Avoiding Spring's Trap: Perfecting Transactional Tests
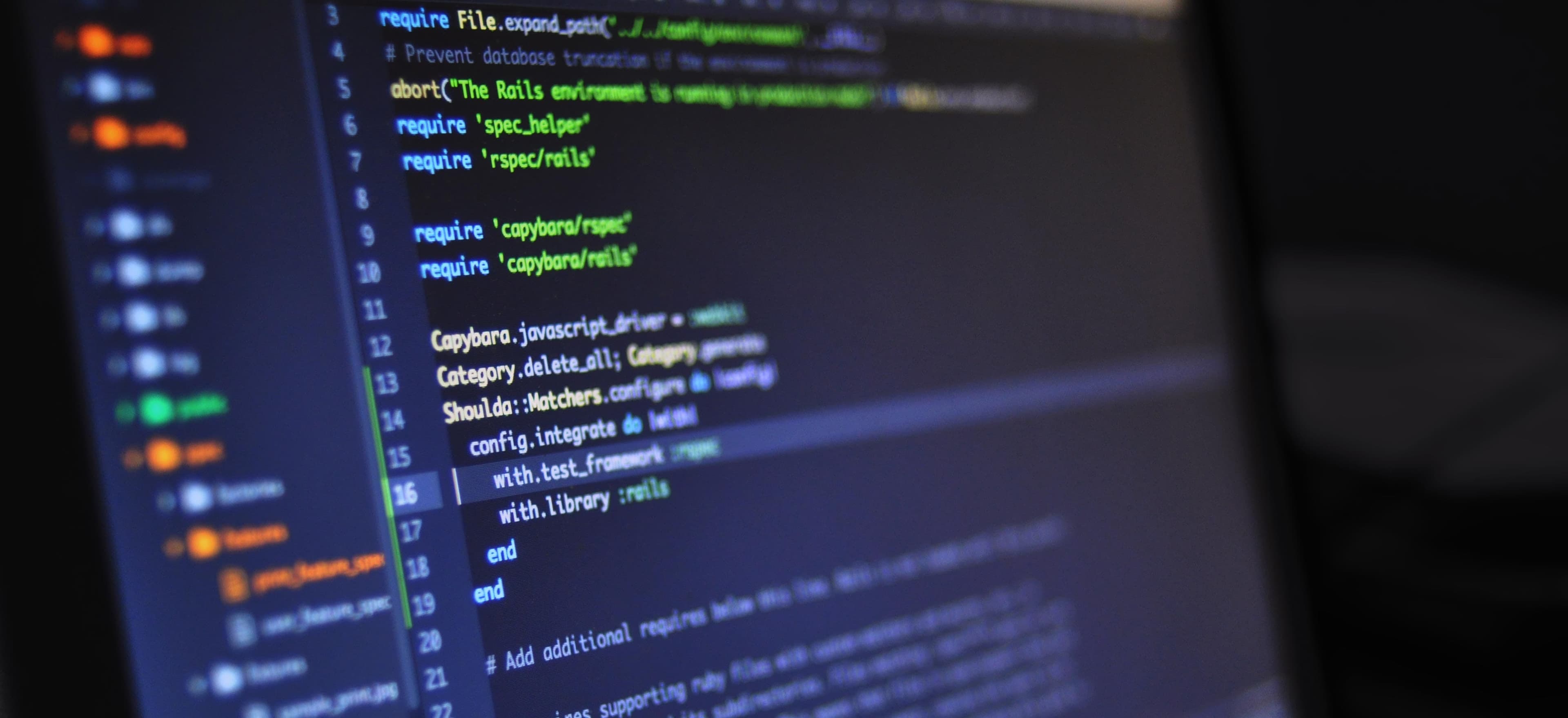
- Published on
Avoiding Spring's Trap: Perfecting Transactional Tests
When writing tests for code that interacts with a database, ensuring that the transactional behavior is correct is crucial. In a Spring application, using the @Transactional
annotation can simplify this task, but it can also create a common pitfall in testing. In this article, we'll explore how to perfect transactional tests in a Spring application to avoid potential traps and ensure reliable test behavior.
Understanding the Problem
In a typical Spring application, the @Transactional
annotation is often used to manage database transactions. When a method is annotated with @Transactional
, it runs within a transaction and the changes made to the database are rolled back after the method completes, thus ensuring that the database remains in a consistent state.
However, when writing tests for methods annotated with @Transactional
, it's important to consider that the same transactional behavior applies. This means that any data manipulation within the method will also be rolled back at the end of the test, which can lead to unexpected results and erroneous test cases.
The Trap of Transactional Tests
Consider the following example:
@Service
public class AccountService {
@Autowired
private AccountRepository accountRepository;
@Transactional
public void updateAccountBalance(Long accountId, BigDecimal newBalance) {
Account account = accountRepository.findById(accountId);
account.setBalance(newBalance);
}
}
In this example, the updateAccountBalance
method is annotated with @Transactional
to ensure that the database transaction is managed properly. However, when testing this method, the changes made to the Account
entity will be rolled back at the end of the test, making it impossible to verify the expected behavior.
Perfecting Transactional Tests
To perfect transactional tests in a Spring application, we can employ the following techniques:
1. Using @Transactional
at Test Class Level
By annotating the test class with @Transactional
, the entire test class will be executed within a transaction, and all changes made to the database during the test will be rolled back at the end.
@RunWith(SpringRunner.class)
@SpringBootTest
@Transactional
public class AccountServiceTests {
@Autowired
private AccountService accountService;
@Test
public void testUpdateAccountBalance() {
// Test logic here
}
}
By using @Transactional
at the test class level, we ensure that the test methods benefit from the same transactional behavior as the code being tested.
2. Utilizing @Rollback
Annotation
The @Rollback
annotation can be used at the method level to override the class-level default rollback behavior, allowing specific test methods to commit changes to the database.
@Test
@Rollback(false)
public void testUpdateAccountBalance() {
// Test logic here
}
By setting @Rollback(false)
on specific test methods, we can prevent the automatic rollback of changes made during the test, thus enabling us to verify the expected database state.
3. Utilizing TransactionTemplate
for Advanced Control
For more fine-grained control over transactions in test methods, using TransactionTemplate
can be highly beneficial. This allows for programmatically demarcating transaction boundaries and specifying commit or rollback behavior.
@Autowired
private TransactionTemplate transactionTemplate;
@Test
public void testUpdateAccountBalance() {
transactionTemplate.execute(status -> {
// Test logic here
return null; // Return value is not relevant
});
}
By utilizing TransactionTemplate
, we have granular control over the transaction behavior within the test, enabling us to manage the transaction as needed for the specific test scenario.
The Bottom Line
In a Spring application, perfecting transactional tests is essential to ensure reliable and accurate testing of database interactions. By understanding the potential traps of transactional tests and employing techniques such as using @Transactional
at the test class level, utilizing @Rollback
annotation, and leveraging TransactionTemplate
for advanced control, we can overcome these challenges and write robust and effective tests. This ensures that our tests accurately reflect the behavior of our database interactions and contribute to the overall quality and stability of our application.
To dive deeper into the concept of transactional testing and gain a more comprehensive understanding of Spring transactions, check out Spring official documentation and the book"Spring in Action".