Overcoming Java Classpath Resource Loading Pitfalls
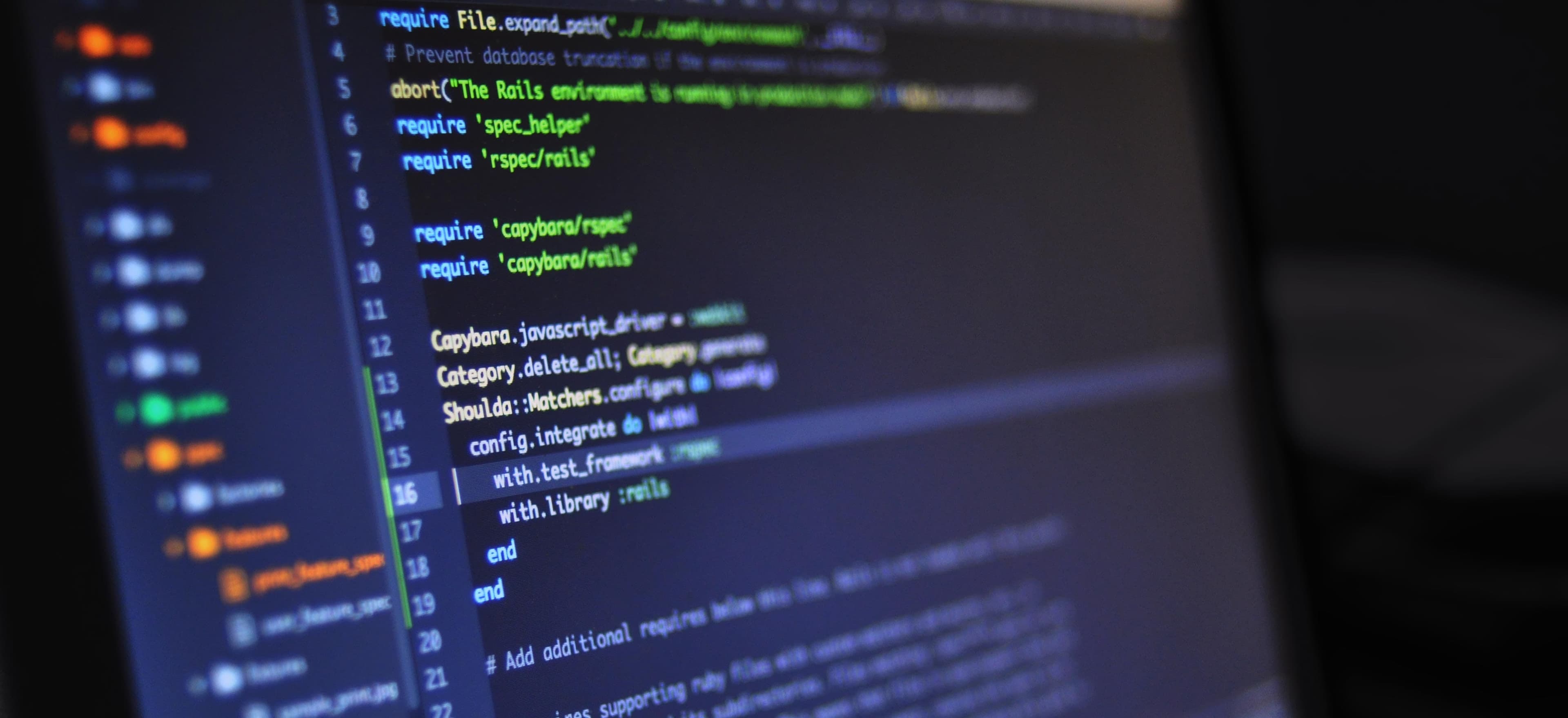
- Published on
Overcoming Java Classpath Resource Loading Pitfalls
When working with Java applications, it's common to encounter challenges related to classpath resource loading. Whether it's loading configuration files, properties, or other resources, the classpath is a critical aspect of Java application development. In this article, we'll explore common pitfalls related to classpath resource loading and how to overcome them effectively.
Understanding the Classpath
The classpath is a parameter that tells the Java Virtual Machine (JVM) where to look for user-defined classes and resources. It is crucial for locating resources such as files, images, and configuration files used by an application. When a Java application is executed, the JVM uses the classpath to locate these resources.
Pitfall 1: Using the Wrong Path
One of the common pitfalls in classpath resource loading is using the wrong path to access the resources. This often happens when developers misunderstand the structure of the classpath and provide incorrect resource paths.
For instance, consider the following incorrect resource loading code:
InputStream stream = MyClass.class.getClassLoader().getResourceAsStream("config.properties");
In this example, the developer assumes that the resource config.properties
is directly available at the root of the classpath. However, this might not be the case, leading to resource loading failures.
Solution 1: Providing Correct Resource Paths
To overcome this pitfall, it's crucial to understand the structure of the classpath and provide the correct resource path. Instead of assuming the resource location, it's better to use a more reliable approach:
InputStream stream = MyClass.class.getClassLoader().getResourceAsStream("/com/example/config.properties");
By providing the correct resource path based on the package structure, you can ensure that the resource is loaded successfully regardless of the environment.
Pitfall 2: Using the Wrong ClassLoader
Another common pitfall is using the wrong ClassLoader to load resources. When dealing with complex classloader hierarchies, it's easy to inadvertently use the wrong classloader, resulting in resource loading issues.
Consider the following erroneous resource loading attempt using the system class loader:
InputStream stream = ClassLoader.getSystemClassLoader().getResourceAsStream("config.properties");
Using the system class loader may not always be appropriate, especially in scenarios where an application is running within a complex classloader environment, such as in a Java EE application server.
Solution 2: Using the Correct ClassLoader
To ensure reliable resource loading, it's important to use the appropriate ClassLoader based on the context of the application. For example, when working with web applications, it's advisable to use the context class loader to access resources:
Thread.currentThread().getContextClassLoader().getResourceAsStream("config.properties");
By using the correct ClassLoader based on the application context, you can avoid resource loading pitfalls related to classloader hierarchy complexities.
Pitfall 3: Ignoring Resource Loading Failures
Ignoring resource loading failures is a detrimental practice that can lead to unexpected runtime errors and application instability. When developers do not handle resource loading failures effectively, the application may encounter runtime exceptions, making it challenging to diagnose and troubleshoot issues.
Consider the following example of ignoring resource loading failures:
File file = new File("config.properties");
if (file.exists()) {
// Proceed with resource loading
} else {
// Ignore the failure
}
In this scenario, if the file does not exist, the code proceeds without handling the failure, potentially leading to runtime errors later in the application.
Solution 3: Handling Resource Loading Failures
To overcome this pitfall, it's essential to handle resource loading failures gracefully. One effective approach is to use try-with-resources to ensure proper resource management and handle failures gracefully:
try (InputStream stream = MyClass.class.getClassLoader().getResourceAsStream("config.properties")) {
// Process the loaded resource
} catch (IOException e) {
// Handle the resource loading failure
}
By handling resource loading failures with appropriate exception handling, developers can ensure that the application responds gracefully to issues related to resource loading.
Final Thoughts
In conclusion, classpath resource loading is a critical aspect of Java application development, and it's essential to understand and overcome common pitfalls associated with it. By providing correct resource paths, using the appropriate ClassLoader, and handling resource loading failures effectively, developers can ensure robust and reliable resource loading in their Java applications.
Understanding the intricacies of classpath resource loading and avoiding common pitfalls can significantly enhance the stability and resilience of Java applications.
For further reading and in-depth exploration, check out the official Oracle documentation on Java Class Loaders and the Classpath: Java Class Loaders and the Classpath.
Checkout our other articles