainable as possible is essential for long-term success in software development. Transition to AssertJ in your next project, and you may soon find it an indispensable tool in your development arsenal!