Common Pitfalls in Spring Boot Microservices Implementation
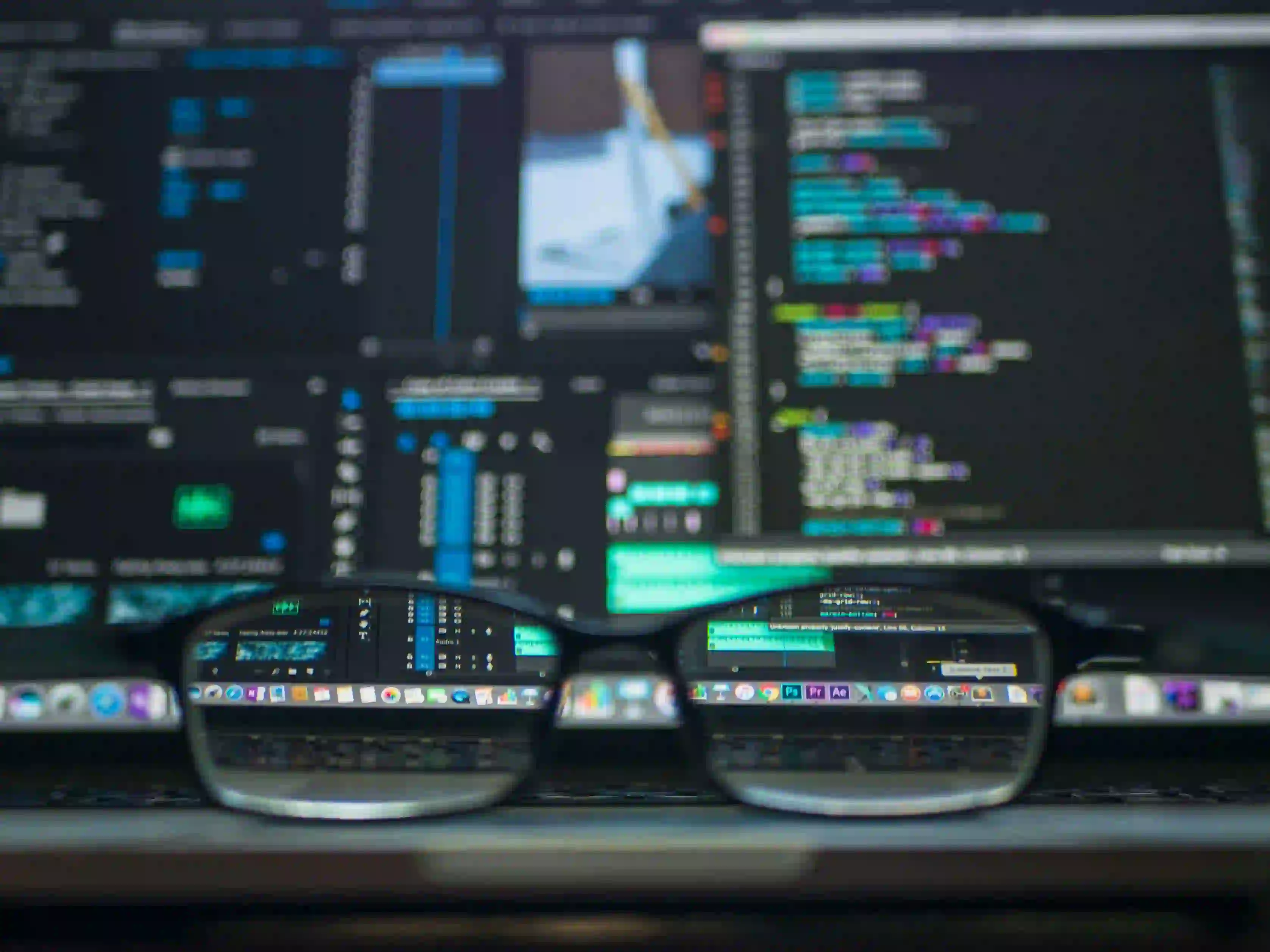
Common Pitfalls in Spring Boot Microservices Implementation
Spring Boot has revolutionized the way developers build Java applications, especially in the realm of microservices. With its ease of use and powerful features, it enables teams to rapidly create and deploy services. However, just because building microservices is more accessible, it doesn’t mean that the process is foolproof. In this blog post, we will discuss some common pitfalls in Spring Boot microservices implementation and how to avoid them.
Table of Contents
- Understanding Microservices Architecture
- Lack of Service Independence
- Poor API Design
- Overlooked Security Aspects
- Ignoring Logging and Monitoring
- Improper Error Handling
- Not Using Spring Cloud
- Conclusion
1. Understanding Microservices Architecture
Before diving into the pitfalls, it’s essential to understand the microservices architecture. Microservices allow developers to break down applications into smaller, independent services. Each service can be developed, deployed, and scaled independently, enabling more agility and flexibility in software development. However, this architecture can lead to complications if not executed properly.
2. Lack of Service Independence
The Pitfall
One of the biggest mistakes teams make is creating tightly coupled services that depend on each other for functionality. When services are interlinked, changes in one service can unwittingly affect another, leading to system failures.
Solution
To achieve true microservices independence, ensure each service has its own data store and is responsible for its own data. Interaction between services should occur through well-defined APIs.
Example of Using REST API Calls
Here’s a simple code snippet illustrating a service making a REST call to another service:
@Service
public class UserService {
private final RestTemplate restTemplate;
public UserService(RestTemplateBuilder builder) {
this.restTemplate = builder.build();
}
public User getUserDetails(Long userId) {
String uri = String.format("http://user-service/users/%d", userId);
return this.restTemplate.getForObject(uri, User.class);
}
}
Why it Matters: Using RESTful APIs allows you to decouple services, reducing interdependencies and enabling independent scaling.
3. Poor API Design
The Pitfall
A common mistake is neglecting proper API design. Poorly thought-out APIs can lead to confusion and misuse by clients, increasing maintenance overhead.
Solution
APIs should be intuitive and self-descriptive. Use versioning to ensure backwards compatibility and provide thorough documentation, such as Swagger or OpenAPI.
@RestController
@RequestMapping("/api/v1/users")
public class UserController {
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.findUserById(id);
return ResponseEntity.ok(user);
}
}
Why it Matters: A well-structured API promotes usability and maintainability, making it easier for clients to integrate and interact with your services.
4. Overlooked Security Aspects
The Pitfall
Security should never be an afterthought. Many teams implement services without proper security measures, exposing sensitive data to potential threats.
Solution
Leverage Spring Security to implement OAuth2 or JWT for securing your microservices. Always validate input and use HTTPS to encrypt data in transit.
Example of Basic Spring Security Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/v1/public/**").permitAll()
.anyRequest().authenticated();
}
}
Why it Matters: Security is critical for protecting user data and maintaining trust. Building security into the foundation of your services is crucial.
5. Ignoring Logging and Monitoring
The Pitfall
Microservices generate significant amounts of logging information. Ignoring logging can expose your application to failures that are hard to trace.
Solution
Implement centralized logging using tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk for effective log management.
logging:
level:
com.example: DEBUG
file:
name: logs/myapp.log
Why it Matters: Centralized logging helps in tracing issues efficiently and can greatly improve the debugging process.
6. Improper Error Handling
The Pitfall
A poorly structured error handling mechanism can lead to unresponsive services and a poor user experience.
Solution
Use a centralized exception handler to manage errors consistently across your services.
Example of Global Exception Handler
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND)
.body(new ErrorResponse(ex.getMessage()));
}
}
Why it Matters: Consistent error handling improves user experience and provides clear feedback to API consumers.
7. Not Using Spring Cloud
The Pitfall
Many developers overlook Spring Cloud, which provides essential tools for managing microservices.
Solution
Utilize Spring Cloud for service discovery (Eureka), API gateway (Zuul or Spring Cloud Gateway), and circuit breaker patterns (Hystrix).
Example of Setting Up Eureka Server
@EnableEurekaServer
@SpringBootApplication
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
Why it Matters: These features enhance scalability and fault tolerance in your application, simplifying microservices management.
8. Conclusion
While Spring Boot provides a powerful framework for building microservices, it’s important to navigate the common pitfalls carefully. By ensuring service independence, designing proper APIs, implementing strong security, maintaining logging, and handling errors effectively, you can build a robust microservices architecture.
For further reading, you may find these resources helpful:
By being mindful of these pitfalls and following best practices, you will enhance not only your microservices but also the overall architecture of your applications. Happy coding!