Mastering Minimal JavaFX Presentations: A Simple Guide
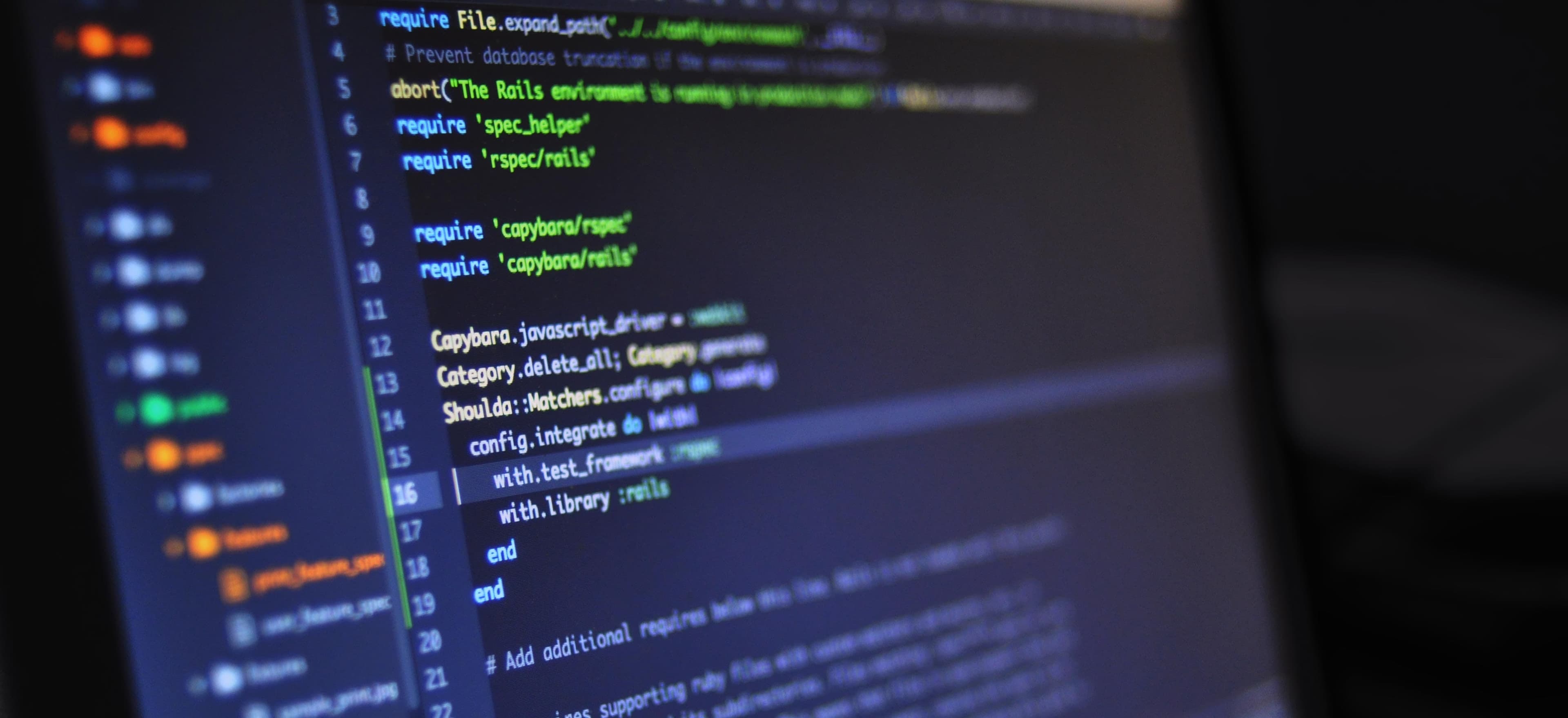
- Published on
Mastering Minimal JavaFX Presentations: A Simple Guide
JavaFX is a powerful framework for building rich client applications. When it comes to creating presentations or slideshows, JavaFX offers a flexible and customizable environment. In this guide, we'll explore how to create minimal, yet stunning presentations using JavaFX. We'll cover the basics of setting up a JavaFX project, creating slides, adding animations, and making the presentation interactive.
Setting Up the Project
To get started, let's create a new JavaFX project using your favorite IDE. Ensure you have JavaFX installed or included in your project's dependencies. If you're using Maven or Gradle, you can easily add JavaFX as a dependency in your pom.xml
or build.gradle
file.
Here's an example of the pom.xml
file with JavaFX dependencies:
<dependencies>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>16</version>
</dependency>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-graphics</artifactId>
<version>16</version>
</dependency>
</dependencies>
After setting up the project, we can proceed to create our presentation.
Creating the Presentation
In JavaFX, we can represent each slide as a separate scene. We'll create a Presentation
class to manage the slides and handle the transitions between them.
First, let's define a basic structure for our Presentation
class:
public class Presentation extends Application {
private Stage primaryStage;
private Group root;
private Scene scene;
private int currentSlideIndex;
// ... other methods and properties
}
In this class, we have a primaryStage
to represent the main window, a Group
called root
to hold the content of each slide, a Scene
to display the content, and an int
to keep track of the current slide index.
Next, we'll create a method to initialize the presentation and set up the initial slide:
@Override
public void start(Stage primaryStage) {
this.primaryStage = primaryStage;
this.root = new Group();
this.scene = new Scene(root, 800, 600, Color.WHITE);
this.primaryStage.setScene(scene);
this.primaryStage.setTitle("JavaFX Presentation");
// Set up the initial slide
setSlide(0);
this.primaryStage.show();
}
In the start
method, we initialize the primary stage, create a new scene with a white background, set the title of the presentation window, and then call the setSlide
method to display the initial slide.
Now, let's define the setSlide
method to switch between slides:
private void setSlide(int slideIndex) {
// Remove previous content
root.getChildren().clear();
// Add content for the new slide
switch (slideIndex) {
case 0:
// Add content for slide 1
break;
case 1:
// Add content for slide 2
break;
// Add more cases for additional slides
}
this.currentSlideIndex = slideIndex;
}
In the setSlide
method, we remove the previous content from the root Group
and add new content based on the slideIndex
. This method allows us to switch between different slides by updating the currentSlideIndex
.
Adding Content and Animations
For each slide, we can add various JavaFX nodes such as text, shapes, images, and even media players. Let's see an example of adding a simple title and subtitle to slide 1:
Text title = new Text("Welcome to JavaFX Presentation");
title.setFont(Font.font("Arial", FontWeight.BOLD, 36));
title.setX(100);
title.setY(100);
Text subtitle = new Text("A minimal guide to JavaFX presentations");
subtitle.setFont(Font.font("Arial", 24));
subtitle.setX(150);
subtitle.setY(150);
root.getChildren().addAll(title, subtitle);
In this code snippet, we create two Text
nodes for the title and subtitle, set their fonts, positions, and then add them to the root Group
.
To make the presentation more dynamic, we can add animations to the slides. Let's create a simple fade-in animation for the title and subtitle:
FadeTransition fadeTransition = new FadeTransition(Duration.seconds(2), title);
fadeTransition.setFromValue(0.0);
fadeTransition.setToValue(1.0);
fadeTransition.play();
fadeTransition = new FadeTransition(Duration.seconds(2), subtitle);
fadeTransition.setFromValue(0.0);
fadeTransition.setToValue(1.0);
fadeTransition.play();
In this example, we use the FadeTransition
to create a smooth fade-in effect for the title and subtitle. We specify the duration of the animation and the target node to animate.
Making the Presentation Interactive
To make the presentation interactive, we can handle user input to navigate between the slides. We can use keyboard events or incorporate buttons for navigation.
Let's add a simple keyboard event handler to switch between slides using the left and right arrow keys:
scene.setOnKeyPressed(event -> {
switch (event.getCode()) {
case LEFT:
if (currentSlideIndex > 0) {
setSlide(currentSlideIndex - 1);
}
break;
case RIGHT:
if (currentSlideIndex < maxSlideIndex) {
setSlide(currentSlideIndex + 1);
}
break;
default:
// Other key events
}
});
In this code snippet, we use the setOnKeyPressed
method of the scene to handle keyboard events. Depending on the pressed key, we update the current slide index using the setSlide
method.
Key Takeaways
In this guide, we've explored the fundamentals of creating a minimal JavaFX presentation. We set up a basic structure for the presentation, added content, animations, and made the presentation interactive. With JavaFX's versatility, you can further enhance your presentation by incorporating CSS styling, media elements, and custom transitions.
By following this simple guide, you're well on your way to mastering minimal JavaFX presentations and unlocking the potential for sophisticated, customized slideshows. Happy presenting!
Now, it's your turn to experiment with JavaFX and create captivating presentations. Dive into the code, unleash your creativity, and craft presentations that leave a lasting impression.
So, go ahead, embrace the power of JavaFX, and captivate your audience with visually stunning presentations tailored to your unique style!