Revolutionizing IoT: Integrating AWS Button with Couchbase
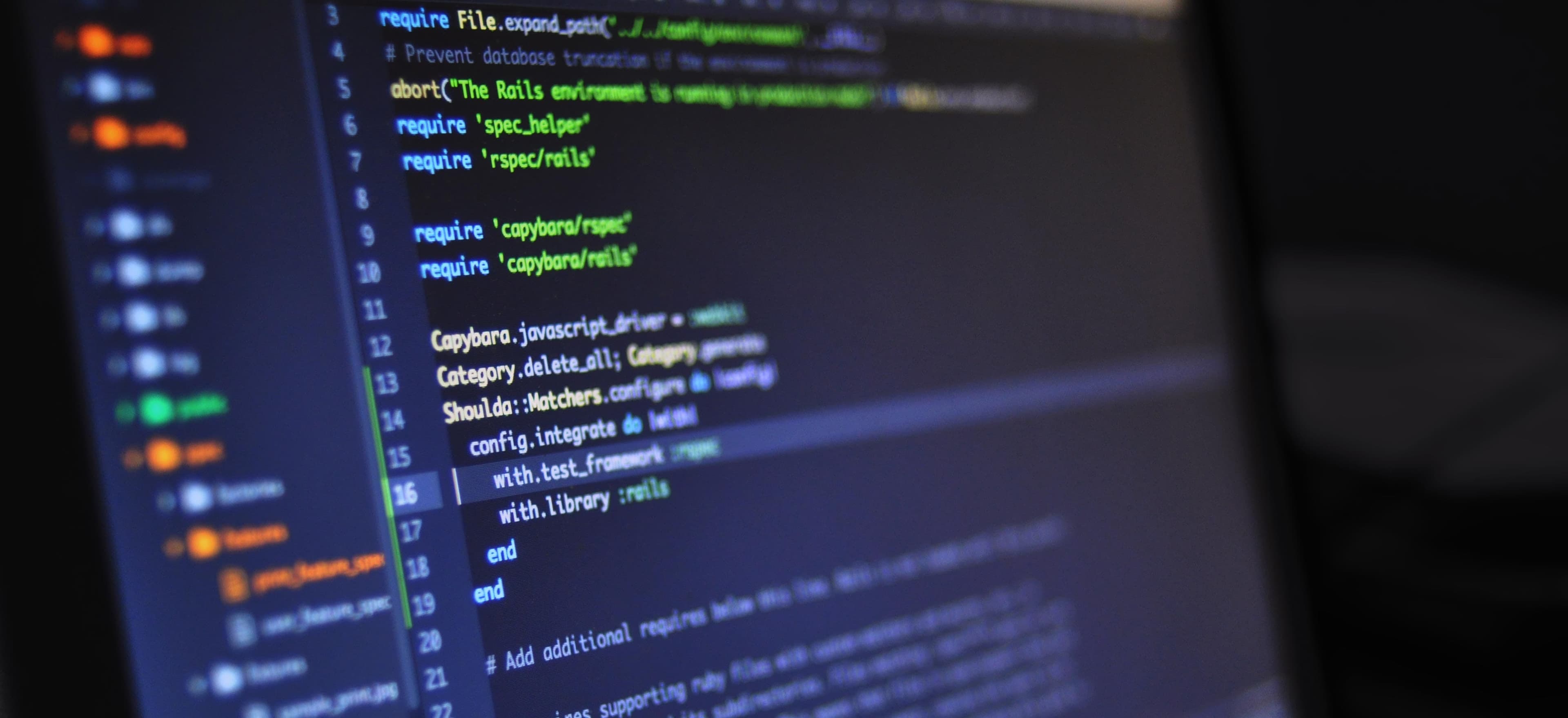
- Published on
Revolutionizing IoT: Integrating AWS Button with Couchbase
In today's fast-paced world, the Internet of Things (IoT) has revolutionized the way we interact with technology. The ability to control devices and gather data remotely has opened up new possibilities across various industries. As a Java developer, integrating IoT devices with powerful data storage solutions is essential for building robust and scalable applications. In this blog post, we will explore the integration of the AWS IoT Button with Couchbase, a leading NoSQL database, using the Java programming language.
Understanding the AWS IoT Button
The AWS IoT Button is a simple, easy-to-use device that allows users to trigger AWS Lambda functions, send alerts, or interact with other AWS services with a single click. It can be programmed to perform a wide range of tasks, making it a versatile tool for IoT applications. As a Java developer, integrating the AWS IoT Button with Couchbase can provide valuable insights and trigger actions based on real-world events captured by the button.
Getting Started with Couchbase
Couchbase is a powerful NoSQL database that provides a flexible data model and scalable architecture, making it ideal for handling IoT data. As a Java developer, leveraging Couchbase's capabilities to store and retrieve IoT data is crucial for building reliable and efficient IoT applications. Before diving into the integration, it's essential to set up a Couchbase cluster and create a bucket to store the IoT data.
Cluster cluster = Cluster.connect("your-couchbase-cluster");
Bucket bucket = cluster.bucket("your-bucket-name");
Collection collection = bucket.defaultCollection();
In this code snippet, we establish a connection to the Couchbase cluster, retrieve a specific bucket, and obtain the default collection for performing data operations. This initialization step is vital for any interaction with the Couchbase database.
Integrating the AWS IoT Button with Couchbase in Java
To integrate the AWS IoT Button with Couchbase in Java, we can use the AWS SDK for Java to handle button clicks and the Couchbase Java SDK to store and retrieve data from the database. Let's walk through a simple example of how to capture a button click event and store the data in Couchbase.
Handling Button Click Events
public class IoTButtonHandler implements RequestHandler<IotButtonEvent, String> {
@Override
public String handleRequest(IotButtonEvent event, Context context) {
String deviceId = event.getSerialNumber();
String clickType = event.getClickType();
// Store the button click event in Couchbase
JsonObject eventData = JsonObject.create().put("device_id", deviceId).put("click_type", clickType);
collection.insert("button_click_" + UUID.randomUUID().toString(), eventData);
return "Button click event stored successfully";
}
}
In this example, we define an AWS Lambda function using the RequestHandler interface from the AWS SDK for Java. The handleRequest method captures the IoT button click event, retrieves the device ID and click type, creates a JSON object to represent the event data, and stores it in Couchbase using the Couchbase Java SDK. This simple yet powerful integration enables the seamless capture and storage of IoT button click events.
Retrieving IoT Data from Couchbase
Once the IoT button click events are stored in Couchbase, we can retrieve and analyze the data to gain valuable insights. As a Java developer, utilizing the flexibility of Couchbase's N1QL query language enables us to perform complex queries and aggregations to extract meaningful information from the IoT data.
String n1qlQuery = "SELECT device_id, click_type, COUNT(*) AS count FROM `" + bucket.name() + "` WHERE type = 'button_click' GROUP BY device_id, click_type";
N1qlQueryResult result = cluster.query(n1qlQuery);
for (N1qlQueryRow row : result) {
JsonObject data = row.value();
String deviceId = data.getString("device_id");
String clickType = data.getString("click_type");
long count = data.getLong("count");
// Perform further analysis or trigger actions based on the IoT data
// ...
}
In this code snippet, we construct an N1QL query to aggregate and group the IoT button click events based on device ID and click type. The query result is then processed to extract the relevant data for further analysis or action triggering. Leveraging the power of Couchbase's querying capabilities allows us to derive meaningful insights from the stored IoT data.
Bringing It All Together
Integrating the AWS IoT Button with Couchbase in Java demonstrates the seamless fusion of IoT device interaction with powerful data storage and retrieval capabilities. As a Java developer, mastering the integration of IoT devices with robust data solutions like Couchbase is essential for building cutting-edge IoT applications. By capturing, storing, and analyzing IoT data, developers can unlock the full potential of IoT devices and drive innovation across various domains.
In conclusion, the integration of the AWS IoT Button with Couchbase in Java represents a significant step towards revolutionizing IoT applications. With the ability to capture real-world events, store data in a reliable database, and query for meaningful insights, this integration paves the way for sophisticated IoT solutions. As technology continues to evolve, Java developers play a vital role in shaping the future of IoT by leveraging powerful tools and frameworks to create impactful applications.
Stay tuned for more insightful content on Java programming and IoT integration. Happy coding!
Make the most out of Java programming by integrating IoT devices with powerful data storage solutions like Couchbase. Learn how to leverage the AWS IoT Button and Couchbase to build robust and scalable IoT applications with this in-depth guide.