Seamless Docker Integration for Continuous Deployment
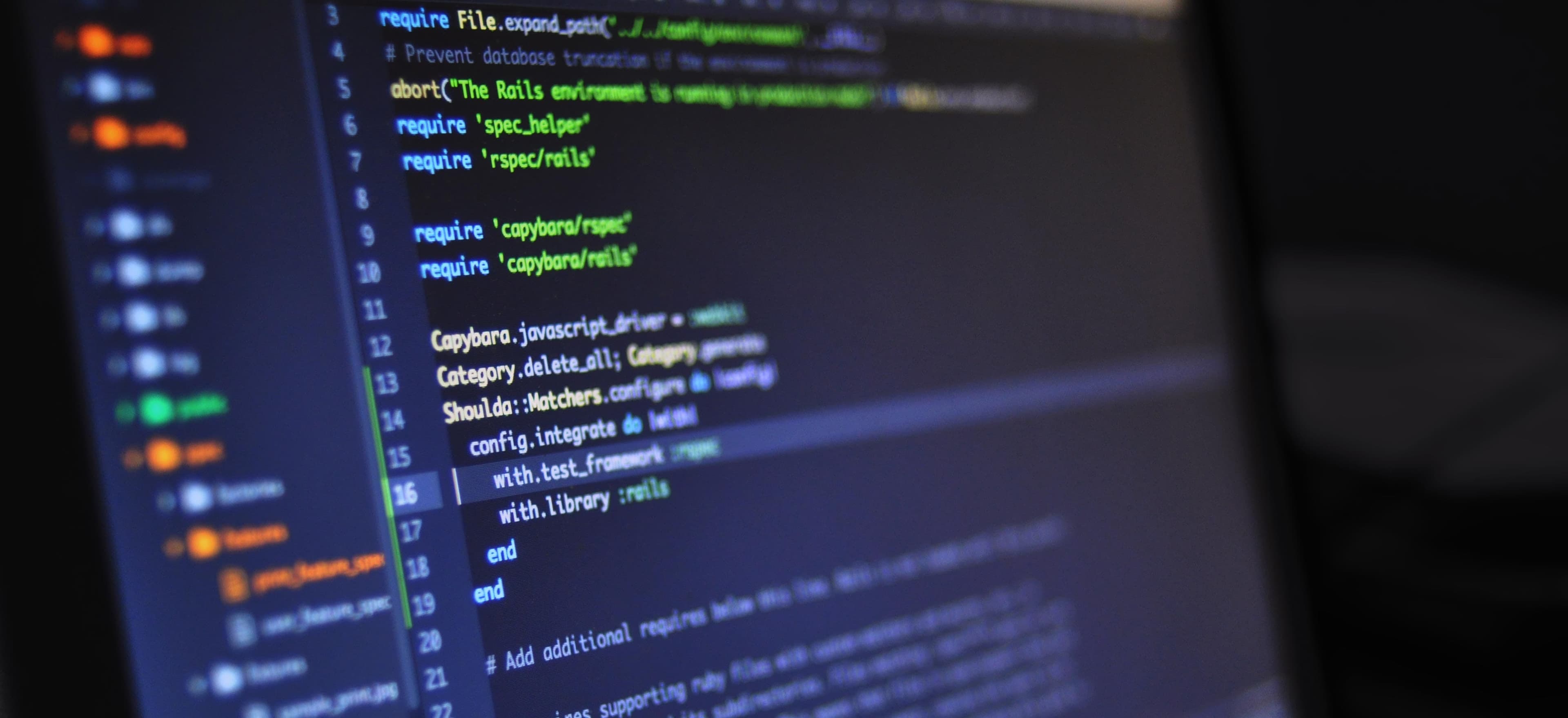
- Published on
Achieving Continuous Deployment with Docker and Java
Continuous deployment is a crucial aspect of modern software development. It enables teams to deliver code changes to production frequently and efficiently, resulting in faster time to market and better feedback loops. In this article, we will explore how Docker can be seamlessly integrated with Java to achieve continuous deployment.
Why Docker?
Docker has gained immense popularity in the software development world due to its ability to package an application and its dependencies into a standardized unit for software development. This unit, known as a container, ensures that the application works consistently across various environments. Docker containers are lightweight, portable, and can be run anywhere, making them an ideal choice for continuous deployment.
Dockerizing a Java Application
The first step towards achieving continuous deployment with Docker and Java is to Dockerize the Java application. Dockerizing a Java application involves creating a Docker image that contains the application code, dependencies, and configurations.
# Use the official image as base
FROM openjdk:11-jre-slim
# Set the working directory in the container
WORKDIR /app
# Copy the application JAR file into the container
COPY target/my-java-app.jar /app
# Specify the command to run the application
CMD ["java", "-jar", "my-java-app.jar"]
In the above Dockerfile, we start with the official OpenJDK 11 image as the base image. We then set the working directory in the container, copy the Java application JAR file into the container, and specify the command to run the application. This Dockerfile creates a Docker image that can run the Java application.
Continuous Integration and Continuous Deployment (CI/CD)
Continuous integration (CI) and continuous deployment (CD) play a crucial role in automating the software release process. CI/CD pipelines help in building, testing, and deploying the application automatically.
Setting up CI/CD Pipelines with Jenkins
Jenkins is a popular open-source automation server that enables CI/CD. Let's take a look at how we can set up a basic CI/CD pipeline for our Dockerized Java application using Jenkins.
-
Setup Jenkins: Install Jenkins on a server or use the Jenkins Docker image to run Jenkins as a container.
-
Create a New Pipeline: In Jenkins, create a new pipeline and link it to your code repository (e.g., GitHub).
-
Configure Build Steps: Define build steps in the Jenkins pipeline to build the Java application, Dockerize it, and push the Docker image to a container registry such as Docker Hub.
-
Trigger Deployment: Once the Docker image is pushed to the registry, trigger the deployment of the new image to the production environment.
By setting up a CI/CD pipeline with Jenkins, every code change to the repository will automatically trigger a series of steps, including building the Java application, creating a Docker image, and deploying it, ultimately leading to continuous deployment.
Orchestration with Kubernetes
Kubernetes is a powerful container orchestration platform that automates the deployment, scaling, and management of containerized applications. When it comes to continuous deployment with Docker and Java, Kubernetes plays a vital role in ensuring that the Dockerized Java application runs reliably and at scale.
By defining Kubernetes deployment manifests, we can specify how many instances of the application should be running, what resources they require, and how they should be exposed to the internet. Kubernetes takes care of managing and scaling the application based on the defined manifests, thereby enabling seamless continuous deployment.
Monitoring and Rollback
Continuous deployment also demands robust monitoring and rollback strategies to ensure the stability and reliability of the application. When it comes to monitoring a Dockerized Java application, tools like Prometheus and Grafana can be integrated with Kubernetes to collect and visualize metrics, providing insights into the application's performance and health.
Additionally, Kubernetes allows for easy rollbacks by managing multiple revisions of deployments. In case a new version of the application introduces issues, Kubernetes enables rolling back to a previous version with minimal downtime, ensuring smooth and reliable continuous deployment.
Closing the Chapter
In conclusion, Docker and Java make a powerful combination for achieving continuous deployment. By Dockerizing the Java application, setting up CI/CD pipelines with Jenkins, orchestrating with Kubernetes, and implementing robust monitoring and rollback strategies, teams can ensure seamless and efficient continuous deployment of their Java applications.
Continuous deployment with Docker and Java is not just a modern software development approach but also a critical aspect of staying agile and competitive in the ever-evolving tech industry.
So, leverage the power of Docker and Java to embrace continuous deployment and stay ahead in the game! Happy coding!
Checkout our other articles