Spring Secrets: Inject Values Into Config Beans Easily!
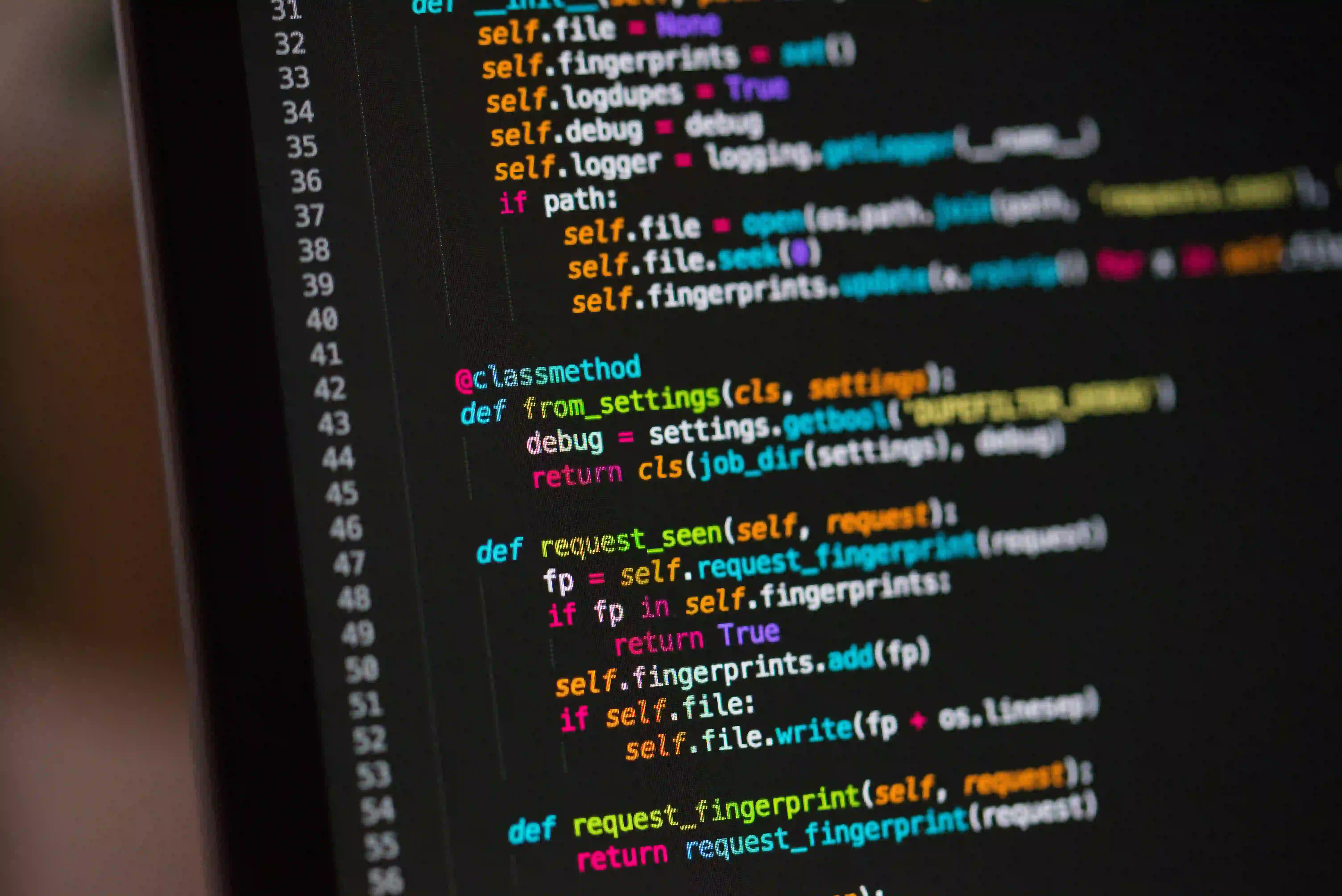
Spring Secrets: Inject Values Into Config Beans Easily!
In the world of software development, particularly in the Java ecosystem, the Spring Framework has established itself as a robust and versatile tool for building enterprise-level applications. One of the key features that makes Spring so powerful is its ability to easily manage and inject configuration values into beans.
In this blog post, we'll explore the various techniques and best practices for injecting values into Spring configuration beans, with a focus on simplicity, flexibility, and maintainability.
Why Configuration Injection Matters
Configuration is an essential aspect of any software application. It allows developers to externalize parameters, such as database connection details, service endpoints, feature toggles, and other settings. Externalizing configuration values is crucial for separating the configuration from the code, making it easier to change and manage without requiring code modifications.
In the context of Spring applications, configuration values are often injected into beans using various approaches provided by the framework. This not only ensures that the configuration is decoupled from the business logic but also enables easy management and testing of different configurations for various environments.
Property Injection
Property injection is one of the fundamental techniques for injecting configuration values into Spring beans. It allows you to externalize configuration into properties files and inject those values into beans at runtime. Let's take a look at how this can be achieved.
Step 1: Define Properties File
First, create a properties file, such as application.properties
or application.yml
, and define the configuration properties:
# application.yml
app:
name: "MyApp"
version: "1.0.0"
max-connections: 100
Step 2: Inject Properties Into Beans
Next, we can inject these properties into a Spring bean using the @Value
annotation:
@Component
public class MyAppConfiguration {
@Value("${app.name}")
private String appName;
@Value("${app.version}")
private String appVersion;
@Value("${app.max-connections}")
private int maxConnections;
// Getters and setters
}
In this example, the @Value
annotation is used to inject the values from the properties file into the MyAppConfiguration
bean.
Step 3: Use the Configuration Bean
Now, the MyAppConfiguration
bean can be used throughout the application, and the injected properties can be accessed as ordinary bean properties.
Property injection provides a straightforward way to externalize configuration values and inject them into beans, making it an essential technique in the Spring developer's toolkit.
Environment-Specific Configuration
In real-world scenarios, applications often need different configurations for various environments, such as development, testing, staging, and production. Spring provides robust support for managing environment-specific configuration through profiles.
Using Profiles for Environment-Specific Configurations
With Spring profiles, you can define different sets of configuration for each environment and activate the appropriate profile at runtime. Let's see how this can be achieved.
Step 1: Define Environment-Specific Properties
Create separate properties files for each environment, such as application-dev.properties
and application-prod.properties
, and define the environment-specific configuration properties in each file.
Step 2: Activate Profiles
In the main configuration file, such as application.yml
, you can activate profiles using the spring.profiles.active
property:
spring:
profiles:
active: dev
By activating the dev
profile, the application will load the configuration properties from application-dev.properties
.
Step 3: Inject Environment-Specific Properties
You can inject environment-specific properties into beans as previously demonstrated using the @Value
annotation.
Using profiles, Spring makes it easy to manage and load environment-specific configuration, allowing for seamless deployment across different environments.
Using @ConfigurationProperties
While @Value
provides a flexible way to inject individual properties, it may not be the most convenient approach for injecting large sets of related properties. This is where the @ConfigurationProperties
annotation shines.
Step 1: Create Configuration Properties Class
Define a POJO (Plain Old Java Object) that corresponds to the set of configuration properties:
@Configuration
@ConfigurationProperties(prefix = "app")
public class AppConfig {
private String name;
private String version;
private int maxConnections;
// Getters and setters
}
Step 2: Define Properties in Properties File
In the properties file, define the properties with the specified prefix:
# application.yml
app:
name: "MyApp"
version: "1.0.0"
max-connections: 100
Step 3: Use the Configuration Properties
Now, the AppConfig
bean can be autowired and used throughout the application, providing a more structured and type-safe way to access configuration properties.
The @ConfigurationProperties
annotation allows for easy groupings of related configuration properties, resulting in cleaner and more maintainable code.
The Last Word
In this blog post, we've delved into the various techniques for injecting configuration values into Spring beans. From the basic property injection using @Value
to the more structured approach with @ConfigurationProperties
, Spring provides an array of options for managing and injecting configuration.
By externalizing configuration values and utilizing Spring's powerful injection mechanisms, developers can build flexible and easily maintainable applications that cater to different environments and deployment scenarios.
Mastering the art of configuration injection in Spring is crucial for achieving robust and adaptable software systems, and with the knowledge gained from this post, you're well on your way to unleashing the full potential of Spring's configuration management capabilities. Happy coding!
Remember to check out the official Spring documentation for a comprehensive guide on bean configuration and dependency injection.