Unveiling Secrets: Processing Hidden Arrays in Neo4j CSVs
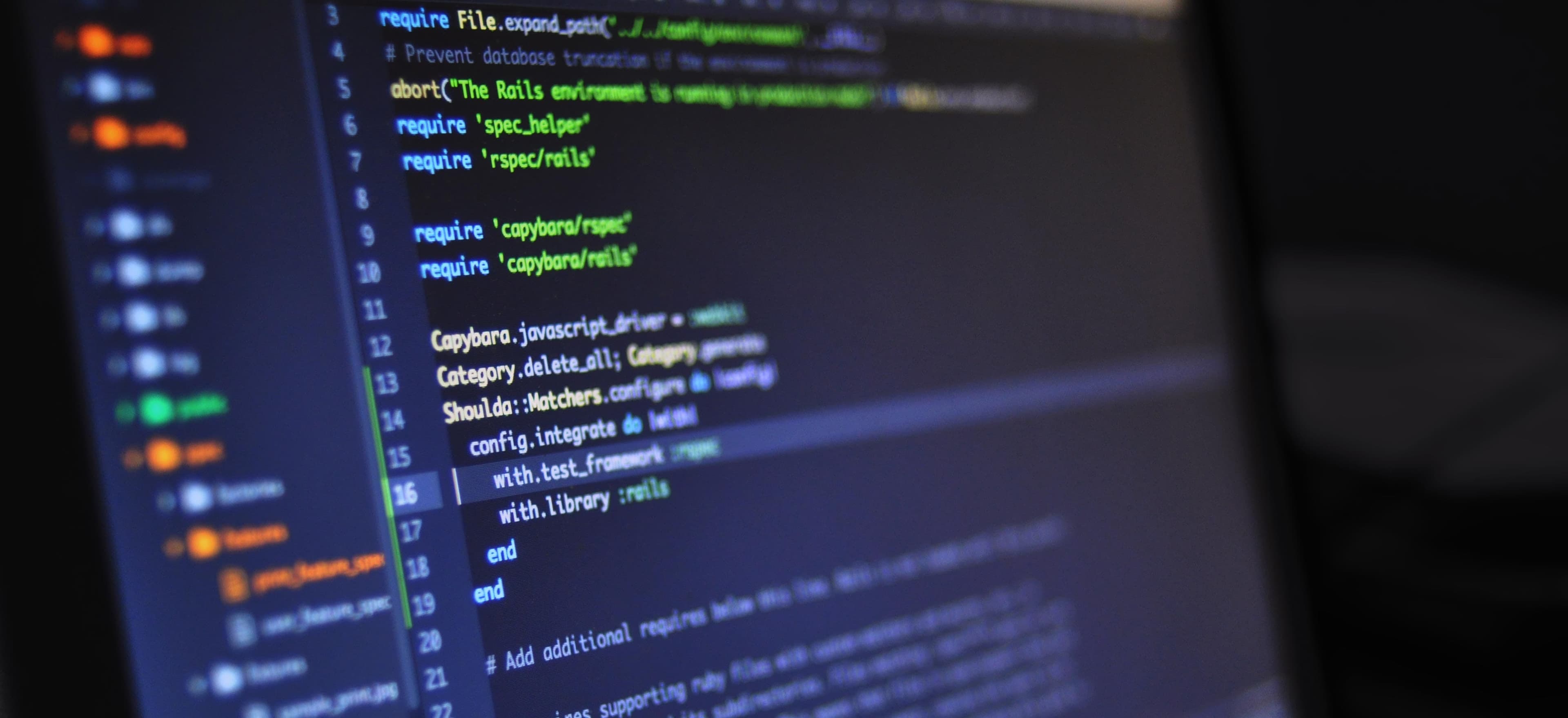
- Published on
Unveiling Secrets: Processing Hidden Arrays in Neo4j CSVs
Neo4j is a renowned graph database that provides an efficient way to represent and store data as nodes and relationships. It often involves importing data from external sources, such as CSV files, to populate the graph database. However, dealing with arrays in CSV files can be challenging, especially when the array elements are hidden within a single cell. In this blog post, we will explore how to process hidden arrays in Neo4j CSVs using Java, unraveling the secrets hidden within these complex datasets.
The Challenge
Imagine a CSV file containing data to be imported into Neo4j, where one of the columns contains an array of values separated by a delimiter, such as a comma. For example, consider a CSV file with the following structure:
id,name,skills
1,John,Drawing,Programming,Writing
2,Amy,Designing,Music
3,Michael,Programming,Photography
In this scenario, the 'skills' column contains hidden arrays where each cell represents a list of skills associated with an individual. To import this data into Neo4j, we need to process these hidden arrays and map the values to the corresponding nodes and relationships effectively.
The Solution
Step 1: Reading the CSV File
We start by reading the CSV file using a library like OpenCSV in Java. We can use the following code snippet to accomplish this:
Reader reader = Files.newBufferedReader(Paths.get("data.csv"));
CSVReader csvReader = new CSVReader(reader);
List<String[]> records = csvReader.readAll();
Step 2: Processing Hidden Arrays
To process the hidden arrays within the 'skills' column, we iterate through the records and split the 'skills' column into individual values. We can achieve this using the following code:
for (String[] record : records) {
String[] skills = record[2].split(",");
// Process the 'skills' array
for (String skill : skills) {
// Map the skill to the corresponding nodes and relationships in Neo4j
}
}
Step 3: Importing Data into Neo4j
Once the hidden arrays have been processed, we can proceed to import the data into Neo4j. We can utilize the Neo4j Java Driver to establish a connection and create nodes and relationships based on the processed data. Here's a simplified example of importing the data:
try (Session session = driver.session()) {
for (String[] record : records) {
String[] skills = record[2].split(",");
// Create a node for each individual
session.run("CREATE (p:Person {id: $id, name: $name})",
Values.parameters("id", record[0], "name", record[1]));
// Create relationships for each skill
for (String skill : skills) {
session.run("MATCH (p:Person {id: $id}) " +
"MERGE (s:Skill {name: $skill}) " +
"MERGE (p)-[:HAS_SKILL]->(s)",
Values.parameters("id", record[0], "skill", skill));
}
}
}
Step 4: Handling Complexity
When dealing with more complex arrays or JSON data within CSVs, utilizing a library like Jackson for JSON parsing can simplify the extraction of nested arrays or objects. Additionally, creating custom data models and using object mapping libraries like MapStruct can streamline the process further.
The Closing Argument
Processing hidden arrays within CSV files for import into Neo4j requires careful handling and manipulation of the data. Leveraging Java for this task provides the flexibility and control needed to effectively process and import complex datasets into the graph database. By following the steps outlined in this post, you can unravel the secrets hidden within hidden arrays and seamlessly populate your Neo4j graph database with structured and meaningful data.
In conclusion, understanding how to process hidden arrays in Neo4j CSVs empowers you to effectively integrate diverse datasets into a graph database, unlocking the full potential of your data. So next time you encounter hidden arrays in your CSV files, embrace the challenge and utilize the power of Java to unveil the secrets within.