Boost Clojure IO: Manage Large Files with Ease!
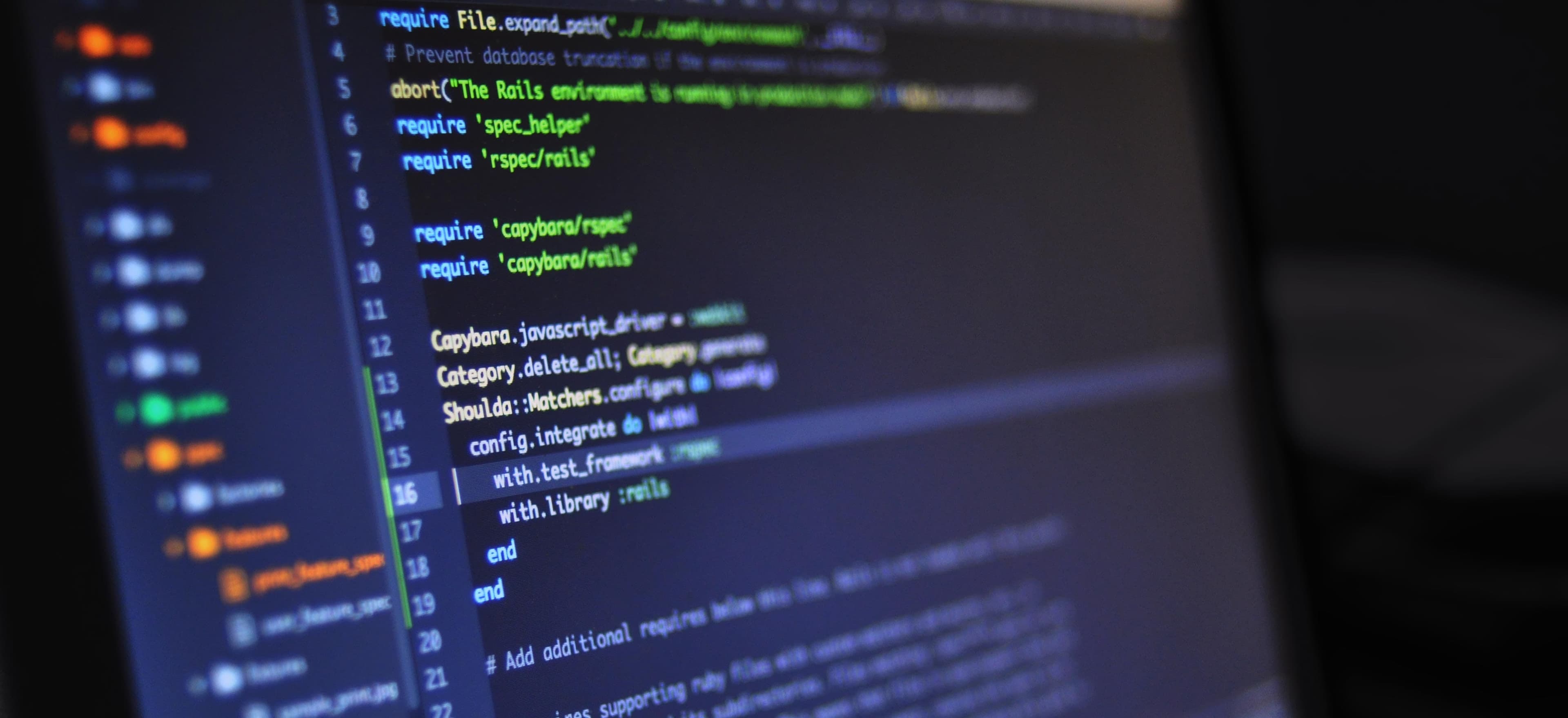
- Published on
Managing Large Files with Clojure IO
Clojure is a powerful and expressive language that runs on the Java Virtual Machine (JVM). One of the key features of Clojure is its robust support for handling I/O operations, including managing large files. In this blog post, we’ll explore some best practices and techniques for efficiently working with large files in Clojure using its I/O library.
Java Interoperability
Clojure seamlessly interoperates with Java, which allows developers to leverage the vast ecosystem of Java libraries. When dealing with large files, this interoperability becomes particularly valuable, as we can tap into the high-performance I/O capabilities of Java.
Reading Large Files
When tasked with reading a large file, traditional approaches using read-line
or slurp
might not be ideal, especially if the file size exceeds the available memory. Clojure provides a more efficient way to handle this using Java’s BufferedReader
for reading large files line by line.
(require '[clojure.java.io :as io])
(defn read-large-file [file-path]
(with-open [reader (io/reader (io/file file-path))]
(doseq [line (line-seq reader)]
(process-line line))))
In this example, we use io/reader
to create a reader for the file, and then line-seq
to iterate over the lines. The with-open
macro ensures that the reader is closed after processing the file, preventing resource leaks.
Writing Large Files
Similarly, when writing large amounts of data to a file, the use of a buffered writer can significantly improve performance. Clojure provides the PrintWriter
class from Java for this purpose.
(defn write-large-file [file-path data]
(with-open [writer (io/writer (io/file file-path))]
(doseq [line data]
(.println writer line)))
In this function, we create a writer using io/writer
and then use doseq
to iterate over the data, writing each line to the file using the PrintWriter
instance.
Memory-Mapped Files
For scenarios where random access to large files is required, Clojure’s Java interoperability allows us to take advantage of memory-mapped files using java.nio
package. Memory-mapped files offer a way to map a file into memory, providing direct access to its contents.
(defn process-large-file [file-path]
(let [fc (java.nio.channels.FileChannel/open (java.nio.file.Paths/get file-path))
buffer (.map fc java.nio.channels.FileChannel$MapMode/READ_ONLY 0 (.size fc))]
(doseq [byte (byte-array (.capacity buffer))]
(process-byte byte))))
In this example, we open a FileChannel
for the file, and then create a direct byte buffer by mapping the file with FileChannel$MapMode/READ_ONLY
. This buffer can then be efficiently iterated over for processing large files.
Parallel File Processing
When dealing with truly massive files, processing them in parallel can lead to significant performance improvements. Clojure’s pmap
function allows for easy parallel processing of sequences, which can be applied to large file processing as well.
(defn parallel-process-large-file [file-path]
(let [lines (-> file-path io/file io/reader line-seq)]
(pmap process-line lines)))
In this function, we use the ->
threading macro to thread the file path through the io/file
, io/reader
, and line-seq
functions, obtaining a sequence of lines. We then use pmap
to process the lines in parallel, potentially leveraging multiple processor cores for faster processing.
Closing the Chapter
Clojure’s rich interoperability with Java provides a solid foundation for efficiently managing large files. By leveraging Java’s I/O capabilities and incorporating Clojure’s functional programming features, developers can tackle the challenges posed by large file processing with confidence and ease.
By following the best practices outlined in this post, you can ensure that your Clojure applications gracefully handle large files, while delivering optimal performance and resource efficiency.
To delve deeper into Clojure I/O and file handling, check out the official documentation and Java NIO package. Happy coding in Clojure!
Checkout our other articles