Overcoming Common Hurdles with EasyCriteria in JPA
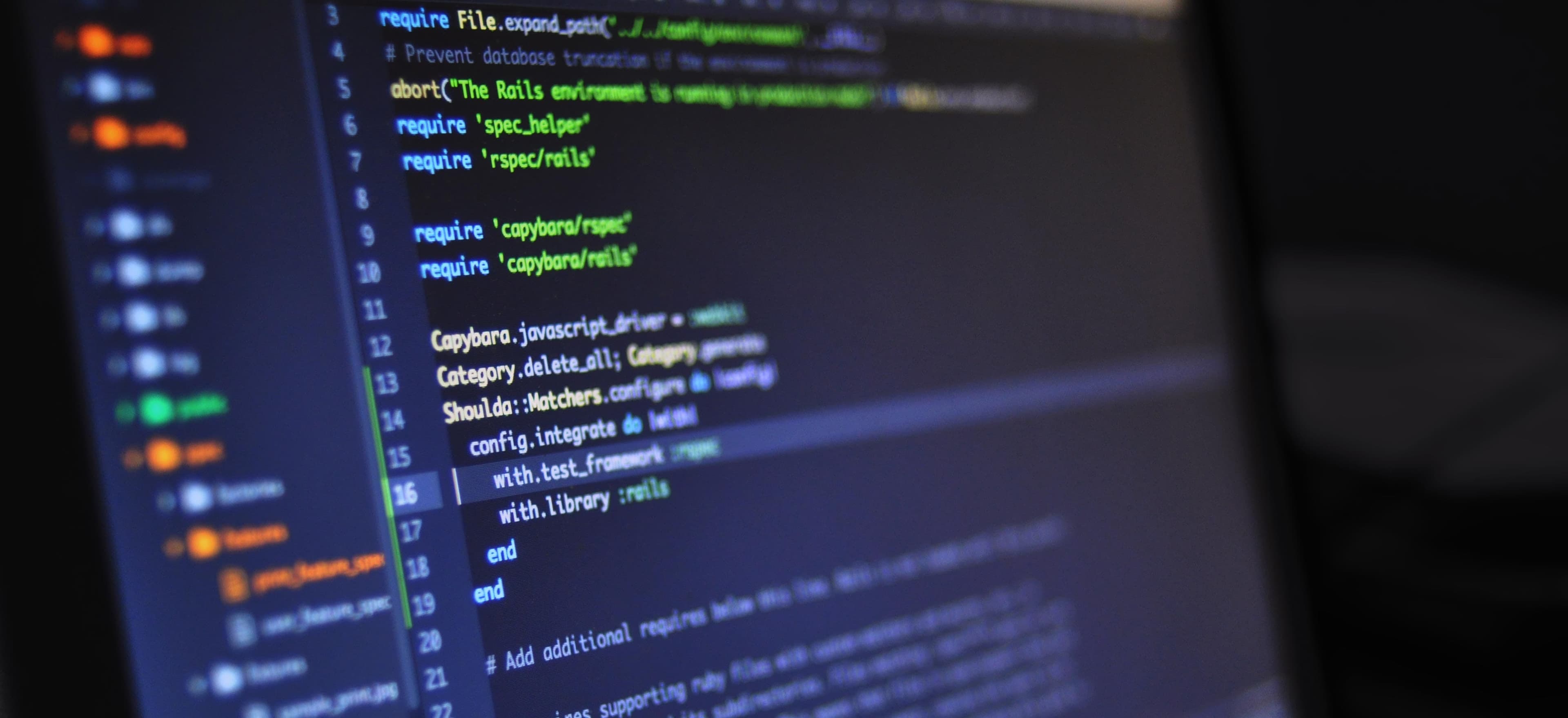
- Published on
Overcoming Common Hurdles with EasyCriteria in JPA
Java Persistence API (JPA) is an essential part of Java EE and Java SE platforms, providing a platform-independent object-relational mapping (ORM) technology for Java developers to manage relational data in Java applications. While JPA offers a powerful set of features, developers often face common hurdles when writing complex queries.
One of the common challenges is creating dynamic queries with optional parameters. This is where EasyCriteria comes to the rescue. EasyCriteria is a lightweight and fluent API that simplifies the process of building dynamic queries in JPA.
In this article, we will explore how EasyCriteria addresses common JPA query challenges and provides a streamlined solution for building dynamic queries.
Understanding the Common Hurdles
Before delving into the solution EasyCriteria offers, it's important to understand the common hurdles developers face when working with JPA.
1. Dynamic Queries
Building dynamic queries in JPA can be cumbersome, especially when dealing with optional search criteria. Writing conditional JPQL (Java Persistence Query Language) or Criteria API queries to accommodate optional parameters often leads to verbose and error-prone code.
2. Maintenance
Maintaining complex JPQL or Criteria API queries can be challenging, as changes to the data model or query requirements may necessitate extensive modifications to the existing queries.
3. Readability
Verbose and cluttered query construction code can reduce the readability of the application, making it harder for developers to grasp the query logic at a glance.
Introducing EasyCriteria
EasyCriteria simplifies the process of building dynamic queries in JPA, addressing the aforementioned challenges with its fluent and expressive API.
Streamlined Query Construction
With EasyCriteria, constructing dynamic queries becomes intuitive and straightforward. Optional search criteria can be seamlessly added to the query without cluttering the code with conditional logic.
Maintenance-Friendly
EasyCriteria promotes maintainability by encapsulating query construction in a concise and readable manner. This abstraction allows for easier modifications when adapting to changes in the data model or query requirements.
Improved Readability
By leveraging EasyCriteria's fluent API, the query construction code becomes more expressive and readable, promoting better comprehension of the query logic.
Using EasyCriteria in Practice
Let's dive into some practical examples to illustrate how EasyCriteria effectively addresses the common hurdles encountered when working with dynamic queries in JPA.
Example 1: Basic Query Construction
Consider a scenario where we need to construct a dynamic query based on the presence of optional parameters such as name and age. Using EasyCriteria, the query construction becomes succinct and manageable.
EasyCriteria<Person> criteria = EasyCriteriaFactory.createQueryCriteria(entityManager, Person.class);
if (name != null) {
criteria.andStringLike("name", "%" + name + "%");
}
if (age != null) {
criteria.andEquals("age", age);
}
List<Person> results = criteria.getResultList();
In this example, EasyCriteria allows us to add search criteria conditionally, simplifying the query construction process while maintaining readability.
Example 2: Chained Criteria
EasyCriteria enables the chaining of criteria, allowing for the construction of more complex queries while keeping the code concise and comprehensible.
EasyCriteria<Person> criteria = EasyCriteriaFactory.createQueryCriteria(entityManager, Person.class);
criteria.andStringLike("name", "%" + name + "%")
.andEquals("age", age)
.setMaxResults(10);
List<Person> results = criteria.getResultList();
By chaining criteria together, EasyCriteria promotes a clean and logical representation of complex query conditions.
Example 3: Sorting
Sorting results based on specific attributes is simplified with EasyCriteria's fluent API, enhancing query expressiveness and clarity.
EasyCriteria<Person> criteria = EasyCriteriaFactory.createQueryCriteria(entityManager, Person.class);
criteria.orderByAsc("name").orderByDesc("age");
List<Person> results = criteria.getResultList();
EasyCriteria's support for sorting enhances the readability and maintainability of the query construction code.
In Conclusion, Here is What Matters
In conclusion, EasyCriteria proves to be a valuable tool for overcoming common hurdles when constructing dynamic queries in JPA. Its fluent and expressive API streamlines query construction, promotes maintainability, and improves query readability. By simplifying the process of building dynamic queries, EasyCriteria enhances developer productivity and contributes to the overall maintainability of JPA-based applications.
Incorporating EasyCriteria into JPA projects can result in more concise, readable, and maintainable code, ultimately leading to a more efficient and enjoyable development experience. With its ability to effortlessly handle dynamic queries, EasyCriteria stands as a beneficial addition to any JPA developer's toolkit.
Elevate your JPA query-building experience with EasyCriteria, and say goodbye to the common hurdles that often accompany dynamic query construction!
Checkout our other articles