Generating Alphabetic Sequence Using Java 8 Functions
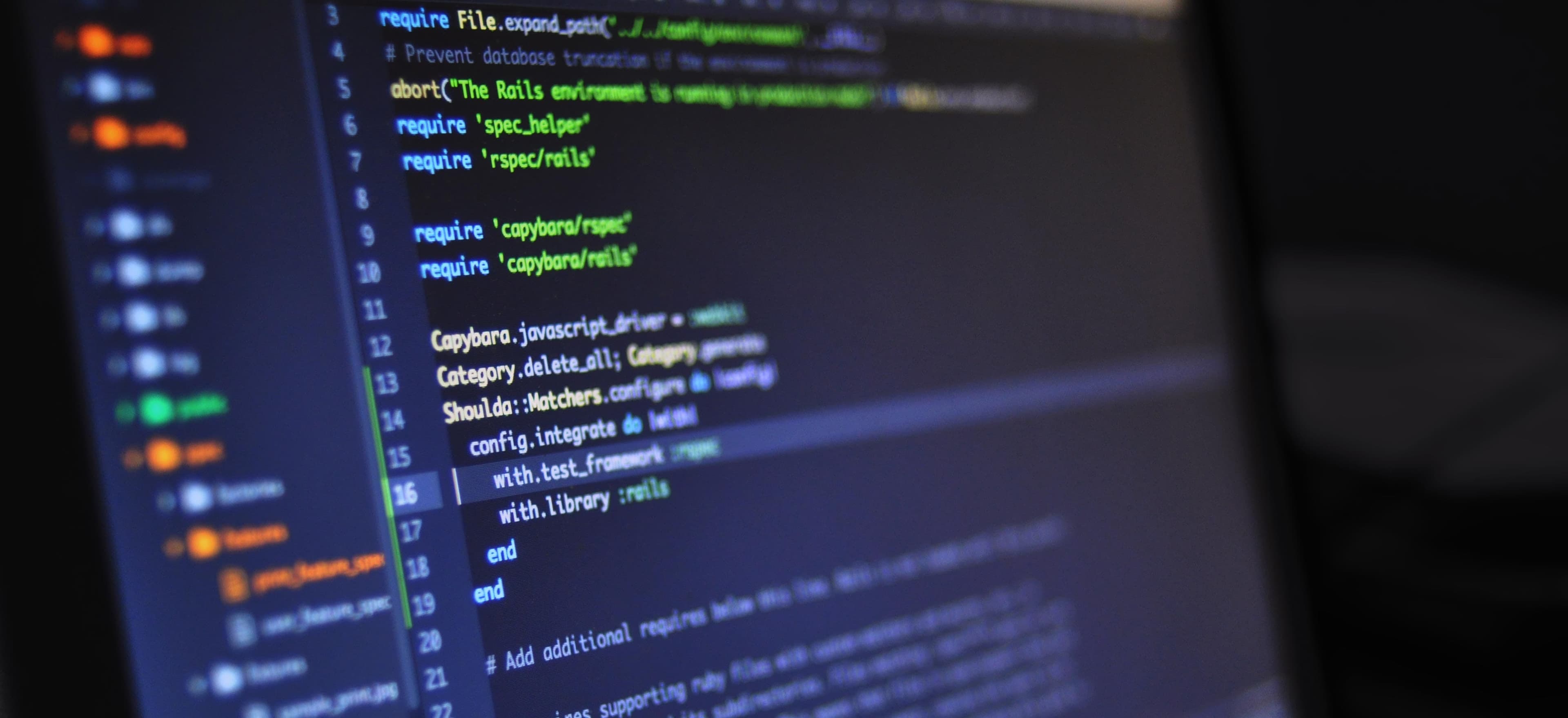
- Published on
Generating Alphabetic Sequence Using Java 8 Functions
In this post, we'll explore how to efficiently generate an alphabetic sequence using Java 8 functions. We'll utilize the powerful features of Java 8, such as Streams and functional interfaces, to simplify the code and make it more elegant.
The Problem
Sometimes, we need to generate an alphabetic sequence, either for testing purposes or as part of a larger algorithm. Traditionally, this might involve using loops and conditional statements to iterate through the characters of the alphabet. However, with the advent of Java 8, we can employ a more functional and concise approach.
Let's dive into the solution using Java 8!
Generating the Alphabetic Sequence
To generate the alphabetic sequence, we can use the IntStream
class to create a stream of integer values, and then map each integer to its corresponding alphabetic character.
import java.util.stream.IntStream;
public class AlphabeticSequenceGenerator {
public static void main(String[] args) {
// Generate alphabetic sequence from 'A' to 'Z'
IntStream.rangeClosed('A', 'Z')
.mapToObj(i -> (char) i)
.forEach(System.out::print);
}
}
In the above code, we use IntStream.rangeClosed('A', 'Z')
to generate a stream of integer values ranging from the ASCII value of 'A' to the ASCII value of 'Z'. Then, we use the mapToObj
method to convert each integer to its corresponding character, and finally, we use forEach
to print each character.
Explanation
-
IntStream.rangeClosed('A', 'Z')
: This method creates a stream of integers from the ASCII value of 'A' to the ASCII value of 'Z', inclusive. -
.mapToObj(i -> (char) i)
: Here, we use themapToObj
method to convert each integer to its corresponding character. This transformation is essential as it allows us to map the integer values to their alphabetic counterparts. -
.forEach(System.out::print)
: Finally, we use theforEach
method to print each character to the console.
Using this approach, we can generate the entire alphabetic sequence in a single line of code, making it concise and easy to understand.
Customizing the Sequence
If we want to generate a sequence from a different starting point or ending point, we can easily modify the rangeClosed
method to suit our needs.
For example, to generate a sequence from 'G' to 'M', we can simply change the range as follows:
IntStream.rangeClosed('G', 'M')
.mapToObj(i -> (char) i)
.forEach(System.out::print);
With this flexibility, we can generate alphabetic sequences tailored to our specific requirements.
To Wrap Things Up
In conclusion, Java 8 provides elegant and concise methods for generating alphabetic sequences using functional programming constructs such as Streams and functional interfaces. By leveraging these features, we can write more expressive and readable code while achieving the desired outcome.
By using the provided code examples and explanations, you can effortlessly generate custom alphabetic sequences in Java 8, making your code more efficient and maintainable.
So go ahead, experiment with different sequences, and leverage the power of Java 8 to simplify your alphabetic sequence generation needs!
Checkout our other articles