Boost Efficiency: Mastering Java ForkJoin for Quick Tasks!
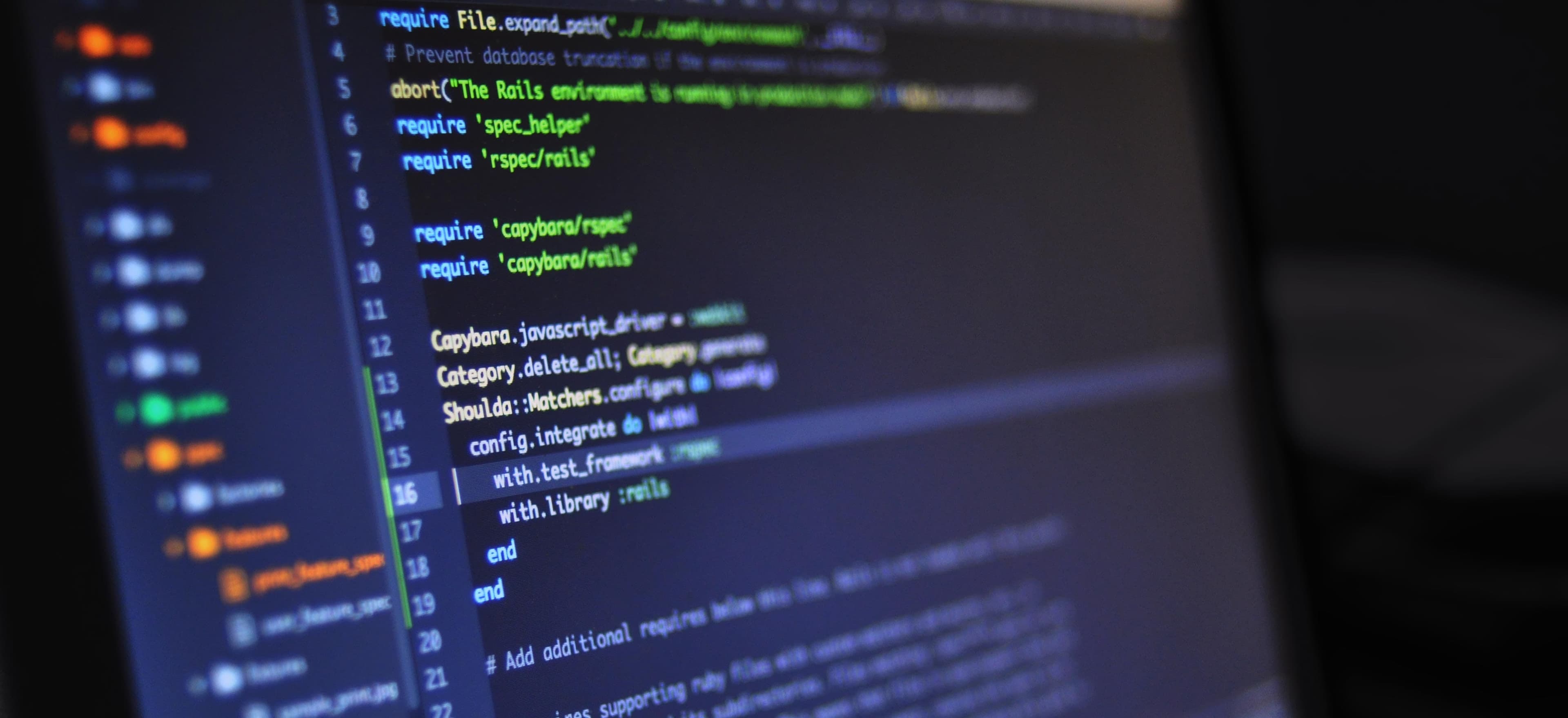
- Published on
Boost Efficiency: Mastering Java ForkJoin for Quick Tasks!
In today's fast-paced world, the demand for lightning-fast performance in Java applications is at an all-time high. As a Java developer, you constantly seek ways to maximize the efficiency of your code, especially when dealing with quick, parallelizable tasks. This is where Java's ForkJoin framework comes into play. Leveraging the power of ForkJoin in your Java projects can significantly enhance the speed and responsiveness of your applications, leading to happier users and more efficient code.
What is ForkJoin?
ForkJoin was introduced in Java 7 as a framework for parallel programming. It is designed to efficiently handle tasks that can be broken down into smaller subtasks and executed in parallel. The core concept behind ForkJoin is the "divide and conquer" approach, where a large task is split into smaller subtasks that can be processed independently. Once the smaller tasks are executed, the results are combined to produce the final outcome.
Why Use ForkJoin?
ForkJoin is particularly well-suited for scenarios where quick tasks can be divided into smaller, independent units of work that can be executed concurrently. Common use cases for ForkJoin include recursive algorithms, sorting, searching, and tasks involving matrix operations.
By harnessing the power of multiple CPU cores and executing tasks in parallel, ForkJoin can lead to significant performance improvements, especially on multi-core systems.
Getting Started with ForkJoin
To begin exploring the efficiency-boosting potential of ForkJoin, let's delve into a simple example that showcases its fundamental concepts.
Example: Parallel Summation
Suppose we want to calculate the sum of an array of integers. Traditionally, this would be done sequentially by iterating through the array and accumulating the sum. However, with ForkJoin, we can distribute the summation across multiple threads, speeding up the process.
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class ParallelSummation extends RecursiveTask<Long> {
private static final int THRESHOLD = 1000;
private int[] array;
private int start;
private int end;
public ParallelSummation(int[] array, int start, int end) {
this.array = array;
this.start = start;
this.end = end;
}
@Override
protected Long compute() {
if (end - start <= THRESHOLD) {
long sum = 0;
for (int i = start; i < end; i++) {
sum += array[i];
}
return sum;
} else {
int mid = (start + end) / 2;
ParallelSummation left = new ParallelSummation(array, start, mid);
ParallelSummation right = new ParallelSummation(array, mid, end);
left.fork();
long rightResult = right.compute();
long leftResult = left.join();
return leftResult + rightResult;
}
}
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
ForkJoinPool pool = new ForkJoinPool();
long result = pool.invoke(new ParallelSummation(array, 0, array.length));
System.out.println("Sum: " + result);
}
}
In this example, we define a ParallelSummation
class that extends RecursiveTask
. The compute
method is overridden to implement the parallel summation logic. If the size of the array to be summed is less than or equal to the THRESHOLD
, the summation is performed sequentially. Otherwise, the array is split into two halves, and the summation of each half is executed in parallel using ForkJoin's fork()
and join()
methods. The results are then combined to obtain the final sum.
By running this example, you can observe the distribution of work across multiple threads, leading to faster computation of the sum.
Benefits of ForkJoin
The example illustrates the key benefits of using ForkJoin for quick tasks:
- Parallel Execution: ForkJoin efficiently distributes subtasks across multiple threads, leveraging the full potential of multi-core systems.
- Automatic Work Stealing: The ForkJoin framework incorporates work-stealing algorithms, ensuring that idle worker threads can efficiently "steal" and execute subtasks from other threads, promoting better CPU utilization.
- Simplified Parallelism: ForkJoin abstracts away many low-level details of thread management, making it easier to parallelize tasks without delving into the complexities of thread synchronization and management.
Best Practices for Using ForkJoin
While ForkJoin presents a powerful tool for enhancing the speed and efficiency of your Java applications, it's essential to follow best practices to maximize its potential.
Understand Task Granularity
Before parallelizing a task with ForkJoin, it's crucial to analyze the granularity of the subtasks. If the tasks are too fine-grained, the overhead of task creation and management may outweigh the benefits of parallelism. On the other hand, overly coarse-grained tasks may underutilize the available CPU resources.
Use RecursiveTask and RecursiveAction Wisely
In the context of ForkJoin, the choice between RecursiveTask
and RecursiveAction
shapes the design of your parallel tasks.
- Use
RecursiveTask
when the parallel task has a return value. This is suitable for tasks like computing results or aggregating data. - Use
RecursiveAction
when the parallel task performs some operation but does not return a value. This is ideal for tasks like file processing or updating data.
Maintain Data Locality
When splitting tasks for parallel execution, aim to maintain data locality to minimize cache coherence overhead. By ensuring that related data is processed together in a task, you can reduce the impact of cache misses and optimize memory access patterns.
Monitor and Tune Concurrency Level
To achieve optimal performance with ForkJoin, it's essential to monitor the concurrency level and tune it according to the characteristics of the tasks and the underlying hardware. Adjusting the parallelism level can prevent oversubscription or underutilization of resources.
Final Thoughts
In the quest for optimal application performance, mastering Java's ForkJoin framework is a valuable asset. By harnessing parallelism and efficient task management, ForkJoin empowers developers to unlock the full potential of multi-core systems, leading to faster execution and improved responsiveness in their Java applications. As you explore ForkJoin further, keep in mind the best practices and considerations discussed to leverage its capabilities effectively and elevate the efficiency of your code.
Experience the power of ForkJoin and witness the transformation of your Java applications into efficient, high-performance solutions!
To dive deeper into ForkJoin and its applications, consider exploring the official documentation and experimenting with more complex parallel tasks. Happy coding!
Checkout our other articles