Turn Java Code into Web Services: A Step-by-Step Guide
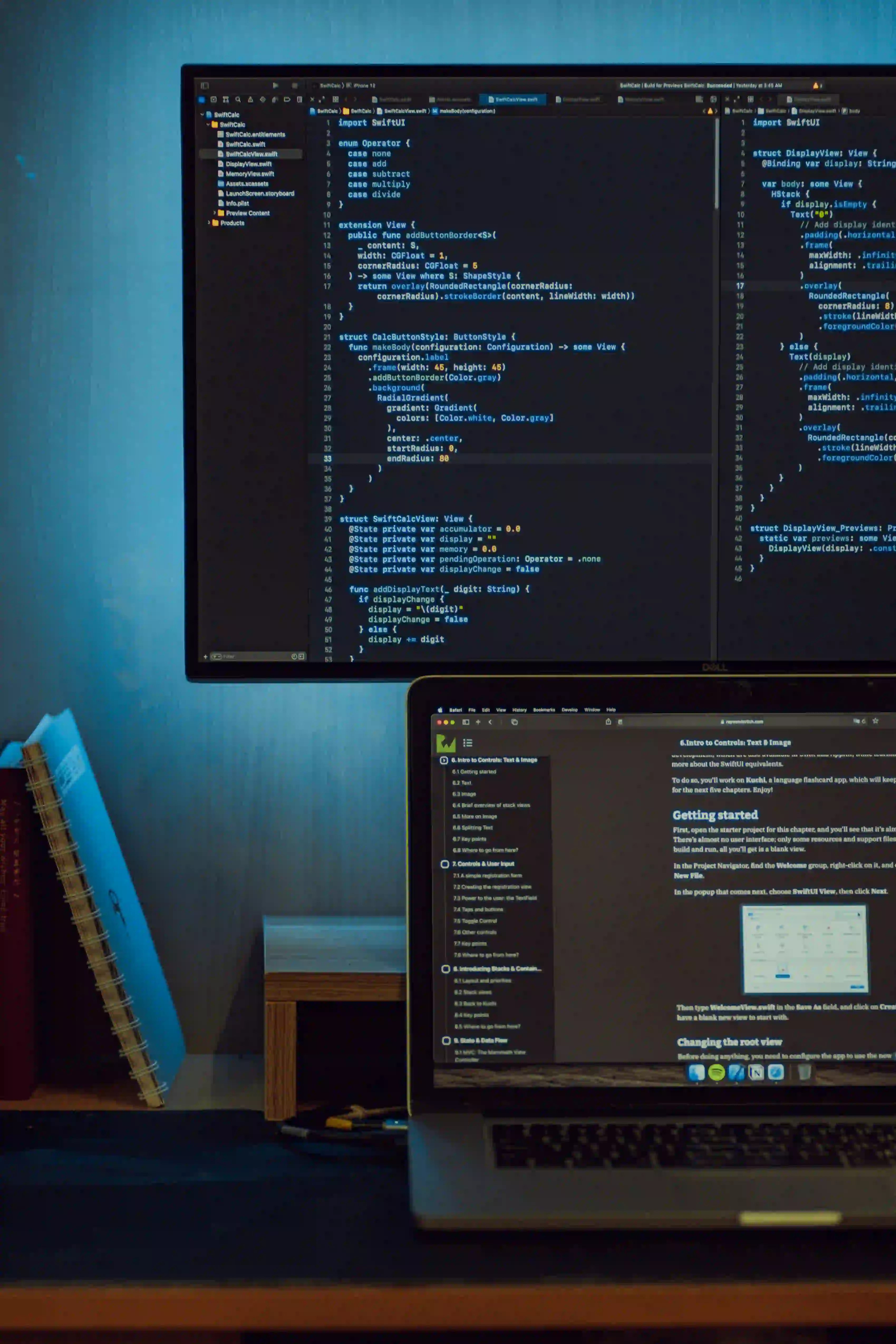
Turning Java Code into Web Services: A Step-by-Step Guide
In the world of software development, turning Java code into web services is a common task. Web services allow different applications to communicate with each other over the internet, providing a powerful means of integrating disparate systems. In this guide, we'll walk through the process of turning Java code into web services, using the popular Spring Boot framework.
What are Web Services?
Web services are software systems designed to support interoperable machine-to-machine interaction over a network. They provide a standard means of communication between different software applications, running on a variety of platforms and/or frameworks. Web services facilitate seamless integration, automating the exchange of data and enabling interactions between different systems.
Why Use Java for Web Services?
Java is a popular choice for building web services due to its robustness, platform independence, and strong community support. Additionally, Java's mature ecosystem offers a wealth of libraries and frameworks specifically designed for creating web services.
Setting Up the Project
To begin, let's set up a new project using Spring Boot, a widely used framework for building web applications and web services in Java.
Step 1: Create a New Spring Boot Project
You can use a build tool like Maven or Gradle to create a new Spring Boot project. For this guide, we'll use Maven.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Step 2: Create a Simple Java Class
Let's create a simple Java class that we'll expose as a web service. For this example, we'll create a GreetingsService
class that provides a method to return a greeting message.
public class GreetingsService {
public String getGreeting() {
return "Hello, World!";
}
}
Step 3: Expose the Java Class as a Web Service
Now, let's use Spring Boot to expose the GreetingsService
class as a web service. We'll create a new controller class, which will handle incoming web requests and map them to the appropriate methods in our GreetingsService
class.
@RestController
public class GreetingsController {
private GreetingsService greetingsService;
@Autowired
public GreetingsController(GreetingsService greetingsService) {
this.greetingsService = greetingsService;
}
@GetMapping("/greeting")
public String getGreeting() {
return greetingsService.getGreeting();
}
}
In this code snippet, we use the @RestController
annotation to mark the class as a controller. We also use the @Autowired
annotation to inject an instance of GreetingsService
into the controller. The @GetMapping
annotation specifies that the getGreeting
method should be called when a GET request is made to the /greeting
endpoint.
Running the Application
With the project set up and the web service implemented, we can now run the application to see our web service in action.
Step 1: Run the Spring Boot Application
You can run the Spring Boot application using the Maven plugin:
mvn spring-boot:run
Step 2: Test the Web Service
Once the application is running, you can test the web service by making a GET request to the /greeting
endpoint using a tool like Postman or simply accessing the URL in a web browser. You should see the greeting message "Hello, World!" returned as the response.
Wrapping Up
In this guide, we've covered the basics of turning Java code into web services using the Spring Boot framework. We've created a simple Java class and exposed it as a web service, demonstrating the power and simplicity of building web services with Java. With further exploration and practice, you can extend this knowledge to build more complex and robust web services to meet your specific requirements.
Now, armed with the knowledge of turning Java code into web services, you can contribute to the world of seamless integrations and efficient data exchange between different software applications. So, roll up your sleeves, dive into the realm of web services with Java, and unleash the power of interconnected systems!
For further reading on web services and Spring Boot, you can explore the official Spring Boot documentation and the comprehensive Java Web Services tutorial by Tutorialspoint.
Happy coding!