Solving Maven Assembly Struggles for Vulnerability Checks
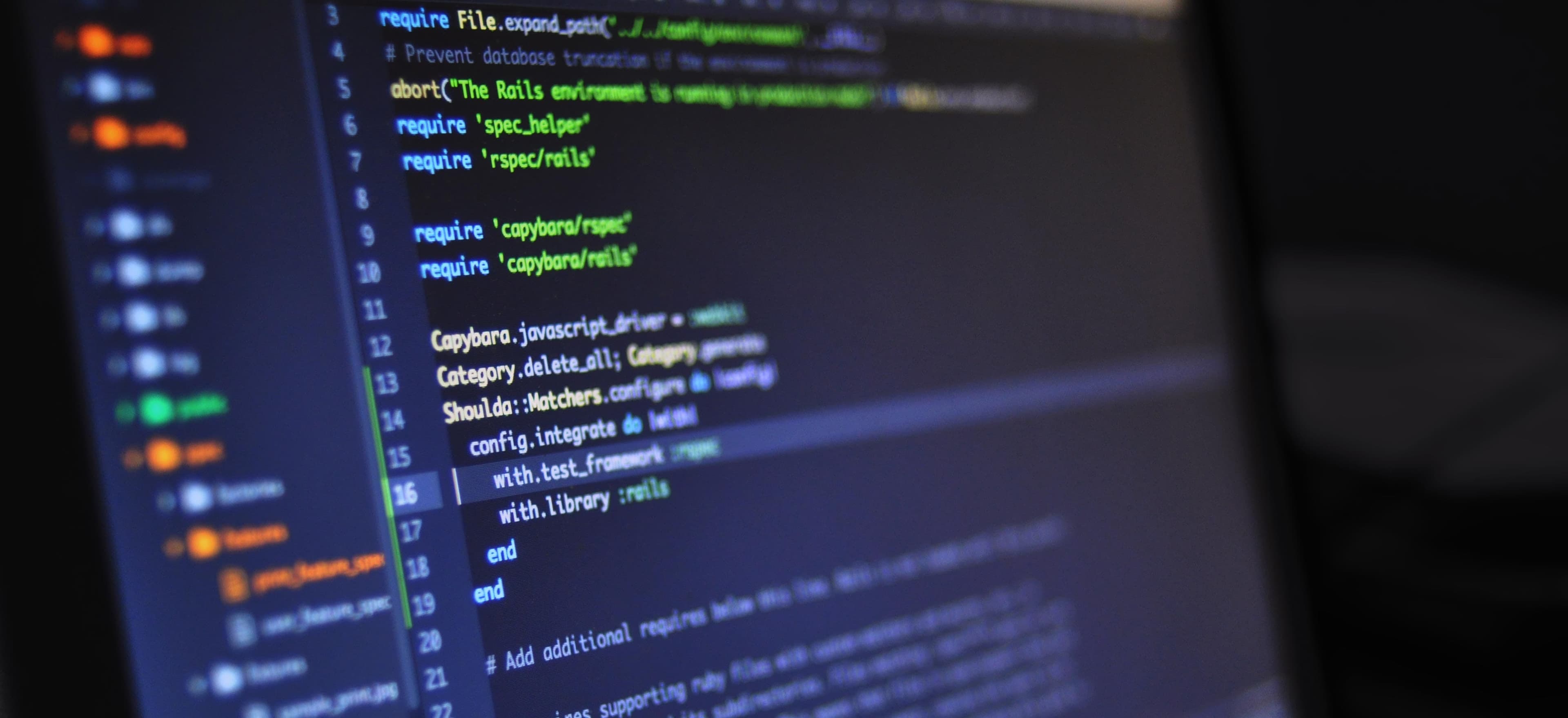
- Published on
Solving Maven Assembly Struggles for Vulnerability Checks
As software development becomes increasingly complex, securing your codebase has never been more critical. One common issue that developers face is ensuring that third-party dependencies in their Java applications are free from vulnerabilities. Fortunately, various tools and techniques can help in this effort, and in this article, we will explore methods to incorporate vulnerability checks into your Maven assembly process. By the end of this article, you will have a clear understanding of how to improve the security of your Java applications through vulnerability checks in Maven.
Understanding the Challenge
Before delving into the solution, it is crucial to comprehend the challenge at hand. When assembling a Java application using Maven, it is essential to ensure that all included dependencies are free from known vulnerabilities. This is crucial for securing your application against potential exploits and attacks. Typically, vulnerabilities are discovered in third-party libraries, and it becomes imperative to identify and address these vulnerabilities within your own applications.
Leveraging OWASP Dependency-Check
One effective approach to addressing this challenge is by integrating the OWASP Dependency-Check tool into your Maven build process. OWASP Dependency-Check is a widely used open-source utility that identifies project dependencies and checks if there are any known, publicly disclosed, vulnerabilities. By leveraging this tool, you can automatically scan your project's dependencies and identify any vulnerable components.
Integrating OWASP Dependency-Check into Maven
To integrate OWASP Dependency-Check into your Maven build process, you can utilize the dependency-check-maven
plugin. Below is an example of how to configure the plugin within your Maven pom.xml
file:
<build>
<plugins>
<plugin>
<groupId>org.owasp</groupId>
<artifactId>dependency-check-maven</artifactId>
<version>6.3.1</version>
<executions>
<execution>
<phase>verify</phase>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
In this configuration, we specify the dependency-check-maven
plugin and set the phase to verify
, which ensures that the vulnerability check occurs as part of the build process. The check
goal triggers the vulnerability analysis.
Viewing the Results
Upon running the Maven build with the integrated OWASP Dependency-Check, the results of the vulnerability analysis will be displayed in the build output. This immediate feedback loop allows you to quickly identify any vulnerable dependencies within your project.
Further Automation with Continuous Integration
While running vulnerability checks locally is a great start, integrating this process into your continuous integration (CI) pipeline can provide ongoing security validation for your codebase. Most modern CI platforms, such as Jenkins, GitLab CI, and CircleCI, allow for easy integration of OWASP Dependency-Check through plugins or custom build steps.
By incorporating vulnerability checks into your CI pipeline, you ensure that any new code additions do not introduce additional security risks. This proactive approach significantly reduces the likelihood of shipping vulnerable code to production.
Handling Vulnerability Findings
Upon identifying vulnerable dependencies, it is essential to have a process in place to handle these findings effectively. Depending on the severity of the vulnerability, you may choose to upgrade to a patched version of the library, seek alternative libraries, or apply mitigations to reduce the risk.
It is crucial to stay informed about security advisories for your project's dependencies. Websites such as the National Vulnerability Database (NVD) and the Common Vulnerabilities and Exposures (CVE) database are valuable resources for understanding the impact of vulnerabilities and the availability of fixes.
Beyond OWASP Dependency-Check
While OWASP Dependency-Check is powerful, it is not the only tool available for vulnerability checks. Snyk is another popular option for identifying and fixing vulnerabilities in open-source code and Docker images. Additionally, the Nexus IQ Server provides a robust platform for automated security, license, and quality policy governance.
Depending on your project's specific requirements and constraints, it is essential to evaluate and leverage the tools that best fit your organization's needs.
The Last Word
In conclusion, securing your Java applications through vulnerability checks in Maven is a critical practice in modern software development. By integrating OWASP Dependency-Check into your Maven build process and extending this practice to your CI pipeline, you enhance the security posture of your applications.
Remember, vulnerability management is an ongoing process, and staying proactive in identifying and addressing vulnerabilities is crucial for maintaining the integrity and security of your codebase.
Incorporate vulnerability checks into your Maven build process today, and take a significant step towards enhancing the security of your Java applications.
For more information on OWASP Dependency-Check, visit OWASP official website.
To explore additional tools for vulnerability management, consider visiting Snyk's website for comprehensive insights.
Now, go forth and secure your Java applications effectively!