Mastering JAX-RS: Easy Bean Validation i18n Error Messages
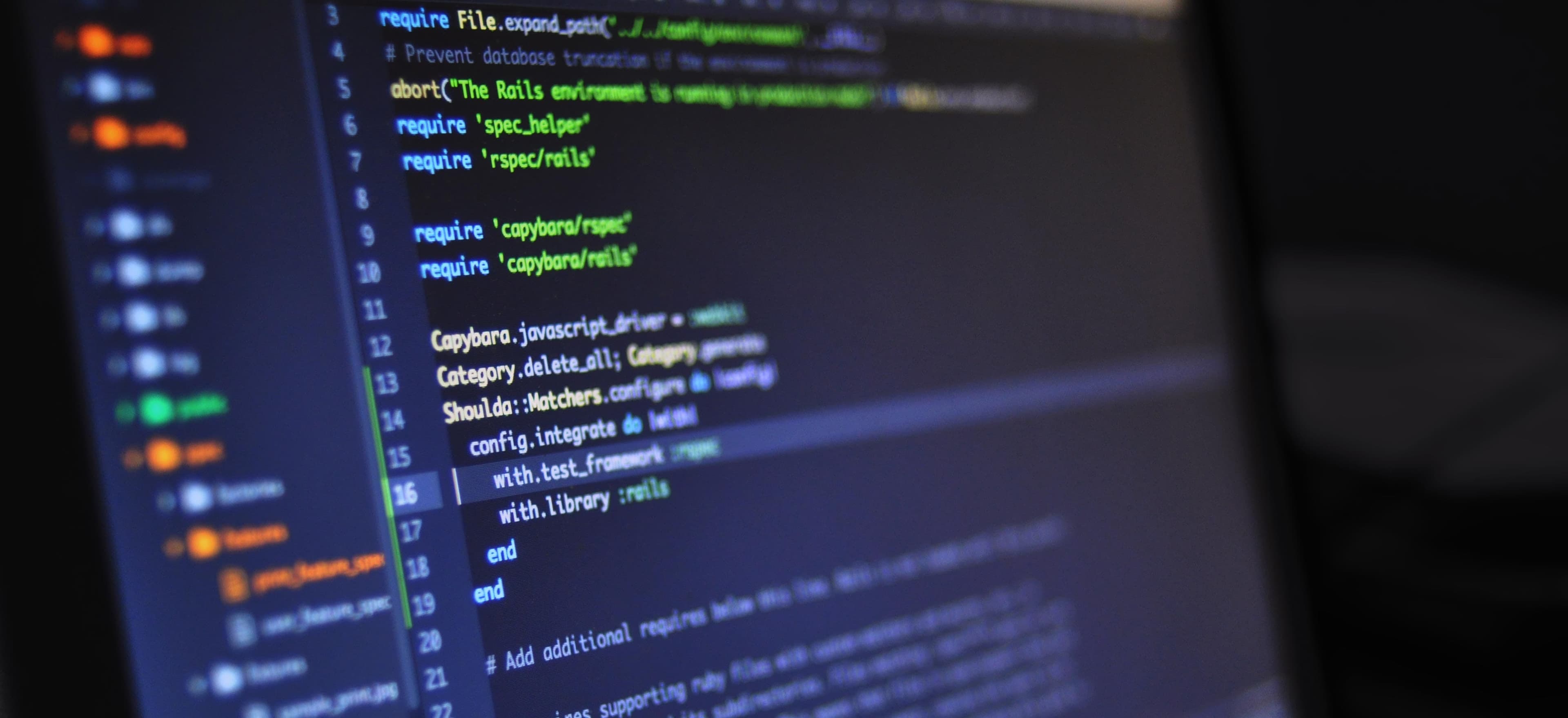
- Published on
Mastering JAX-RS: Easy Bean Validation i18n Error Messages
When developing a Java application with JAX-RS, ensuring that the API endpoints handle input validation efficiently is crucial. One way to achieve this is by utilizing Bean Validation, which provides a declarative way to validate Java beans. However, the default error messages provided by Bean Validation may not be suitable for multilingual applications. In this article, we will explore how to easily customize and internationalize error messages for Bean Validation in a JAX-RS application.
Understanding Bean Validation
Bean Validation (JSR 380) is a standard part of the Java EE platform that allows developers to define constraints on Java beans using annotations. These constraints include things like maximum and minimum values, regular expressions, and more. When these constraints are violated, Bean Validation automatically generates error messages.
Let's consider a simple example where we have a User
class with constraints defined using Bean Validation annotations:
public class User {
@NotNull
private String username;
@Email
private String email;
@Size(min = 6, max = 20)
private String password;
// Getters and setters
}
In this example, the @NotNull
, @Email
, and @Size
annotations define constraints for the username
, email
, and password
fields of the User
class, respectively.
When the constraints are violated, Bean Validation provides default error messages, which may not be suitable for all scenarios. Additionally, these error messages are in English by default, which may not be ideal for international applications.
Customizing Error Messages
To customize error messages, we can create a resource bundle (a properties file) for each supported language and locale. For example, we can have ValidationMessages.properties
for English and ValidationMessages_fr.properties
for French.
Let's create a ValidationMessages.properties
file to customize the error messages for our User
class:
javax.validation.constraints.NotNull.message=Username is required
javax.validation.constraints.Email.message=Invalid email address
javax.validation.constraints.Size.message=Password must be between {min} and {max} characters
In this file, we override the default error messages for the @NotNull
, @Email
, and @Size
constraints. We use the keys corresponding to the fully qualified class names of the constraint annotations followed by .message
.
Similarly, we would have ValidationMessages_fr.properties
with French error messages.
Now, we need to instruct Bean Validation to use these custom error messages. We can achieve this by configuring a message interpolator.
Configuring Message Interpolator
In a JAX-RS application, we can utilize a ConstraintViolationExceptionMapper
to provide a custom message interpolator for Bean Validation. This mapper is responsible for mapping ConstraintViolationException
to a meaningful HTTP response.
Below is a simplified version of a ConstraintViolationExceptionMapper
that configures a custom message interpolator:
@Provider
public class CustomConstraintViolationExceptionMapper implements ExceptionMapper<ConstraintViolationException> {
@Context
private HttpServletRequest request;
@Override
public Response toResponse(ConstraintViolationException exception) {
Locale locale = request.getLocale();
ResourceBundle resourceBundle = ResourceBundle.getBundle("ValidationMessages", locale);
MessageInterpolator interpolator = new ResourceBundleMessageInterpolator(new ParameterMessageInterpolator(new DefaultMessageInterpolator(), resourceBundle));
ValidatorFactory factory = Validation.byDefaultProvider().configure().messageInterpolator(interpolator).buildValidatorFactory();
Validator validator = factory.getValidator();
Set<ConstraintViolation<?>> violations = exception.getConstraintViolations();
// Process violations and build the response
// ...
return Response.status(Status.BAD_REQUEST).entity(/* error response entity */).build();
}
}
In this code, we obtain the request locale, load the appropriate resource bundle based on the locale, and create a custom message interpolator using the ResourceBundleMessageInterpolator
.
We then configure the ValidatorFactory
to use this custom message interpolator and obtain a Validator
instance. Finally, we process the constraint violations and build an appropriate error response.
By using this approach, we are able to provide custom and internationalized error messages for Bean Validation in our JAX-RS application.
The Last Word
In this article, we have explored how to customize and internationalize error messages for Bean Validation in a JAX-RS application. By leveraging resource bundles and a custom message interpolator, we can easily provide localized error messages for different languages and locales. This ensures that our API endpoints can communicate validation errors effectively, catering to a diverse user base.
Bean Validation, when integrated with JAX-RS, not only facilitates input validation but also ensures a smooth user experience by providing clear and concise error messages.
For further information on Bean Validation, check out the official documentation here. Additionally, you can learn more about JAX-RS from the Jersey documentation here.
Incorporating these practices into your JAX-RS applications will elevate the quality and user-friendliness of your APIs, setting a solid foundation for a robust and internationally accessible system.