Unlocking Iframes: Boost Your Website's Functionality Now!
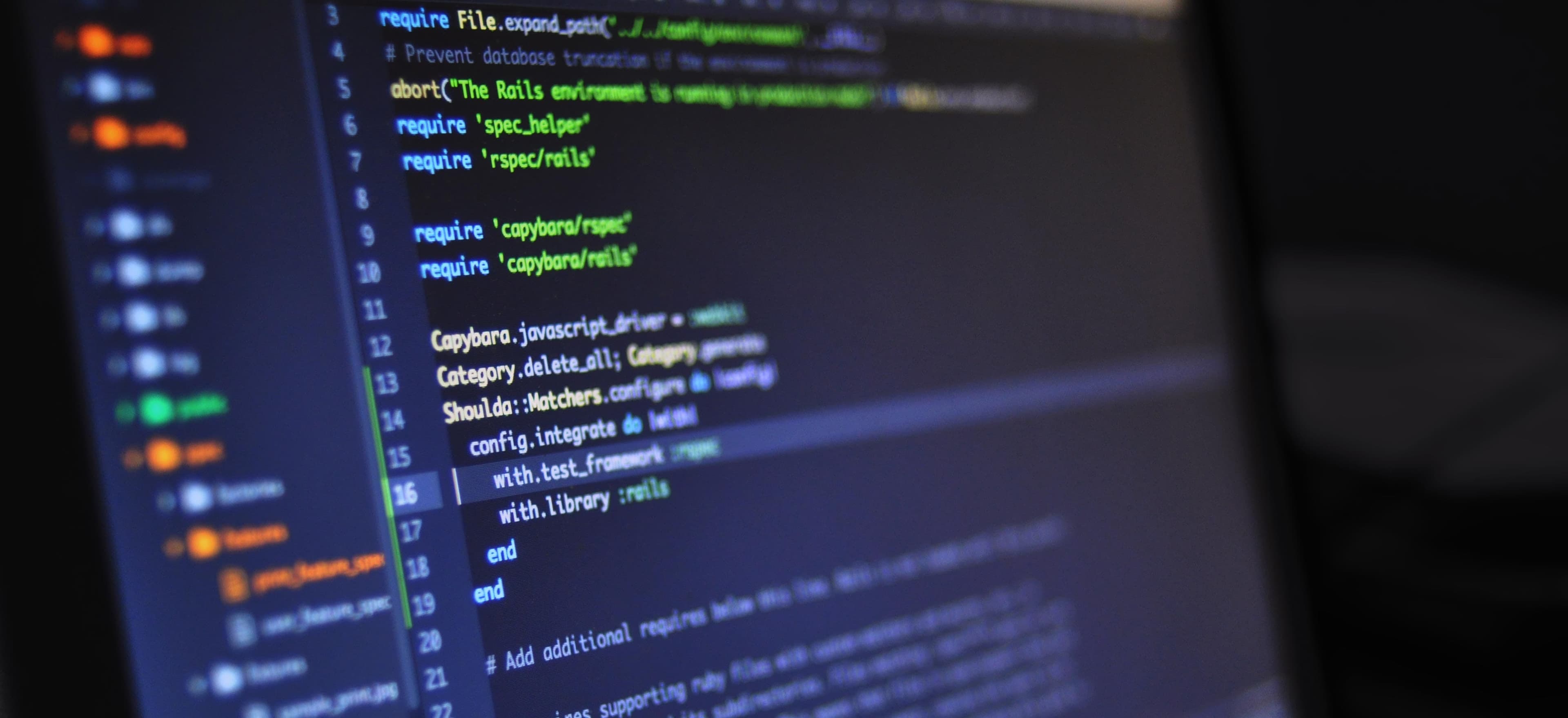
- Published on
Unlocking Iframes: Boost Your Website's Functionality Now!
Iframes, or inline frames, have been a staple of web development since the early days of the internet. They allow developers to embed external content seamlessly into their web pages, from maps and videos to interactive applications. However, iframes come with their own set of challenges, especially when it comes to styling, communication between the parent and child document, and handling events.
In this article, we will explore the potential of iframes and how to unlock their full potential using Java. We will cover common challenges, best practices, and demonstrate how Java can be used to enhance the functionality of iframes.
Understanding Iframes
Before diving into the details of using Java with iframes, let's understand what iframes are and how they are typically used in web development.
An iframe is an HTML element that allows you to embed another HTML document inside the current one. This embedded document is often hosted on a different domain or server, making iframes a powerful tool for integrating content from third-party sources.
Here's a basic example of an iframe:
<iframe src="https://example.com"></iframe>
This simple snippet creates an iframe that loads the content from https://example.com
within the parent document.
Challenges with Iframes
While iframes provide a convenient way to integrate external content, they come with their own set of challenges. Some of the common issues developers face when working with iframes include:
- Styling: Styling iframes can be tricky, especially when it comes to ensuring a consistent look and feel across different browsers and devices.
- Communication: Establishing communication between the parent document and the content inside the iframe can be complex, particularly when trying to pass data or trigger events.
- Security: Iframes can pose security risks, such as clickjacking and other forms of malicious attacks if not handled properly.
Enhancing Iframes with Java
Java provides powerful tools and libraries that can be leveraged to overcome the challenges associated with iframes. Let's explore some ways Java can enhance the functionality of iframes.
Styling Iframes
Styling iframes using Java can be achieved by accessing the iframe's content document and manipulating its styles programmatically. For example, let's say you want to dynamically set the width and height of an iframe based on the content it's loading:
import org.w3c.dom.Document;
import org.w3c.dom.Element;
public class IframeStyler {
public void setIframeSize(String iframeId, int width, int height) {
Document iframeDoc = getIframeDocument(iframeId);
if (iframeDoc != null) {
Element iframeElement = iframeDoc.getElementById(iframeId);
iframeElement.setAttribute("width", width + "px");
iframeElement.setAttribute("height", height + "px");
}
}
private Document getIframeDocument(String iframeId) {
// Code to retrieve the iframe's content document
}
}
In this example, we are using the org.w3c.dom
package to access the iframe's content document and manipulate its attributes. This allows for dynamic customization of the iframe's appearance based on the requirements of the parent document.
Communication Between Parent and Iframe
Handling communication between the parent document and the content inside the iframe is a common requirement, especially in scenarios where data needs to be shared or events need to be triggered. Java can facilitate this communication by using techniques such as postMessage.
Here's a simplified example of using postMessage to communicate between the parent and the iframe:
public class IframeCommunicator {
public void sendMessageToIframe(String iframeId, String message) {
String script = "window.frames['" + iframeId + "'].postMessage('" + message + "', '*')";
// Execute the script in the parent document
}
public void receiveMessageFromIframe() {
// Code to listen for messages from the iframe and handle them accordingly
}
}
In this example, we are using the postMessage method to send a message from the parent document to the iframe. Additionally, we can also listen for messages from the iframe and process them accordingly.
Security Considerations
When working with iframes, it's crucial to prioritize security to prevent vulnerabilities such as clickjacking. Java provides security features and best practices that can be applied to ensure the safe integration of iframes within web applications.
For instance, Java servlet filters can be used to add security headers to responses containing iframes to mitigate clickjacking attacks. By setting the X-Frame-Options header to DENY or SAMEORIGIN, you can control whether the content can be embedded in a frame on other sites.
public class FrameOptionsFilter implements Filter {
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) {
HttpServletResponse httpServletResponse = (HttpServletResponse) response;
httpServletResponse.setHeader("X-Frame-Options", "DENY");
chain.doFilter(request, response);
}
}
By incorporating such security measures within your Java web application, you can ensure that iframes are safely integrated without exposing your website to potential security threats.
Lessons Learned
In conclusion, iframes are a versatile tool for integrating external content into web applications, but they come with their own set of challenges. Java provides powerful capabilities to overcome these challenges and enhance the functionality of iframes. From dynamically styling iframes to facilitating communication and ensuring security, Java empowers developers to unlock the full potential of iframes in their web applications.
By harnessing the capabilities of Java alongside iframes, developers can enrich the user experience, expand functionality, and ensure a secure integration of external content within their web applications. Embracing Java's features for working with iframes opens up a world of possibilities for building interactive, dynamic, and powerful web applications.