NoSQL Confusion: Picking the Perfect Database for You
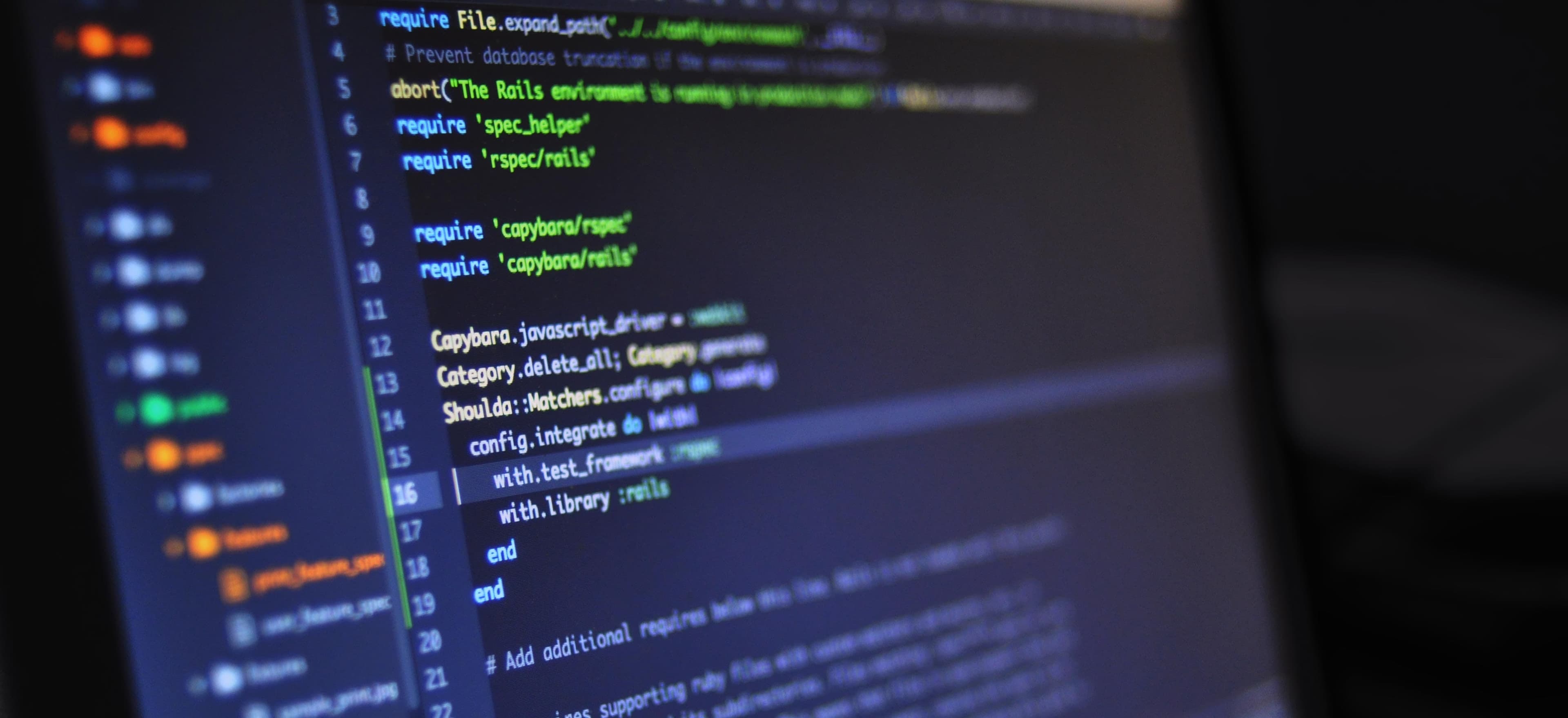
- Published on
Understanding NoSQL Databases in Java
In the world of Java development, the choice of a suitable database is crucial for the success of any project. With the advent of NoSQL databases, developers now have a wide array of options to choose from. NoSQL databases offer flexibility, scalability, and performance advantages over traditional relational databases. However, the abundance of NoSQL database options can be overwhelming, leading to confusion when trying to select the perfect fit for a specific project. In this blog post, we will explore the landscape of NoSQL databases and discuss the factors to consider when choosing the right NoSQL database for your Java project.
What are NoSQL Databases?
NoSQL databases are non-relational databases designed to handle large volumes of structured, semi-structured, or unstructured data. Unlike traditional SQL databases, NoSQL databases eschew the rigid structure of tables and rows in favor of more flexible data models, such as key-value pairs, document stores, column-family stores, and graph databases.
Types of NoSQL Databases
Key-Value Stores
- Example: Redis
- Use Case: High-speed caching and session storage
- Why: Simplicity and high performance for read and write operations.
Document Stores
- Example: MongoDB
- Use Case: Content management, real-time analytics
- Why: Schema-less design allowing for flexible and dynamic data structures.
Column-Family Stores
- Example: Apache Cassandra
- Use Case: Time series data, messaging systems
- Why: Horizontal scalability and high availability.
Graph Databases
- Example: Neo4j
- Use Case: Social networks, fraud detection
- Why: Ability to represent and navigate relationships between data points.
Factors to Consider When Choosing a NoSQL Database
Data Model
- Consideration: What type of data does your application handle?
- Why: Choosing a database with a data model that closely matches your application's data structure can simplify development and improve performance.
Scalability
- Consideration: Do you anticipate your data volume to grow significantly in the future?
- Why: NoSQL databases offer horizontal scalability, allowing you to add more machines to handle increased data loads.
Consistency
- Consideration: Is strong consistency a requirement for your application?
- Why: NoSQL databases often offer varying levels of consistency, from strong to eventual consistency, depending on the trade-offs between availability and data integrity.
Query Flexibility
- Consideration: How do you need to query your data?
- Why: Different NoSQL databases offer different query languages and capabilities, so choosing one that aligns with your application's query needs is crucial.
Example: Using MongoDB in a Java Application
Setting Up MongoDB in Java
To use MongoDB in a Java application, you can add the following Maven dependency to your project's pom.xml
file:
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongo-java-driver</artifactId>
<version>3.12.7</version>
</dependency>
Connecting to MongoDB
Here's an example of connecting to a MongoDB database and inserting a document using the official MongoDB Java driver:
MongoClient mongoClient = new MongoClient("localhost", 27017);
MongoDatabase database = mongoClient.getDatabase("mydb");
MongoCollection<Document> collection = database.getCollection("myCollection");
Document document = new Document("name", "John Doe")
.append("age", 30)
.append("email", "john.doe@email.com");
collection.insertOne(document);
Why MongoDB for This Use Case?
- Document Model: The flexibility of MongoDB's document model aligns well with the dynamic nature of the data being handled.
- Query Flexibility: MongoDB's rich query language allows for complex and expressive querying of data.
- Scalability: MongoDB's horizontal scalability makes it suitable for handling potential data growth.
My Closing Thoughts on the Matter
In conclusion, the selection of a NoSQL database for your Java project should be based on a thorough understanding of your application's data characteristics, scalability requirements, consistency needs, and query patterns. By taking these factors into account and evaluating the strengths of the different types of NoSQL databases, you can make an informed decision that will set your project up for success.
Remember, the "perfect" NoSQL database is the one that best aligns with your specific project requirements.
For more in-depth information on NoSQL databases and their integration with Java, check out the official MongoDB Java Driver documentation and Redis Java client documentation.
Make the right choice, and happy coding!