Master Jest: The Art of Testing Promise Rejections
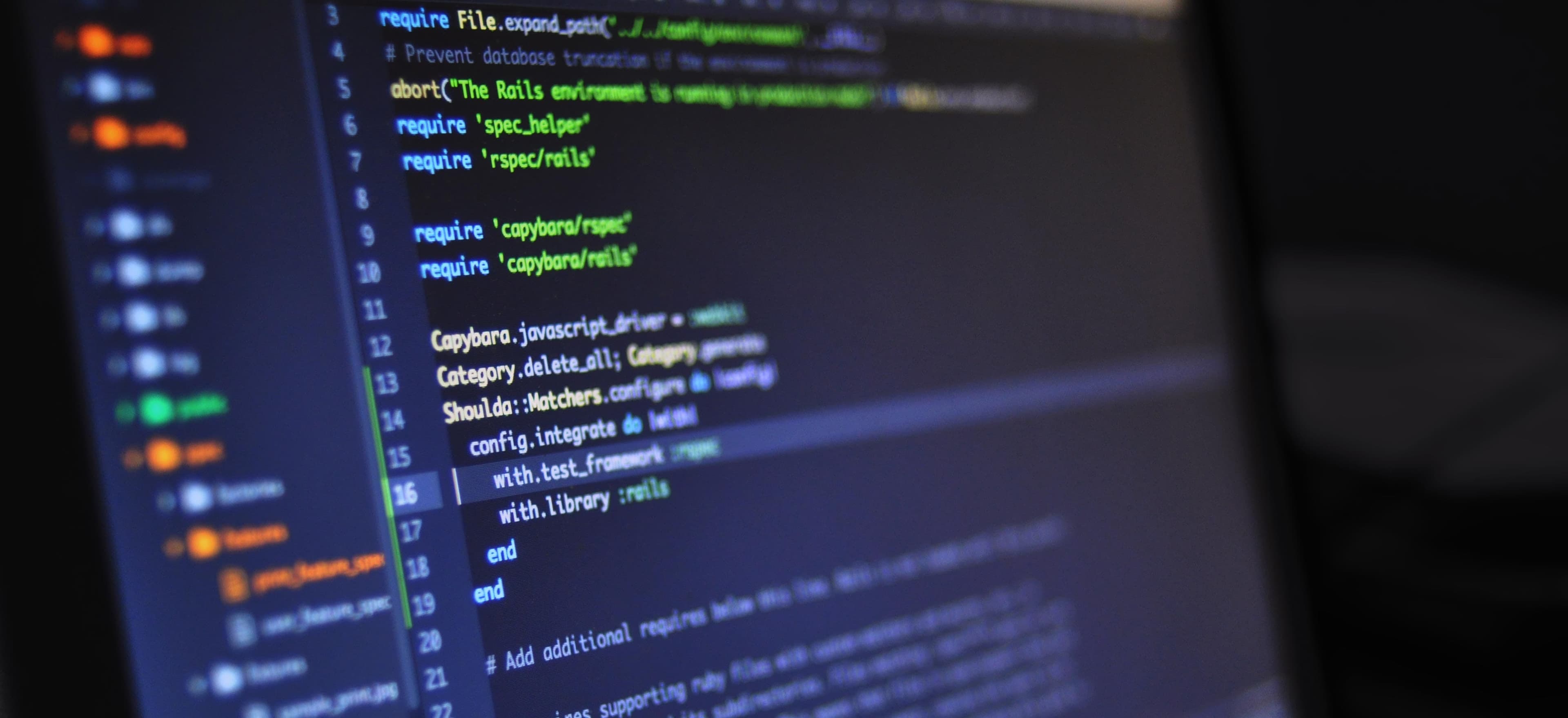
- Published on
Master Jest: The Art of Testing Promise Rejections
Testing asynchronous code is an essential part of ensuring the reliability and correctness of your applications. In JavaScript, promises are a common choice for managing asynchronous operations, and testing promise rejections is a crucial part of ensuring the robustness of your code. In this post, we will dive deep into how to effectively test promise rejections using Jest, a popular testing framework for JavaScript.
Understanding Promise Rejections
Before we delve into testing promise rejections, it's imperative to have a solid understanding of what a promise rejection is. In JavaScript, promises represent operations that produce a single value at some point in the future, be it a resolved value or a reason why it couldn't be resolved. A promise can be in one of three states: pending, fulfilled, or rejected. When a promise is rejected, it means that the operation it represents has failed, and it emits a reason for the failure.
Setting up Jest
To get started with testing promise rejections, you'll need to have Jest installed in your project. If you haven't already set up Jest, you can do so by installing it via npm:
npm install --save-dev jest
Once Jest is set up, you can proceed with writing tests for your asynchronous code.
Testing Promise Rejections with Jest
Jest provides powerful and intuitive ways to test promise rejections. Let's consider a hypothetical asynchronous function that fetches user data. When the user data is not found, the function should reject with an error message. Here's how we can test this scenario using Jest:
// asyncFunction.js
async function fetchUserData(userId) {
return new Promise((resolve, reject) => {
// Assume that the user data is fetched asynchronously
setTimeout(() => {
if (userId) {
resolve({ id: userId, name: 'John Doe' });
} else {
reject(new Error('User not found'));
}
}, 1000);
});
}
Now, let's write a test case using Jest to verify that the promise rejection is handled correctly:
// asyncFunction.test.js
const fetchUserData = require('./asyncFunction');
test('fetchUserData rejects with an error message for invalid userId', async () => {
expect.assertions(1);
try {
await fetchUserData(null);
} catch (error) {
expect(error.message).toBe('User not found');
}
});
In this test case, we use the expect.assertions
method to ensure that a certain number of assertions are called within the test. This is important for verifying that the promise rejection is properly handled. We then use a try...catch
block to await the fetchUserData
function and catch the rejection. Finally, we use Jest's expect
to assert that the error message matches the expected message.
Testing Promise Rejections with Matchers
Jest provides an array of matchers that can be used to test values in different ways. When testing promise rejections, we can leverage the rejects
matcher to assert that a promise is rejected. Here's how we can refactor the previous test case using the rejects
matcher:
// asyncFunction.test.js
const fetchUserData = require('./asyncFunction');
test('fetchUserData rejects with an error message for invalid userId', () => {
return expect(fetchUserData(null)).rejects.toThrow('User not found');
});
In this example, we use the expect
function to assert that fetchUserData(null)
rejects with an error message that matches the expected message using the rejects.toThrow
matcher. This approach provides a more concise and readable way to test promise rejections in Jest.
Key Takeaways
Testing promise rejections is a fundamental aspect of writing robust and reliable JavaScript code. With Jest, you have a powerful tool at your disposal for effectively testing promise rejections in your asynchronous code. By understanding the nuances of promise rejections and leveraging Jest's testing capabilities, you can ensure that your asynchronous code behaves as expected, even when facing errors. Happy testing!
In conclusion, mastering the art of testing promise rejections in Jest will enhance the reliability and robustness of your JavaScript applications. By leveraging the techniques and matchers provided by Jest, you can confidently handle and validate promise rejections in your asynchronous code. Asynchronous operations are essential in modern web development, and thorough testing is key to delivering high-quality, error-resilient applications.
As you continue to strengthen your JavaScript skills, remember the significance of testing promise rejections and the valuable role Jest plays in this process. Embracing best practices and robust testing methodologies will elevate the quality and reliability of your codebase, leading to more resilient and dependable applications.
Now that you have delved into the art of testing promise rejections in Jest, integrating these techniques into your testing suite will undoubtedly contribute to the overall excellence of your JavaScript projects.
Test wisely, and code confidently with Jest!
Remember, error handling is an inherent part of software development, and mastering the testing of promise rejections is a powerful skill to acquire in your journey as a JavaScript developer.
Start implementing these concepts in your codebase and witness the reinforced reliability and robustness of your applications. Happy coding and testing!
In conclusion, mastering the art of testing promise rejections in Jest will enhance the reliability and robustness of your JavaScript applications. By leveraging the techniques and matchers provided by Jest, you can confidently handle and validate promise rejections in your asynchronous code. Asynchronous operations are essential in modern web development, and thorough testing is key to delivering high-quality, error-resilient applications.
As you continue to strengthen your JavaScript skills, remember the significance of testing promise rejections and the valuable role Jest plays in this process. Embracing best practices and robust testing methodologies will elevate the quality and reliability of your codebase, leading to more resilient and dependable applications.
Now that you have delved into the art of testing promise rejections in Jest, integrating these techniques into your testing suite will undoubtedly contribute to the overall excellence of your JavaScript projects.
Test wisely, and code confidently with Jest!
Remember, error handling is an inherent part of software development, and mastering the testing of promise rejections is a powerful skill to acquire in your journey as a JavaScript developer.
Start implementing these concepts in your codebase and witness the reinforced reliability and robustness of your applications. Happy coding and testing!
Checkout our other articles