Mastering Java 8: Filter, Map & Collect Stream Explained!
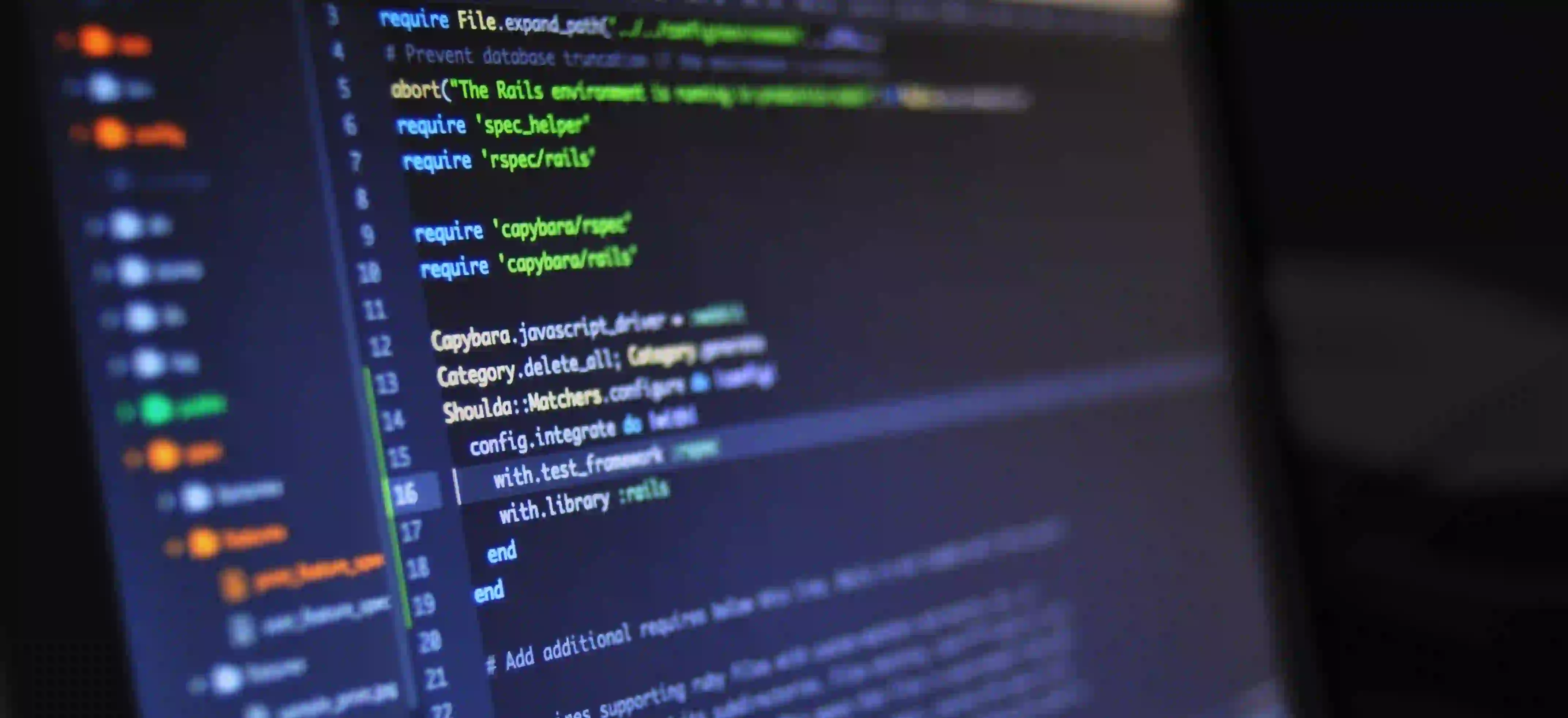
Mastering Java 8: Filter, Map & Collect Stream Explained!
Java 8 brought a multitude of new features to the table, significantly transforming the way developers write code. One of the most powerful additions is the Stream API, which provides a functional approach to processing collections of objects. In this blog post, we will delve into the concepts of filtering, mapping, and collecting streams in Java 8, highlighting their significance and demonstrating their usage through code examples.
Understanding Streams in Java 8
Streams in Java 8 represent a sequence of elements and provide a declarative approach to processing them. Thanks to their functional nature, streams allow for concise and expressive code, promoting readability and maintainability.
Filtering Streams
Filtering is a fundamental operation when working with data. In the context of streams, filtering allows us to select elements based on certain criteria. The filter
method takes a Predicate
as an argument, which determines whether an element should be included in the resulting stream. Let's take a look at a simple example to illustrate this:
List<String> languages = Arrays.asList("Java", "Kotlin", "Scala", "Python", "Ruby");
List<String> filteredLanguages = languages.stream()
.filter(lang -> lang.length() > 4)
.collect(Collectors.toList());
System.out.println(filteredLanguages); // Output: [Kotlin, Scala, Python]
In this example, we start with a list of languages and use the filter
method to only retain those with a length greater than 4 characters. The resulting stream is then collected into a list, which contains the filtered elements.
Mapping Streams
Mapping operations in streams allow for transforming elements from one form to another. The map
method is commonly used for this purpose, where a Function
is applied to each element, producing a new stream of the transformed elements. Consider the following example:
List<String> languages = Arrays.asList("Java", "Kotlin", "Scala");
List<Integer> nameLengths = languages.stream()
.map(String::length)
.collect(Collectors.toList());
System.out.println(nameLengths); // Output: [4, 6, 5]
In this case, the map
method applies the length
method of String
to each element, resulting in a stream of the lengths of the language names.
Collecting Streams
After processing a stream, it's often necessary to collect the results into a more manageable form, such as a list, set, or map. The collect
method serves this purpose by transforming the elements of the stream into a different type of result. Let's consider an example where we collect the filtered elements into a set:
List<String> languages = Arrays.asList("Java", "Kotlin", "Scala", "Python", "Ruby");
Set<String> filteredLanguagesSet = languages.stream()
.filter(lang -> lang.startsWith("J"))
.collect(Collectors.toSet());
System.out.println(filteredLanguagesSet); // Output: [Java]
In this example, the filter
method is used to retain only the languages starting with the letter "J", and the resulting elements are collected into a set using the toSet
collector.
Integration of Filtering, Mapping, and Collecting
Combining filtering, mapping, and collecting operations allows for powerful data processing pipelines. Let's consider a more comprehensive example to illustrate the integration of these operations:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Eve");
List<String> modifiedNames = names.stream()
.filter(name -> name.length() > 3)
.map(String::toUpperCase)
.collect(Collectors.toList());
System.out.println(modifiedNames); // Output: [ALICE, CHARLIE, DAVID]
In this example, we filter out names with a length less than or equal to 3 characters, then transform the remaining names to uppercase, and finally collect the results into a list.
Benefits of Stream Operations
The use of stream operations such as filtering, mapping, and collecting brings several benefits to Java developers:
-
Conciseness: Stream operations enable concise and readable code, reducing the verbosity often associated with traditional looping constructs.
-
Clarity: By expressing operations as functional transformations, streams promote clarity in code, making it easier to understand the intent of the processing.
-
Parallelism: Stream operations can seamlessly leverage parallel processing, offering potential performance gains without additional effort from the developer.
-
Reusability: The functional nature of stream operations encourages the creation of reusable code components, enhancing maintainability and extensibility.
Lessons Learned
The Stream API in Java 8 introduces a paradigm shift in the way data is processed, emphasizing functional transformations and declarative processing. By mastering the concepts of filtering, mapping, and collecting streams, developers can harness the full potential of this powerful API, leading to more expressive, maintainable, and efficient code.
In conclusion, the adoption of stream operations represents a significant advancement in modern Java development, and understanding their intricacies is paramount for any developer striving to excel in the language.
For further exploration, refer to the official Java 8 documentation and the comprehensive guide on Oracle's Stream API.
Mastering the art of processing streams unlocks a world of possibilities within Java 8 and beyond, enabling developers to craft elegant solutions to complex problems. Let the seamless combination of filtering, mapping, and collecting propel your code to new heights!