Java vs. JavaScript: Clarifying Confusions Once and For All
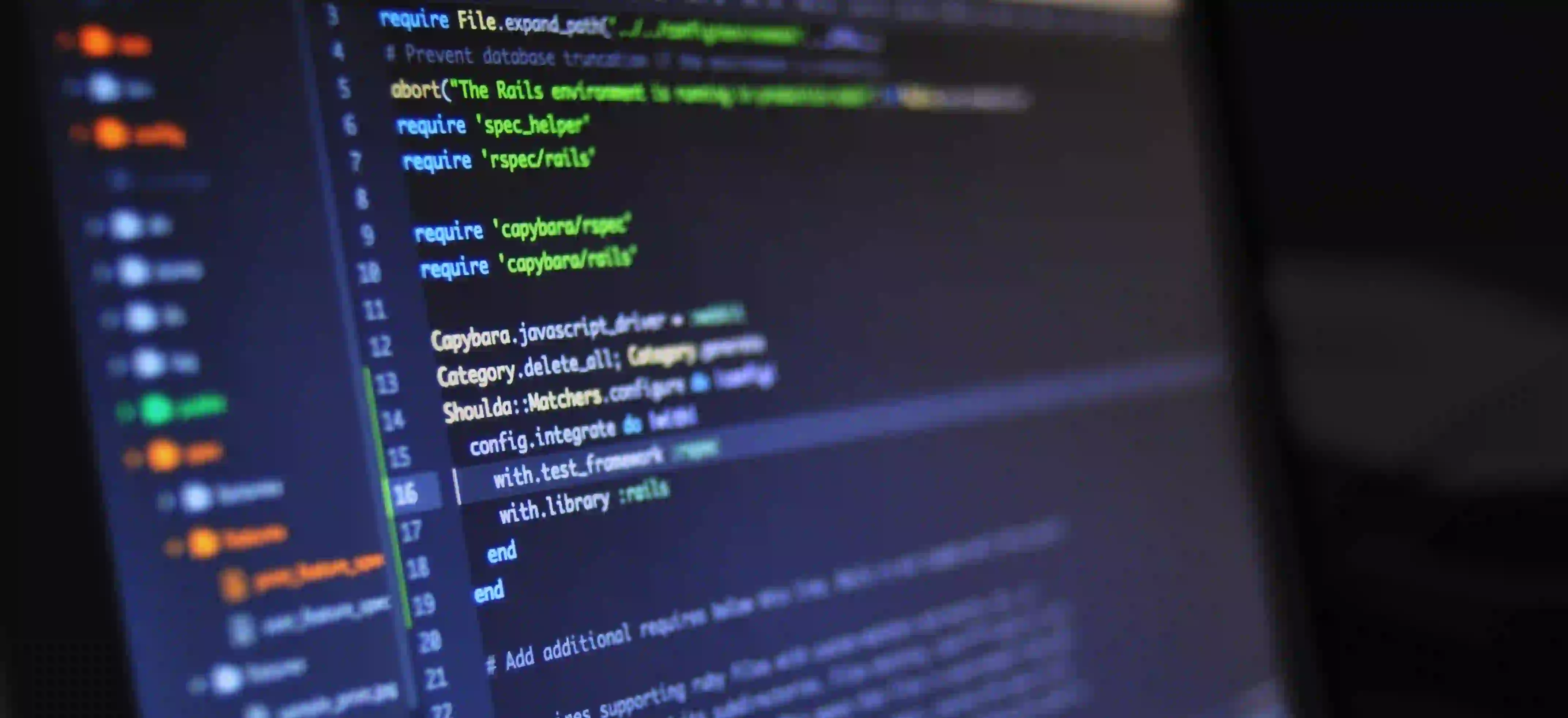
Java vs. JavaScript: Clarifying Confusions Once and For All
Java and JavaScript are two popular programming languages that often cause confusion due to their similar names. Despite the resemblance, Java and JavaScript are fundamentally different languages with distinct use cases and features. In this post, we will delve into the specifics of both languages, highlighting their differences and use cases to dispel any lingering confusion.
Java: A Powerhouse for Backend Development
Java, originally developed by Sun Microsystems (now owned by Oracle), is a high-level, class-based, object-oriented programming language. Java is renowned for its "write once, run anywhere" mantra, meaning that Java code can run on any device that supports Java without the need for recompilation. This versatility makes Java an ideal choice for developing applications that are intended to run on various platforms.
Why Use Java?
- Platform Independence: Java's platform independence is one of its defining features. The ability to run the same Java code on any platform without modification is a compelling reason to choose Java for backend development.
- Scalability and Performance: Java's robustness and scalability make it well-suited for building enterprise-level applications that demand high performance.
- Rich Ecosystem: With a vast array of libraries, frameworks, and tools, Java offers developers a rich ecosystem to leverage for various development needs.
Sample Java Code:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In this simple Java program, the main
method serves as the entry point to the application, and the System.out.println
statement is used to print "Hello, World!" to the console.
JavaScript: The Language of the Web
Unlike Java, JavaScript is primarily used for front-end web development. JavaScript is a dynamic, prototype-based scripting language that is executed in the browser. With the rise of single-page applications and interactive web interfaces, JavaScript has become an indispensable part of web development.
Why Use JavaScript?
- Client-Side Interactivity: JavaScript enables the creation of dynamic and interactive user experiences within web pages, making it an essential tool for front-end development.
- Asynchronous Programming: With features like callbacks and promises, JavaScript is well-suited for handling asynchronous operations, such as fetching data from a server without blocking the main thread.
- Node.js: In addition to front-end development, JavaScript is now used for server-side development through platforms like Node.js, further expanding its versatility.
Sample JavaScript Code:
function greet(name) {
return `Hello, ${name}!`;
}
console.log(greet('World'));
In this JavaScript example, the greet
function accepts a name
parameter and returns a greeting message. The console.log
statement is used to output the result to the browser's console.
Clarifying the Differences
Now that we've explored the individual strengths of Java and JavaScript, let's pinpoint the key distinctions between the two languages.
Execution Environment
- Java: Java applications are typically run on a Java Virtual Machine (JVM), which abstracts the underlying hardware and operating system, providing the promised platform independence.
- JavaScript: JavaScript code is executed by a web browser's JavaScript engine, allowing for dynamic manipulation of web page content and behavior.
Typing and Syntax
- Java: Java is a statically-typed language, meaning that variable types must be explicitly declared and checked at compile time. It follows a syntax similar to C and C++.
- JavaScript: JavaScript is dynamically typed, allowing variables to hold values of any type without the need for explicit type declarations. Its syntax is influenced by C and Java but exhibits significant differences in usage.
Use Cases
- Java: Backend development, enterprise applications, Android app development.
- JavaScript: Front-end web development, single-page applications, server-side development with Node.js.
Final Considerations
In conclusion, while Java and JavaScript share a portion of their names, their purposes and areas of application are vastly divergent. Java excels in backend development and enterprise-level applications, leveraging its platform independence and robust performance. On the other hand, JavaScript dominates the realm of front-end web development, offering dynamic interactivity and asynchronous capabilities. Understanding the distinctions between Java and JavaScript is crucial for selecting the appropriate language based on the requirements of a given project.
By shedding light on the differences between Java and JavaScript, we hope to have cleared up any ambiguities and provided a clear understanding of the unique domains in which each language operates. Whether it's developing a scalable enterprise application with Java or crafting an interactive web interface with JavaScript, both languages have their own strengths and niches in the world of software development.