Cracking the Code: Simplifying PlantUML Diagrams
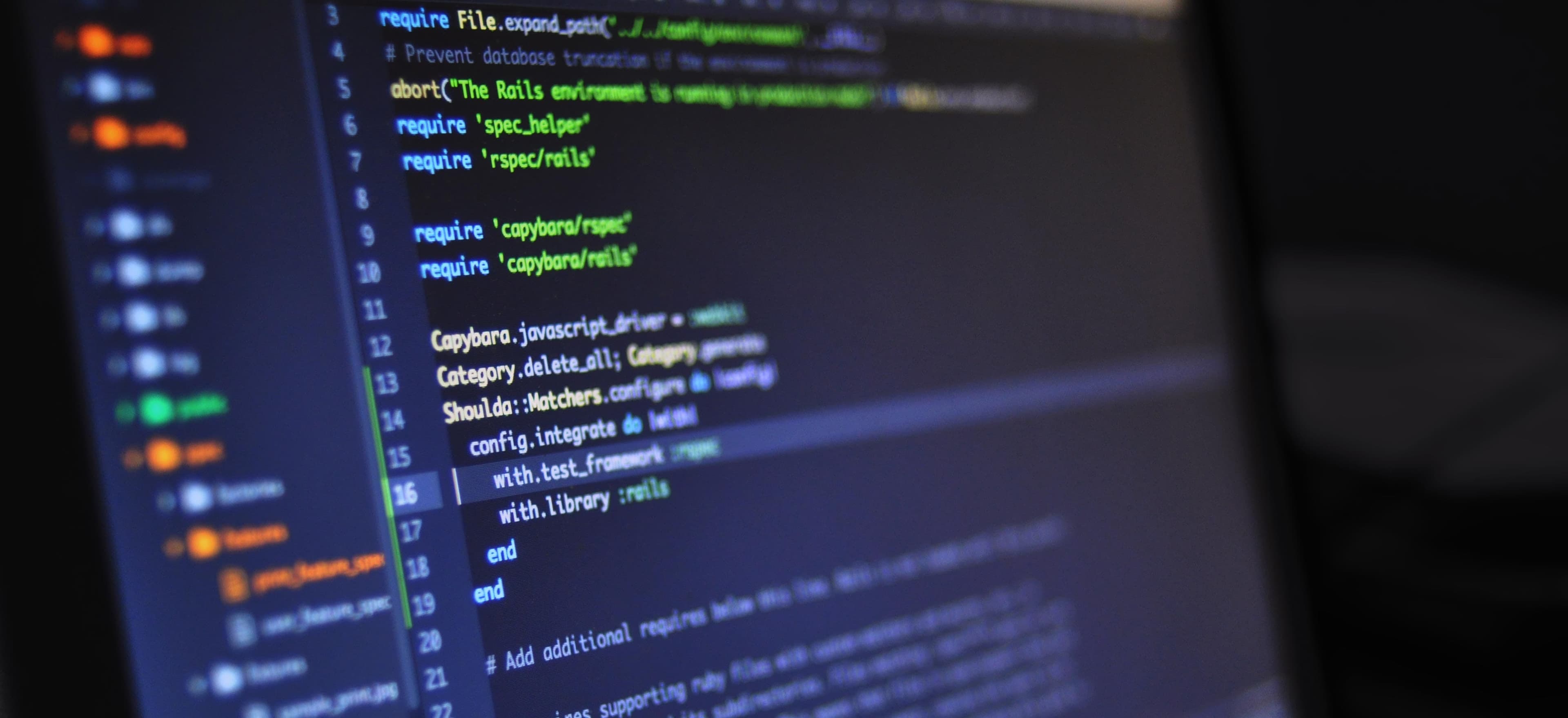
- Published on
Cracking the Code: Simplifying PlantUML Diagrams
When it comes to visualizing and documenting software systems, creating clear and concise diagrams is essential. PlantUML is a popular open-source tool that uses simple textual descriptions to generate various types of UML diagrams. While PlantUML offers immense flexibility, creating complex diagrams can sometimes lead to large, unwieldy code. In this blog post, we'll explore techniques to simplify PlantUML diagrams, making them easier to understand and maintain.
1. Using Macros to Encapsulate Repeated Elements
One common issue when working with PlantUML is the repetition of code to create similar elements across different parts of a diagram. In such cases, macros can be incredibly useful. Let's consider the following example:
!define RECTANGLE(x, y, label)
rectangle "x, y" as label
!enddefine
RECTANGLE(100, 50, "Example 1")
RECTANGLE(200, 150, "Example 2")
By using the !define
and !enddefine
directives, we can create a macro called RECTANGLE
that takes x
and y
coordinates along with a label
. This way, we can easily reuse the macro to create multiple rectangles without duplicating the code every time.
2. Grouping Related Components with the Package Keyword
In complex diagrams, it's crucial to maintain a clear structure and organization. The package
keyword in PlantUML allows us to group related components together visually. Consider the following example:
package "Main Components" {
class MainClass
class SubClass1
class SubClass2
}
package "Helper Components" {
class HelperClass1
class HelperClass2
}
By using the package
keyword, we can clearly denote the main components and helper components, making the diagram more structured and easier to comprehend.
3. Utilizing Skin Parameters for Consistent Styling
Consistency in styling is essential for ensuring that diagrams are both visually appealing and easily understandable. PlantUML provides the skinparam
directive, which allows us to customize various visual aspects of the diagrams. Consider the following example:
skinparam rectangle {
BorderColor black
BackgroundColor #FFA07A
FontStyle bold
}
In this example, we use skinparam
to set the border color, background color, and font style for rectangles. By utilizing skinparam
consistently throughout the diagram, we can ensure a cohesive and professional look.
4. Abstraction with Interfaces and Abstract Classes
When representing complex systems, it's vital to abstract away implementation details and focus on high-level concepts. PlantUML provides support for interfaces and abstract classes, allowing us to denote abstraction in our diagrams. Consider the following example:
interface Shape {
void draw()
}
abstract class AbstractShape {
int x
int y
abstract void draw()
}
By using interfaces and abstract classes, we can clearly convey the concept of abstraction and high-level relationships within the diagram.
5. Using Comments Effectively for Clarity
In complex diagrams, adding comments can greatly enhance the understandability of the diagram. PlantUML supports both single-line and multi-line comments that can be used to provide additional context and explanations. Consider the following example:
class Example {
// This is an example class
int data
/*
This method performs a specific operation
@param param the input parameter
*/
void performOperation(int param)
}
By leveraging comments effectively, we can provide insights into the purpose and functionality of various diagram elements.
The Closing Argument
Simplifying PlantUML diagrams not only makes them easier to understand but also contributes to the overall maintainability of the diagrams. By using macros, grouping components, maintaining consistent styling, abstracting concepts, and leveraging comments, we can create diagrams that effectively convey the intended information without unnecessary complexity.
Incorporating these techniques into your PlantUML workflows can streamline the diagram creation process and ensure that the resulting diagrams are clear, organized, and easy to work with.
For further exploration, you can dive into the official PlantUML documentation to discover advanced features and best practices for diagram creation.
By implementing these strategies, you can crack the code of simplifying PlantUML diagrams, making your software visualization and documentation tasks more efficient and effective.
Checkout our other articles