Java Weak References: Avoiding Memory Leaks
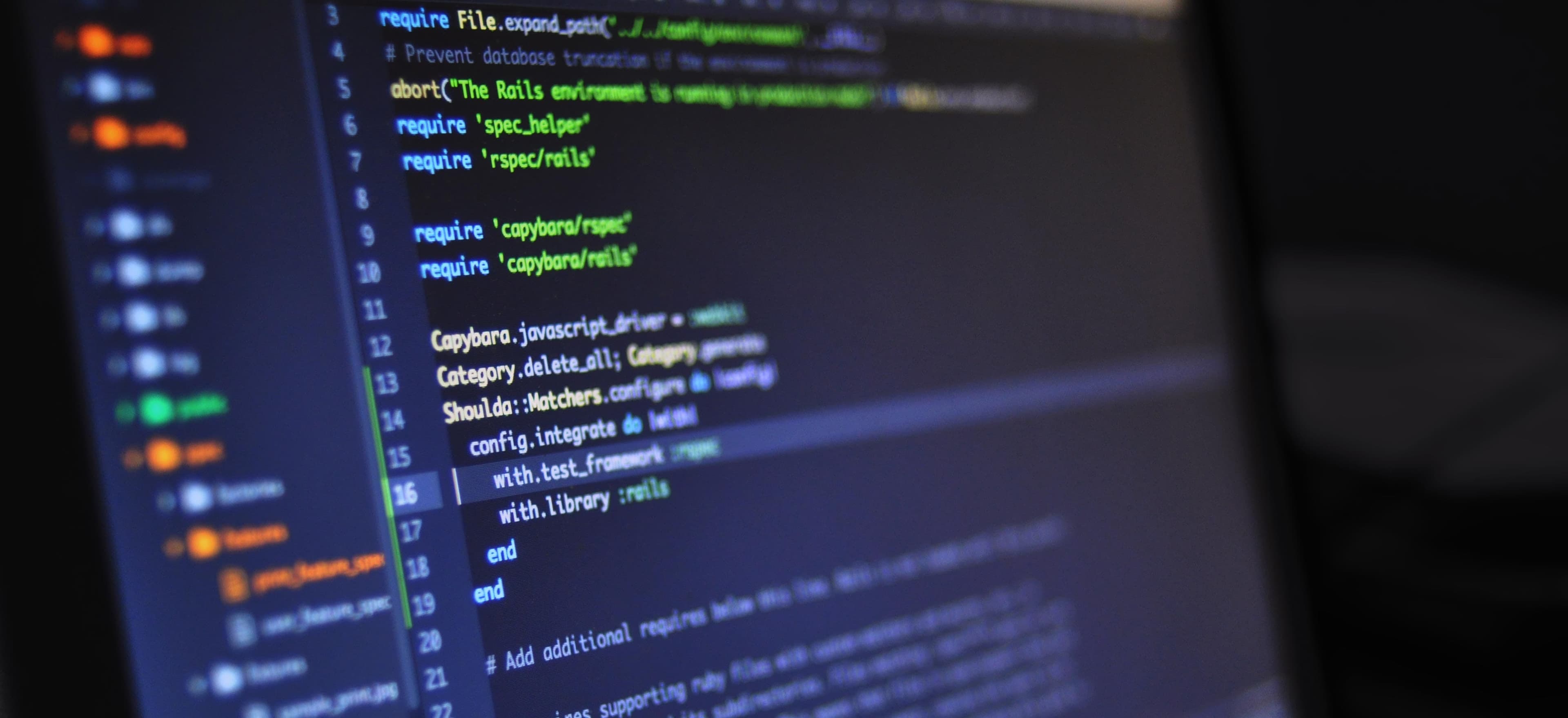
- Published on
Understanding Weak References in Java
In Java, memory management is a crucial aspect of application development. Without proper memory management, an application might suffer from memory leaks leading to degraded performance and even unexpected crashes. One of the key concepts in Java memory management is the usage of references, which can be strong, soft, weak, or phantom.
In this blog post, we will specifically delve into the world of weak references in Java, their significance, and how they can be leveraged to prevent memory leaks in your applications.
What are Weak References?
In Java, a weak reference is a type of reference that does not prevent the referenced object from being garbage collected. This means that if the only remaining references to an object are weak references, the garbage collector is free to reclaim the memory used by the object.
Weak references are represented by the java.lang.ref.WeakReference
class. They can be particularly useful when working with cache-like structures or event listeners, where you want an object to be garbage collected as soon as there are no strong references to it.
Why Use Weak References?
Imagine a scenario where you have a cache that holds a large number of objects, and you want these objects to be eligible for garbage collection when they are no longer in use. Using strong references for this cache could lead to memory leaks, as the objects would never be garbage collected as long as the cache holds strong references to them.
This is where weak references come into play. By using weak references for the objects in the cache, you allow the garbage collector to remove those objects when they are no longer strongly referenced, thus preventing memory leaks and ensuring efficient memory usage.
Implementing Weak References in Java
Let's take a look at a simple example to understand how weak references can be implemented in Java. Suppose we have a class MyObject
that we want to store in a cache using weak references.
import java.lang.ref.WeakReference;
import java.util.HashMap;
import java.util.Map;
public class ObjectCache {
private Map<String, WeakReference<MyObject>> cache = new HashMap<>();
public void addToCache(String key, MyObject obj) {
cache.put(key, new WeakReference<>(obj));
}
public MyObject getFromCache(String key) {
WeakReference<MyObject> ref = cache.get(key);
return ref != null ? ref.get() : null;
}
}
In this example, we have a simple ObjectCache
class that uses a HashMap
to store MyObject
instances using weak references. When adding an object to the cache, we create a new WeakReference
and store it in the map. When retrieving an object from the cache, we check if the weak reference is still valid and return the object if it is.
Avoiding Memory Leaks with Weak References
Memory leaks can occur when objects are no longer needed but are still being held in memory due to lingering references. By using weak references, we can ensure that objects are garbage collected as soon as they are no longer needed, thus avoiding memory leaks and optimizing memory usage.
Consider a scenario where we have a listener registration mechanism, and we want to ensure that listeners are automatically removed from memory when they are no longer in use. Using weak references for the listener registrations can prevent memory leaks in such scenarios, as the listeners can be garbage collected once they are no longer in use.
Key Takeaways
In conclusion, understanding and utilizing weak references in Java can play a crucial role in preventing memory leaks and optimizing memory usage in your applications. By allowing objects to be garbage collected when there are no strong references to them, weak references provide a powerful mechanism for efficient memory management.
In scenarios where objects need to be held in memory but should not prevent the garbage collector from reclaiming their memory, weak references offer an elegant solution. Leveraging weak references can lead to more robust and scalable applications, ensuring that memory is utilized optimally.
In your own Java projects, consider the use of weak references when dealing with cache-like structures, event listeners, or any scenario where objects should be eligible for garbage collection when no longer strongly referenced.
By incorporating weak references into your Java toolkit, you can enhance the performance and reliability of your applications, while also gaining a deeper understanding of memory management in the Java programming language.
And with that, it's time to put your newfound knowledge of weak references into practice in your own Java applications!
For further reading on this topic, be sure to check out Java's official documentation on WeakReference and this insightful article on memory management in Java.