Overcoming Dependency Injection Challenges with Scala Macros
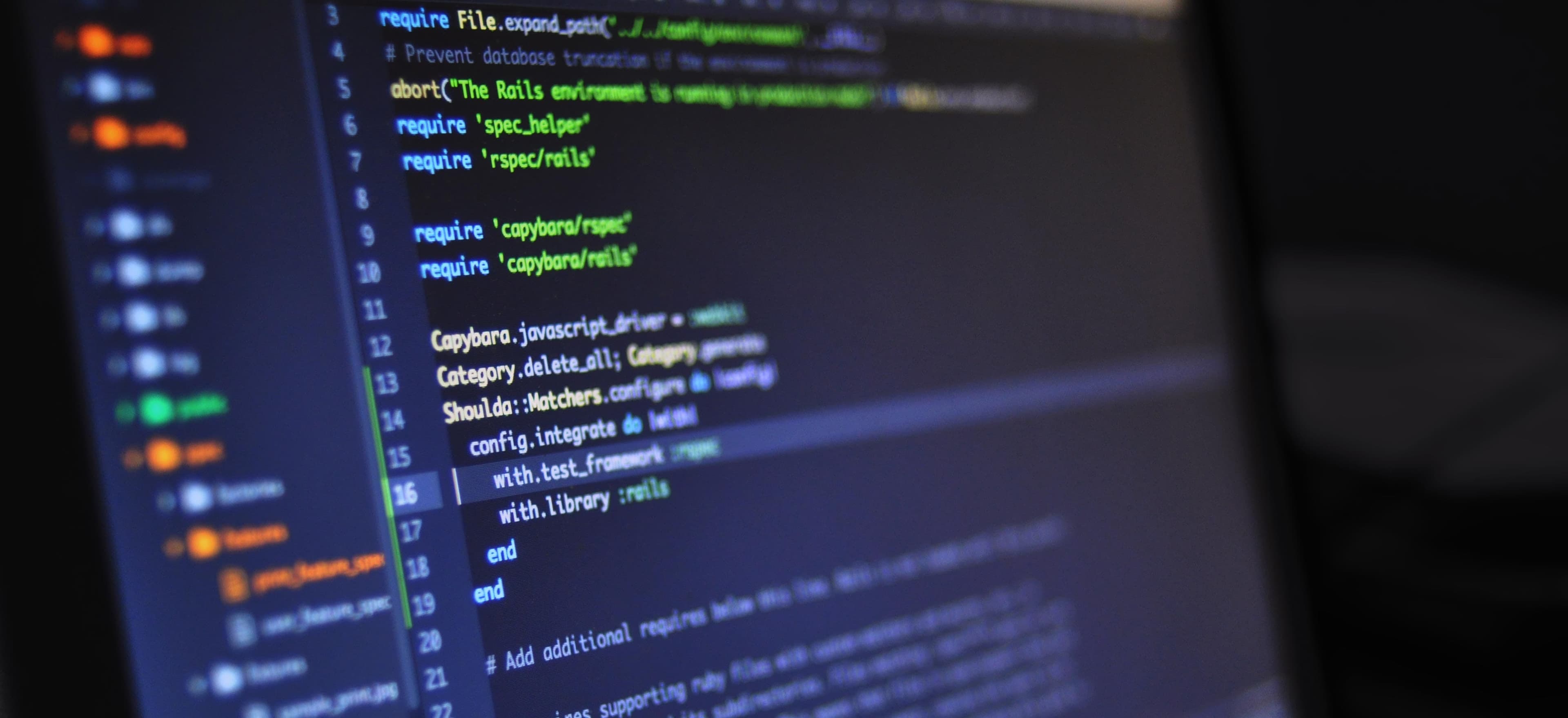
- Published on
Overcoming Dependency Injection Challenges with Scala Macros
Dependency injection is a popular design pattern in Java for implementing the inversion of control principle. It allows the creation and interconnection of objects with their dependencies without involving the class itself. While there are numerous frameworks available to implement dependency injection in Java such as Spring and Guice, they often come with runtime or reflection overhead. This is where Scala macros can offer an interesting solution. In this blog post, we will explore how Scala macros can be used to overcome dependency injection challenges in Java.
Understanding Dependency Injection
Dependency injection is a design pattern that allows the removal of hard-coded dependencies from the code, thus making it easier to manage, test, and scale. In simpler terms, rather than a class creating its dependencies, the dependencies are injected into the class from the outside. This allows for more flexibility, modularity, and testability.
In the context of Java, dependency injection is commonly achieved using frameworks like Spring or Guice. These frameworks use annotations or configuration files to manage the dependencies and inject them into the classes at runtime. While this approach is popular and widely adopted, it comes with its own set of challenges, such as:
-
Reflection Overhead: Dependency injection frameworks often rely on runtime reflection to resolve and inject dependencies, which can impact performance.
-
Complex Configuration: Configuring dependencies using annotations or XML files can become complex and hard to manage in large-scale applications.
-
Runtime Errors: Since dependency injection happens at runtime, errors related to missing or misconfigured dependencies may only surface during application execution.
Introducing Scala Macros
Scala macros provide a powerful metaprogramming mechanism that allows the generation of code at compile-time. With Scala macros, we can analyze and manipulate the abstract syntax trees (AST) of Scala code during compilation, enabling advanced compile-time code generation and transformation.
Using Scala Macros for Dependency Injection
One of the challenges of traditional dependency injection frameworks in Java is the overhead they introduce at runtime. Scala macros, being a compile-time feature, can help mitigate this issue by generating the necessary code during compilation. Let's look at a simplified example to illustrate how Scala macros can assist in dependency injection.
```scala
import scala.language.experimental.macros
import scala.reflect.macros.blackbox
object Injector {
def inject[T]: T = macro injectImpl[T]
def injectImpl[T: c.WeakTypeTag](c: blackbox.Context): c.Expr[T] = {
import c.universe._
val tpe = weakTypeOf[T]
// Perform custom logic to generate and inject dependencies at compile-time
// Example: Construct and inject dependencies based on annotations or configuration
// Return the generated code as an expression
c.Expr(q"new $tpe(/* Injected dependencies */)")
}
}
In this example, the `inject` method in the `Injector` object serves as the entry point for invoking the Scala macro. The `injectImpl` method then utilizes the Scala macro API to inspect the type `T` and generate the necessary code to inject dependencies during compilation. This code generation happens at compile-time, thereby eliminating the runtime overhead associated with traditional dependency injection frameworks.
By leveraging the power of Scala macros, we can achieve efficient and performant dependency injection in Java without sacrificing the benefits of inversion of control.
## Benefits of Using Scala Macros for Dependency Injection
### Performance Optimization
By generating code at compile-time, Scala macros eliminate the reflection overhead and runtime resolution typically associated with traditional dependency injection frameworks. This results in improved performance and reduced startup times for applications.
### Compile-Time Safety
Since dependency injection logic is evaluated during compilation, any errors or misconfigurations related to dependencies are caught early in the development cycle. This leads to more robust and predictable code, reducing the likelihood of runtime failures due to dependency issues.
### Seamless Integration with Java
Scala macros can be seamlessly integrated with existing Java codebases, allowing Java developers to harness the power of metaprogramming for dependency injection while leveraging their existing knowledge and tools.
## A Final Look
In this blog post, we have explored how Scala macros can be used to overcome dependency injection challenges in Java. By leveraging the compile-time code generation capabilities of Scala macros, we can achieve efficient, performant, and statically-typed dependency injection without the overhead of traditional runtime frameworks.
While Scala macros offer a compelling alternative for dependency injection, it is essential to weigh the benefits against the complexity introduced by metaprogramming. As with any advanced programming technique, thorough understanding and careful consideration of trade-offs are crucial when incorporating Scala macros into a Java codebase.
In conclusion, Scala macros provide an intriguing avenue for addressing dependency injection challenges in Java and offer a glimpse into the potential of compile-time metaprogramming for enhancing software design and performance.
For further exploration into Scala macros and their application in Java, consider delving into the official [Scala Macros Guide](https://docs.scala-lang.org/overviews/macros/overview.html) and [Scala Reflect API](https://docs.scala-lang.org/overviews/reflection/overview.html).
Incorporate Scala macros into your Java projects to unlock the power of compile-time metaprogramming and elevate your dependency injection strategies to a new level of efficiency and performance.